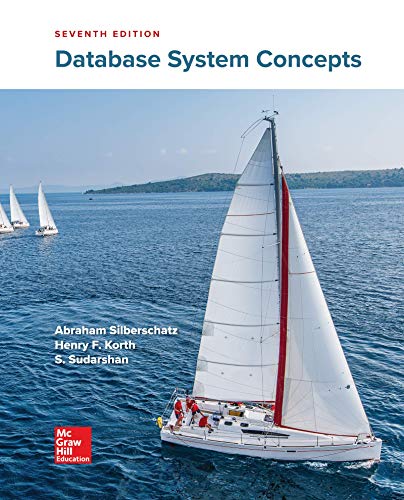
Concept explainers
Given a singly linked list, find the middle of the linked list. For example, if the given linked list is 1->2->3->4->5 then the output should be 3. If there are even nodes, then there would be two middle nodes, we need to print the second middle element. For example, if given linked list is 1 >2->3->4->5->6 then the output should be 4.
Make the following adjustments to the class HWLinkedList code below
- Add the method addAtHead to the class below. The method should simply add a new
element to the beginning of the linked list instead of the end.
- Add the method searchList to the class below. The method should return true if the user
specified object is found and false otherwise. The method must be recursive.
- Add the remove method
public class HWLinkedList<E>
{
private class Node<E>
{
private E data;
Node<E> next;
public Node(E data)
{
this.data = data;
this.next = null;
}
}
private Node<E> head;
public HWLinkedList()
{
this.head = null;
}
private String toString (Node<E> head)
{
if (head == null)
return””;
return head.data.toString() + “\n” + toString(head.next);
}
public String toString()
{
return toString(head);
}
private void add(Node<E> head, E data)
{
if (head.next == null)
head.next = new Node<E>(data);
else
add(head.next, data)
}
public void add(E data)
{
if (head == null)
head = new Node<E>(data);
else
add(head, data);
}
}
to generate a solution
a solution
- In c++ please Example of Output: Input a string: ........ letters r b с f 2. Demonstrate by writing a program to calculate the frequency of every letter in an input string s. S e a. Your program should be able to input a string. b. Your message should be constructed from at least 50 letters. c. Calculate the frequency of each element in the string and store the results into a linked list. d. Your program should be able to print each letter and its respective frequency. e. Identify the letter with the highest frequency in your message. f. Sort the linked list in ascending order using an algorithm of your choice. Write a short justification of your choice. frequency 1 4 6 12 20 24 30arrow_forwardA singly linked list contains n - 1 strings that are binary representations of numbers from the set {0, 1,.…, n – 1} where n is an exact power of 2. However, the string corresponding to one of the numbers is missing. For example, if n = 4, the list will contain any three strings from 00, 01,10 and 11. Note that the strings in the list may not appear in any specific order. Also note that the length of each string is lgn, hence the time to compare two strings in O(lgn). Write an algorithm that generates the missing string in O(n).arrow_forwardGiven a singly linked list L, where x and y are two data elements that occupy the nodes NODEX and NODEY with PREVIOUSX as the node, which is the previous node of NODEX, write a pseudo-code to swap the date x and y in list L by manipulating the links only (data swapping is not allowed). Assume that x and y are available in the list and are neither neighbors nor the end nodes of list L. For example, given the list L shown in Figure P6.10(a), with L, NODEX, NODEY and PREVIOUSX marked on it, the swapping should yield the list shown in Figure P6.10(b). NODEX and NODEY are neither immediate neighbors nor the end nodes of list L. Th PREVIOUS X NODE X ‘oddada g c PREVIOUS X (a) Before swapping g and x NODE Y с NODE Y X NODE X addgħa W (b) After swapping g and x Figure P6.10. Swapping of elements in a singly linked list by manipulating links iarrow_forward
- Execute a program that will split a circularly linked list P with n nodes intotwo circularly linked lists P1, P2 with the first n/2 and the last n – n/2 nodes ofthe list P in them.arrow_forwardGiven a linked list of N nodes ..arrow_forwardGiven a singly linked list of integers, reverse the nodes of the linked list 'k' at a time and return its modified list. 'k' is a positive integer and is less than or equal to the length of the linked list. If the number of nodes is not a multiple of 'k,' then left-out nodes, in the end, should be reversed as well.Example :Given this linked list: 1 -> 2 -> 3 -> 4 -> 5 For k = 2, you should return: 2 -> 1 -> 4 -> 3 -> 5 For k = 3, you should return: 3 -> 2 -> 1 -> 5 -> 4 Note :No need to print the list, it has already been taken care. Only return the new head to the list. Input format :The first line contains an Integer 't' which denotes the number of test cases or queries to be run. Then the test cases follow. The first line of each test case or query contains the elements of the singly linked list separated by a single space. The second line of input contains a single integer depicting the value of 'k'. Remember/Consider :While specifying the list…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
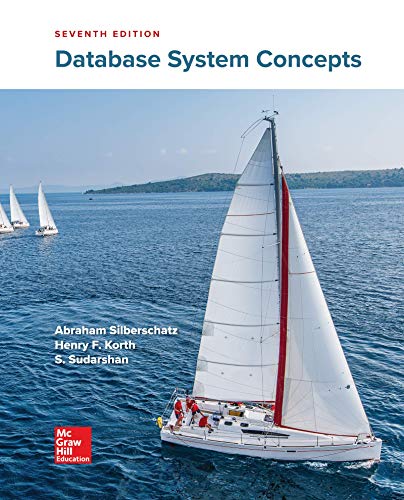
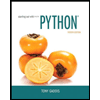
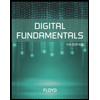
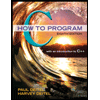
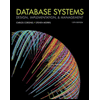
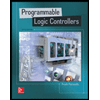