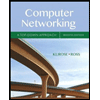
2. ParseInts
File ParseInts.java contains a program that does the following:
Prompts for and reads in a line of input
Uses a second Scanner to take the input line one token at a time and parses an integer from each token as it is extracted.
Sums the integers.
Prints the sum.
Save ParseInts to your directory and compile and run it. If you give it the input
10 20 30 40
it should print
The sum of the integers on the line is 100.
Try some other inputs as well. Now try a line that contains both integers and other values, e.g.,
We have 2 dogs and 1 cat.
You should get a NumberFormatException when it tries to call Integer.parseInt on “We”, which is not an integer. One way around this is to put the loop that reads inside a try and catch the NumberFormatException but not do anything with it. This way if it’s not an integer it doesn’t cause an error; it goes to the exception handler, which does nothing. Do this as follows:
Modify the program to add a try statement that encompasses the entire while loop. The try and opening { should go before the while, and the catch after the loop body. Catch a NumberFormatException and have an empty body for the catch.
Compile and run the program and enter a line with mixed integers and other values. You should find that it stops summing at the first non-integer, so the line above will produce a sum of 0, and the line “1 fish 2 fish” will produce a sum of 1. This is because the entire loop is inside the try, so when an exception is thrown the loop is terminated. To make it continue, move the try and catch inside the loop. Now when an exception is thrown, the next statement is the next iteration of the loop, so the entire line is processed. The dogs-and-cats input should now give a sum of 3, as should the fish input.
// ****************************************************************
// ParseInts.java
//
// Reads a line of text and prints the integers in the line.
//
// **************************************************************** import java.util.Scanner;
public class ParseInts
{
public static void main(String[] args)
{
int val, sum=0;
Scanner scan = new Scanner(System.in); String line;
System.out.println("Enter a line of text");
Scanner scanLine = new Scanner(scan.nextLine());
while (scanLine.hasNext())
{
val = Integer.parseInt(scanLine.next());
sum += val;
}
System.out.println("The sum of the integers on this line is " + sum);
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Write a program that uses an initially empty file to store a sorted list of integers entered by the user. Theintegers are stored in binary form. Each time the program is run, it opens the file and outputs the list of storedintegers onto the screen. The program then asks the user to enter a new integer X. The program then looks at theinteger at the end of the file. If that integer is less than or equal to X, the program stores X at the end of the fileand closes the file. Otherwise, the program starts at the end of the file and works toward the beginning, movingeach value in the file that is greater than X up by one until it reaches the position in the file where X should bestored. The program then writes X at that position and closes the file.arrow_forwardWrite a python script that opens a text file names.txt in "reading" mode, uses a for loop to scan the file, read each line and print it. screenshot of text file includedarrow_forwardcan you do it pythonarrow_forward
- Java Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardIn java Browse Project Gutenberg (https://www.gutenberg.org/) for plain text versions of one or more works of literature of your choosing. Write a program that repeatedly searches a file for a target word or phrase.• Allow the user to supply the name of the file to search by means of a command-line argument; if the user does not supply a command line argument, ask for a file name interactively.• You may use either an array or an ArrayList to store each line in the file; but if you use an array, you’ll have to determine the array size (number of lines) before allocating the array. There are several ways to do this.• Report how many lines were read from the file.• Search for either individual words or phrases (Hint: use the .contains method, it’ll work for either a word or a phrase)• Show both the line number and the line itself for each line that contains the search target entered by the user (you may count the first line in the file as line 0).• If a search word is part of a larger…arrow_forwardWrite a java program that will generate a permuterm index from the terms in the files in an input directory. The program should prompt the user for a single directory and find all of the terms (after processing) in each file in it. The program should then find the permutations of each term and store them (keeping track of the original word each permutation comes from). After all of the text files in the directory have been read, the output of the program should display the permutations in sorted order along with the original term. Use the dollar sign as the end of term symbol.arrow_forward
- I am trying to figure out why the sum at the end of my Java code is not working correctly. It keeps showing as zero and I do not know what the issue is. Here is the program requirements Write a program in a single file that: Main: Creates 10 random doubles, all between 1 and 11, Calls a method that writes 10 random doubles to a text file, one number per line. Calls a method that reads the text file and displays all the doubles and their sum accurate to two decimal places. SAMPLE OUTPUT 10.62691196041722.7377903389094555.4279257388651281.37420580654725091.18587002624988364.1803912764852284.9109699989306755.7108582343439587.7908570073730523.1806714736219543 The total is 47.13 Here is my code: import java.util.*;import java.io.*; public class AssignmentTwo { public static void main(String[] args) {//main method double randNum [] = new double [10]; for(int i = 0; i< randNum.length; i++) { randNum[i] = (double)(Math.random()* (10) + 1);…arrow_forwardWrite a program that reads data from a file containing integers that ends with -999. Output the numbers that are divisible by 7 Output the numbers that are divisible by 11 Output the numbers that are not divisible by 7 or 11arrow_forwardWrite a complete java program that: asks the user for a file name tests whether the file exists and tells the user if it does exist asks the user whether it should be deleted. Either delete or do not delete the file based on the user input. If they don't delete, ask them if they would like to write to the file If they want to write, ask what they want to write to the file and write it. Make sure to provide plenty of console output dialog specifying what status/actions are taken at each step.arrow_forward
- In Java I need to design an ESP Game: Design a program to test your extrasensory perception. The program will randomly pick a card (you could consider only the card suit, for example [Hearts, Diamonds, Clubs, Spades]. ) Prompt the user to enter a suit, then display whether the user guessed correctly. ESP Game Outcomes: Modify your ESP game to write the outcome of a game to a file. Allow the user to play the ESP game multiple times, writing each outcome to a file. Then, determine the number of times the user guessed correctly reading from the file. Please include flowcharts and/or class diagramsarrow_forwardWrite a Java code that creates two files with the names file4 and file4a. create two int arrays with 10 elements each with the names arrfile4 and arrfile4a; these arrays are to have different values write the contents of arrfile4 to file4write the contents of arrfile4a to file4aarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
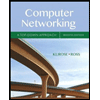
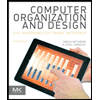
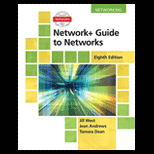
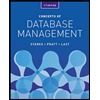
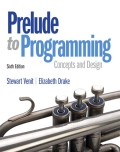
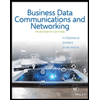