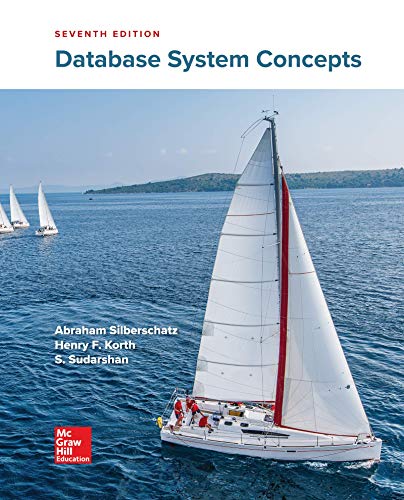
Given a sequence of 5 element keys < 23, 26, 16, 38, 27 > for searching task:
a) Given the hash function H(k) = (3.k) mod 11, insert the keys above according to its original sequence (from left to right) into a hash table of 11 slots. Indicate the cases of collision if any.
b) In case of any collisions found above in a) part, determine the new slot for each collided case using Linear Probing to solve the collision problem. Clearly show your answer for each identified case. No step of calculation required. (Answer with “NO collision found”, in case there is no collisions found above)
c) In case of any collisions found above in a) part, determine the new slot for each collided case using Double-Hashing (with functions below) to solve the collision problem. Show your steps and calculations with a table as in our course material. d1 = H(k) = (3.k) mod 11 di = (di−1 + ((5·k) mod 10) + 1) mod 11 , i ≥ 2 (Answer with “NO collision found”, in case there is no collisions found above)
d) Suppose the given sequence < 23, 26, 16, 38, 27 > is stored in linear structure of an array (stored from left, as the beginning of the array) for simple sequential searching, find the average search length (ASL) if they have probabilities < 0.1, 0.2, 0.2, 0.2, 0.3 > respectively in searching. Clearly show the steps of your calculations.

Step by stepSolved in 3 steps

- Answer the following ture or false question 1. The 9-entry hash table that results from using the hash function, h(i) = (2i+7) mod 9, to hash the keys 12, 5, 13, 4, 10, 19, 8, 3, and 28, assuming collisions are handled by linear probing as follows: 0 1 2 3 4 5 6 7 8 10 19 3 28 12 8 13 4 5 Group of answer choices True False 2. Here is how the built-in sum function can be combined with Python’s comprehension syntax to compute the sum of all numbers in an n x n data set, represented as a list of lists: sum(sum(subset) for subset in data) Group of answer choices True False 3. Here is the comparison for asymptotic growth rate: (logn) < (n) < (nlogn) < (n^2) < (n^3) < (2^n) Group of answer choices True False 4. Here is the Python’s list comprehension syntax to produce the list of this 26 characters ['a', 'b', 'c', ..., 'z']: [chr(k) for k in range(97, 123)] Group of answer choices True Falsearrow_forwardGiven the hash function: h(i) = i % 13Show the array after inserting the following keys: 18, 41, 22, 44, 59, 32, 31, 73, in this order. Use linear probing to resolve collisions.arrow_forward7. Let T be a hash table in which collisions are resolved by chaining. Given a key k, briefly explain how a search operation for k in T works.arrow_forward
- Please answer all parts of the question, thanksarrow_forwardGiven a sequence of 5 keys < 53, 66, 32, 41, 88 > for searching task: a) Given the hash function H(k) = (3.k) mod 7, insert the keys above according to its original order (from left to right) into a hash table of 7 slots. Indicate the cases of collision. Clearly show the steps and calculation with a table. b) In case of any collisions found above in a) part, determine the new slot for each collided case using Linear Probing to solve the collision problem. Clearly show your answer for each identified case. No step of calculation required. o (Answer with “NO collision found”, in case there is no collisions found above) c) In case of any collisions found above in a) part, determine the new slot ONLY for the first collided case using Double-Hashing (with functions below) to solve the collision problem. Clearly show the steps of your calculation. d1 = H(k) = (3.k) mod 7 di = (di−1 + ((5·k) mod 6) + 1) mod 7 , i ≥ 2 o (Answer with “NO collision found”, in case there is no collisions…arrow_forwardInsert the following sequence of keys in the hash table.Keys = {4, 2, 1, 3, 5, 6, 8}Use linear probing technique for collision resolution: h(k, i) = [h(k) + i] mod mHash function, h(k) = 3k + 1Table size, M = 10 Draw the hash table, compute the index where the hashvalue will be inserted for each key, and show how you would use the technique when a collisionoccurs.arrow_forward
- What is the worst-case performance of a lookup operation in a hashmap and why? Group of answer choices A- O(1), hashmap always has a constant time lookup, and that is why we like using this associative data structure. B- O(lg(n)) hashmap has a log(n) lookup because we are able to perform a binary search on the keys because our hashmap always maintains a sorted order of entries added. C- O(n) because we can have a bad hash function that puts all of our items in the same bucket, thus we would have to iterate through all n items.arrow_forwardGiven the input set A = {1, 2, 3, 4, 5} and output set B = {1, 2, 3, 4}, is it possible to find a hash function H from A to B that has no hash collision, i.e., LaTeX: \forall ∀ a1, a2 LaTeX: \in ∈A, H(a1) LaTeX: \ne ≠H(a2) If yes, please give the function. Otherwise, please explain. (Hint: pigeonhole principle)arrow_forwardSuppose we use a hash function h to hash n distinct keys into an array T oflength m. Assuming simple uniform hashing, what is the expected number ofcollisions? More precisely, what is the expected cardinality of ffk; lg W k ¤ l andh.k/ D h.l/g?arrow_forward
- With Hash tables we have chaining and open addressing. What are the algorithms for both?how you would insert or search a hash table under either approach? Please give me examples of how I would code these algorithmsarrow_forward1) Suppose hashing is being done by using open addressing with linear probing. The hash function tobe used for an element with key k is h(k) = k mod 11. Show the contents of the hash table afterinserting the following keys in order into the hash table:27 40 22 15 31 18 36 19 20arrow_forwardThe best technique of hashing when memory space is not a problem is O Linear probing O Quadratic probing O Double hashing O Separate chainingarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
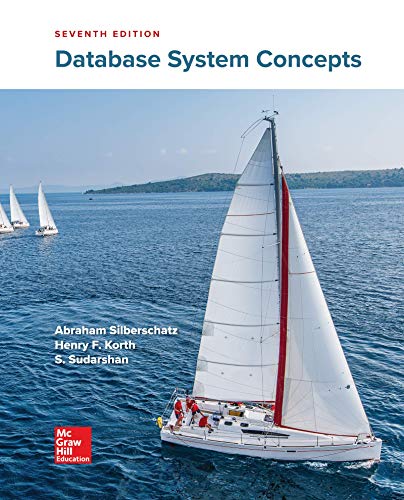
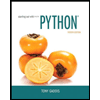
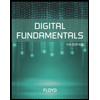
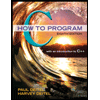
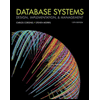
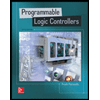