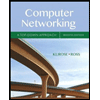
// FixedDebugFive04
// Program prompts user for any number of values
// (up to 20)
// and averages them
// C# Program
using System;
public class DebugFive04
{
public static void Main()
{
int[] numbers = new int[20];
const int QUIT = 999;
int x;
int num;
double average;
double total = 0;
Console.Write("Please enter a number or " +
QUIT + " to quit...");
num = Convert.ToInt32(Console.ReadLine());
x = 0;
while ((x < numbers.Length) && num != QUIT)
{
numbers[x] = num;
total += numbers[x];
Console.Write("Please enter a number or " +
QUIT + " to quit...");
num = Convert.ToInt32(Console.ReadLine());
x++;
}
average = total / x;
Console.WriteLine("The average is {0}", average);
Console.WriteLine("The numbers are:");
for (int y = 0; y < x; ++y)
Console.Write("{0,6}", numbers[y]);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- C programming #include <stdio.h>int main() {int i, j, n ;printf("height? ") ;scanf("%2d", &n) ;for (i = 1 ; i <= n ; i++) {// printf("%d: ", i) ;for (j = 1 ; j <= i ; j++) {// Pick *one* of the following// printf("%d", j % 10) ;printf("*") ;}printf("\n") ;}return 0 ;} How can I get output like this by editing the given programming -- height? 5************************* and then how can I get the following?- height? 7 1 123 12345 1234567 123456789 12345678901 1234567890123arrow_forwardFix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forwardC programmingarrow_forward
- #include using namespace std; int main() { } int kidsInClass1; int kidsInClass2; int numClasses; double kidsAvgMethodl; double kidsAvgMethod2; kidsInClass1= 3; kidsInClass2 = 2; numClasses = 2; Type the program's output kidsAvgMethodl = static_cast (kidsInClass1 + kidsInClass2) /static_cast (numClasses); kidsAvgMethod2 (kidsInClass1 + kidsInClass2) / numClasses; cout << kidsAvgMethod1 << endl; cout << kidsAvgMethod2 << endl; return 0; =arrow_forwardFor C programming languagearrow_forwardpublic class Side Plot { * public static String PLOT_CHAR = "*"; public static void main(String[] args) { plotxSquared (-6,6); * plotNegXSquaredPlus20 (-5,5); plotAbsXplus1 (-4,4); plotSinWave (-9,9); public static void plotXSquared (int minX, int maxx) { System.out.println("Sideways Plot"); System.out.println("y = x*x where + minX + "= minX; row--) { for (int col = 0; col row * row; col++) { System.out.print (PLOT_CHAR); if (row== 0){ } System.out.print(" "); } for(int i = 0; i <= maxx* maxX; i++) { System.out.print("-"); } System.out.println(); Sideways PHOT y = x*x where -6<=x<=6 Sideways Plot y = x*x where -6<=x<=6 How do I fix my code to get a plot like 3rd picture?arrow_forward
- Please explain this question void main() {int a =300; char *ptr = (char*) &a ; ptr ++; *ptr =2; printf("%d", a); }arrow_forwardThis assignment is not graded, I just need to understand how to do it. Please help, thank you! Language: C++ Given: Main.cpp #include #include "Shape.h" using namespace std; void main() { /////// Untouchable Block #1 ////////// Shape* shape; /////// End of Untouchable Block #1 ////////// /////// Untouchable Block #2 ////////// if (shape == nullptr) { cout << "What shape is this?! Good bye!"; return; } cout << "The perimeter of your " << shape->getShapeName() << ": " << shape->getPerimeter() << endl; cout << "The area of your " << shape->getShapeName() << ": " << shape->getArea() << endl; /////// End of Untouchable Block #2 //////////} Shape.cpp string Shape::getShapeName() { switch (mShapeType) { case ShapeType::CIRCLE: return "circle"; case ShapeType::SQUARE: return "square"; case ShapeType::RECTANGLE: return "rectangle"; case…arrow_forwardc++ code Screenshot and code is mustarrow_forward
- C++ - No library functions like atoi Write a machine language program to output your first name on the output device. Submit your "machine code" followed by a 'zz.' An example of the machine code to output "hello" is shown below. This is an example of what a machine language submission would look like: 50 00 48 50 00 65 50 00 6c 50 00 6c 50 00 6f 00 zzarrow_forwardc languagearrow_forwardint main(){ long long int total; long long int init; scanf("%lld %lld", &total, &init); getchar(); long long int max = init; long long int min = init; int i; for (i = 0; i < total; i++) { char op1 = '0'; char op2 = '0'; long long int num1 = 0; long long int num2 = 0; scanf("%c %lld %c %lld", &op1, &num1, &op2, &num2); getchar(); long long int maxr = max; long long int minr = min; if (op1 == '+') { long long int sum = max + num1; maxr = sum; minr = sum; long long int res = min + num1; if (res > maxr) { max = res; } if (res < minr) { minr = res; } } else { long long int sum = max * num1; maxr = sum; minr = sum; long long int res = min * num1;…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
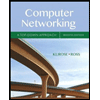
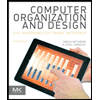
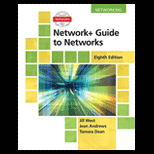
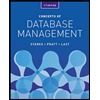
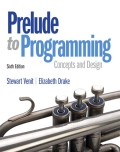
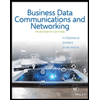