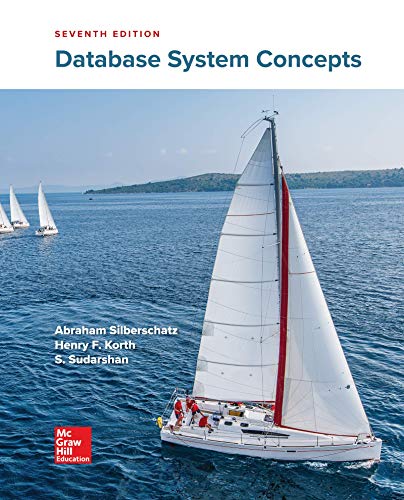
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Forms often allow a user to enter an integer. Write a program that takes in a string representing an integer as input, and outputs yes if every character is a digit 0-9.
Ex: If the input is:
1995
the output is:
yes
Ex: If the input is:
42,000
or
1995!
the output is:
no
Hint: Use a loop and the Character.isDigit() function.
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String userString;
// Add more variables as needed
userString = scnr.next();
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- IN PYTHON Write a script which uses the input function to read a string, an int, and a float, as input from keyboard prompts the user to enter his/her name as string, his/her age as integer value, and his/her income as a decimal. For example your output will display as: Mark is 30 years old and his income is 2000 Please show code.arrow_forwardIn java please help with the following: Given a string that contains space separated words, write an application that displays the words in ascending order (If two words are the same, display only one). You need to use the right data structure for storing the words. For example, for input:String input="apple banana apple orange blueberry"; The program will print out: apple banana blueberry orangearrow_forwardQuestio Write a program that receives a series of characters from the user, the program stops if the user enters the same character two consecutive times, the program should display the number of entered characters at the end of the program (the last duplicated character is counted only once): Sample Run: Enter your characters: a sbnmkrr we got 7 characters please wirte it in java using loops please answer it in java using netbeans.arrow_forward
- 24. Write a program which asks the user for an integer number and prints out whether the input number is a multiple of 7. A. Example 1 i. Input: • ii. Output: B. Example 2 i. Input: Please enter number: 9 ii. Output: Number is not a multiple of seven 25. Write a program which asks the user for two integer numbers (say N1 and N2) and prints out whether the input number NI is a multiple of N2. A. Example 1 i. Input: • B. Example 2 ii. Output: • i. Input: A. Example Please enter number: 49 Number is a multiple of seven i. Input: ii. Output: Number 40 is not a multiple of 3 26. Write a program which asks the user for an integer number and prints out whether the input number is even or odd. Please enter first number: 49 Please enter second number: 7 Number 49 is a multiple of 7 Please enter first number: 40 Please enter second number: 3 • Please enter number: 42 i. Input ii. Output: Number is even 27. Write a program that asks the user for an integer number and prints a pattern. A. Example…arrow_forwardCode in Pythonarrow_forwardIn Java create a class CharsToUnicodeWhile. Write code that reads from the user a string of characters and then prints them in Unicode. The user can quit the program by typing “Q' or “q”, otherwise the program stays in a loop and reads another string to convert it to Unicode. Use a while loop to test “Q' or “q” and a for loop to traverse the entered string, character by character. Hint: Initialize the while-loop condition BEFORE you enter the loop and update the while-loop condition AT THE END of the body of the loop.arrow_forward
- This program does not throw an error, but when an odd number is input for the variable number, it prompts the user for a letter, but doesn't allow the user to actually input a letter. How would you fix this error?arrow_forwardin java Strings itemToFind and inputItem are read from input. Integer wordCount is initialized with 1. Write a while loop that iterates until inputItem is equal to "Done". In each iteration of the loop: Increment wordCount if inputItem is equal to itemToFind. Read string inputItem from input. Click here for example Note: Assume that input has at least two strings. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 import java.util.Scanner; public class SimpleWhileLoop { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); StringitemToFind; StringinputItem; intwordCount; itemToFind=scnr.next(); inputItem=scnr.next(); wordCount=1; /* Your code goes here */ System.out.println(itemToFind+" occurs "+wordCount+" time(s)."); } }arrow_forwardC Language - Write a program that takes in three integers and outputs the largest value. If the input integers are the same, output the integers' value.arrow_forward
- code in c++arrow_forwardImplement a program that prompts the user to enter two positive numbers as formatted below Enter num1: Enter num2: Input validation and Integer Overflow MUST be completed input must be all digits input must be a positive number, but cannot exceed INTEGER OVERFLOW Check the std::string input number doesn't exceed ULONG_MAX Check the sum of the inputted numbers doesn't exceed ULONG_MAX If NOT valid, then re-prompt as formatted below INVALID RE-Enter num 3 incorrect inputs in a row and stop program. This is the 3 strikes and you're out rule. PROGRAM ABORT Outputs the sum of the two positive integers when input is valid as formatted below in the example test runs. Expected Program Output: Test Run #1 Enter num1: 12345 Enter num2: 9876 12345 + 9876 ------- 22221 Test Run #2 Enter num1: 378 Enter num2: 16429 378 + 16429 ------- 16807 Test Run #3 Enter num1: -378 INVALID RE-Enter num1: abc INVALID RE-Enter num1: 18446744073709551616 PROGRAM ABORT Test Run #4 Enter num1: -378…arrow_forward1. Write a pyrhon program that prints out a classic hangman stick figure. The program should ask the user to enter a number from 1-6 and the corresponding hangman should be printed. The value the user inputs corresponds to the number of incorrect guesses in a real hangman game and so the completeness of the hangman will correspond to the number of ‘incorrect guesses’ inputted by the user (e.g., if the user enters 1, then only the head of the hangman will be printed; full example below). Example:Enter a number from 1-6: 1O Enter a number from 1-6: 2O|Enter a number from 1-6: 3O\||Enter a number from 1-6: 4O\|/|Enter a number from 1-6: 5O\|/|/Enter a number from 1-6: 6O\|/|/ \ 2. Modify your program from problem 1 so that the user input is checked to be a validsingle digit (1-6) before printing the corresponding hangman. If the input is not valid, theninstead of a hangman the following message should be printed “Invalid input: you must enter asingle number from 1-6.” Example:Enter a…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
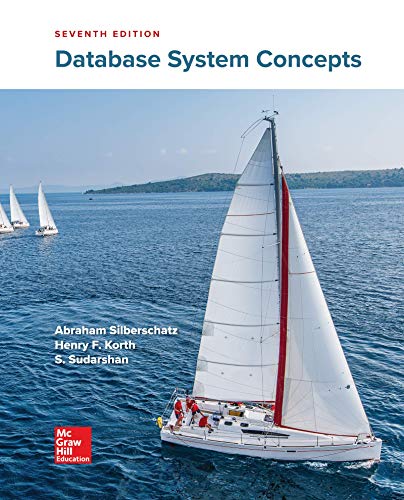
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
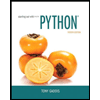
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
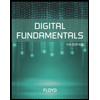
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
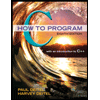
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
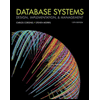
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
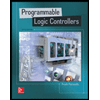
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education