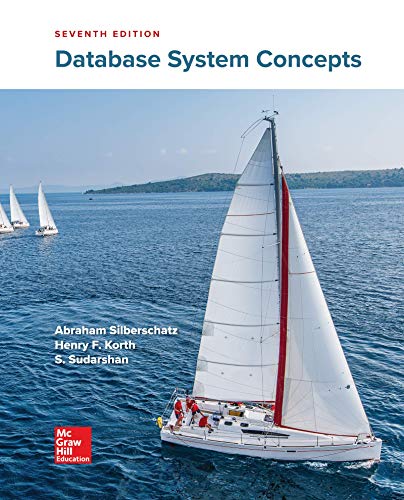
Given main(), define the Team class (in files Team.h and Team.cpp). For class member function GetWinPercentage(), the formula is:
teamWins / (teamWins + teamLosses)
Note: Use casting to prevent integer division.
Ex: If the input is:
Ravens
13
3
where Ravens is the team's name, 13 is number of team wins, and 3 is the number of team losses, the output is:
Congratulations, Team Ravens has a winning average!
If the input is Angels 80 82, the output is:
Team Angels has a losing average.
#ifndef TEAMH
#define TEAMH
#include <string>
class Team {
// TODO: Declare private data members - teamName, teamWins, teamLosses
// TODO: Declare mutator functions -
// SetTeamName(), SetTeamWins(), SetTeamLosses()
// TODO: Declare accessor functions -
// GetTeamName(), GetTeamWins(), GetTeamLosses()
// TODO: Declare GetWinPercentage()
};
#endif
#include "Team.h"
using namespace std;
// TODO: Implement mutator functions -
// SetTeamName(), SetTeamWins(), SetTeamLosses()
// TODO: Implement accessor functions -
// GetTeamName(), GetTeamWins(), GetTeamLosses()
// TODO: Implement GetWinPercentage()

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardc++ A FastCritter moves twice as fast as a regular critter. When asked to move by n steps, it actually moves by 2 * n steps. Implement a FastCritter class derived from Critter whose move function behaves as described. Complete the following file: fastcritter_Tester.cpp #include <iostream>using namespace std; #include "critter.h" /**A FastCritter moves twice as fast as a regular critter.*/class . . .{public:void move(int steps);}; . . . int main(){FastCritter speedy;speedy.move(10);cout << speedy.get_history() << endl;cout << "Expected: [move to 20]" << endl;speedy.move(-1);cout << speedy.get_history() << endl;cout << "Expected: [move to 20, move to 18]" << endl;return 0;} Use the following file: critter.h #ifndef CRITTER_H #define CRITTER_H #include <string> #include <vector> using namespace std; /** A simulated critter. */ class Critter { public: /** Constructs a critter at position 0 with blank history. */ Critter();…arrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forward
- Each function inside the class A(n)_ is a virtual function. Provide your thoughts by filling in the blanks.arrow_forwardIn C++arrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forward
- In C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forwardc++ A FastCritter moves twice as fast as a regular critter. When asked to move by n steps, it actually moves by 2 * n steps. Implement a FastCritter class derived from Critter whose move function behaves as described. Complete the following file: fastcritter_Tester.cpp #include <iostream>using namespace std; #include "critter.h" /**A FastCritter moves twice as fast as a regular critter.*/class FastCritter:public Critter{public:void move(int steps);}; . . . int main(){FastCritter speedy;speedy.move(10);cout << speedy.get_history() << endl;cout << "Expected: [move to 20]" << endl;speedy.move(-1);cout << speedy.get_history() << endl;cout << "Expected: [move to 20, move to 18]" << endl;return 0;} Use the following file: critter.h #ifndef CRITTER_H #define CRITTER_H #include <string> #include <vector> using namespace std; /** A simulated critter. */ class Critter { public: /** Constructs a critter at position 0 with blank…arrow_forwardI already have the code for the assignment below, but the code has an error in the driver class. Please help me fix it. The assignment: Make a recursive method for factoring an integer n. First, find a factor f, then recursively factor n / f. This assignment needs a resource class and a driver class. The resource class and the driver class need to be in two separate files. The resource class will contain all of the methods and the driver class only needs to call the methods. The driver class needs to have only 5 lines of code The code of the resource class: import java.util.ArrayList;import java.util.List; public class U10E03R{ // Recursive function to // print factors of a number public static void factors(int n, int i) { // Checking if the number is less than N if (i <= n) { if (n % i == 0) { System.out.print(i + " "); } // Calling the function recursively // for the next number factors(n, i +…arrow_forward
- Java - Car Value (Classes)arrow_forwardIn GO language. Create a struct that has student name, id, and GPA. Write functions to create a student, modify the student’s id, and modify the student's GPA, and print the student’s information. (This is like creating a class and methods). Now create an array of three students and test your functions. You may hardcode your values if using a web conpiler. (Please hardcode the values!)arrow_forwardarray of Payroll ObjectsDesign a PayRoll class that has data members for an employee’shourly pay rate and number of hours worked. Write a program withan array of seven PayRoll objects. The program should read thenumber of hours each employee worked and their hourly pay ratefrom a file and call class functions to store this information in theappropriate objects. It should then call a class function, once foreach object, to return the employee’s gross pay, so this informationcan be displayed.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
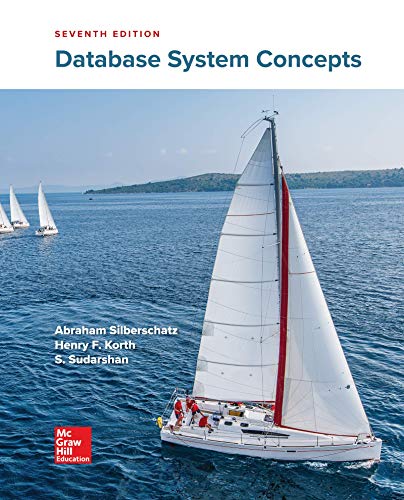
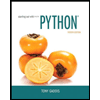
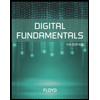
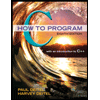
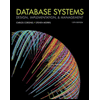
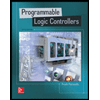