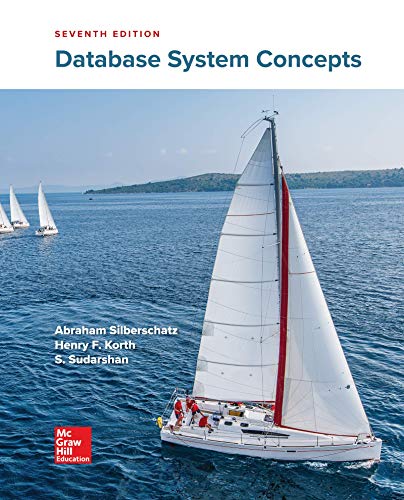
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Given string userString on one line, character charToFind on a second line, and integer strIndex on a third line, output "Found match" if the character at index strIndex of userString matches charToFind. Otherwise, output "No match". End with a newline.
Ex: If the input is:
warmth a 1
then the output is:
Found match
import java.util.Scanner;
public class MatchingChar {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String userString;
char charToFind;
int strIndex;
userString = scnr.nextLine();
charToFind = scnr.next().charAt(0);
strIndex = scnr.nextInt();
/* Your code goes here */
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- using System; class main { publicstaticvoid Main(string[] args) { Random rnd = new Random(); char randomChar = (char)rnd.Next('a','z'); Console.Write("Hi, my name is Stevie. I am thinking of a letter in the alphabet.\nGuess which letter I am thinking of! "); char guessedColor = Console.ReadLine()[0]; do { if(guessedColor != randomChar) { Console.WriteLine("Your guess is not correct.Please enter another letter "); guessedColor = Console.ReadLine()[0]; } if(guessedColor == randomChar) { Console.WriteLine("You guessed correctly!"); } }while(guessedColor != randomChar); } } Hello! This program is written in C# to play a guessing game with the user. The user guesses a letter of the alphabet and the program says if the guess is wrong or not. How would it be changed to guess words? For example, word in the animal category?arrow_forwardThis is the code that needs to be updated: .ORIG x3000 LD R0, NUM LD R1, DEN JSR GCD ADD R4, R1, #0 ADD R1, R2, #0 JSR DIVIDE ST R2, NUM ADD R0, R4, #0 JSR DIVIDE ST R2, DEN HALT; you can try other values for NUM and DEN by replacing these values in the simulatorNUM .FILL #10 DEN .FILL #3 ; Divide R0 by R1, putting quotient in R2 and remainder in R3DIVIDE ; Euclid's algorithm for GCD of R0 and R1, result in R2GCD .ENDarrow_forwardPlease create a flowchart for the following program: // Import Scanner classimport java.util.Scanner; // Create a class Trianglepublic class Main{ // Method used to check given three sides form a valid triangle or not public static boolean isTriangle(double a, double b, double c) { // If sum of any two sides is greater than third side if((a+b > c) && (a+c > b) && (b+c > a)) { // Print triangle is valid System.out.println("Three sides form a valid triangle."); // Return true return true; } // If any of the above condition is false else {// Print triangle is invalidSystem.out.println("Three sides form a invalid triangle.\n"); // Return false return false; } } // Method used to compute the area of triangle public static double triArea(double a, double b, double c) { // Compute s double s = (a + b + c) / 2; // Compute the area of triangle double area = Math.sqrt(s*(s - a)*(s - b)*(s - c)); // Return the…arrow_forward
- I have the following code: import java.util.*; import java.io.*; public class GradeBook { publicstaticvoidmain(String[] args)throwsIOException{ // TODO Auto-generated method stub File infile =newFile("students.dat"); Scanner in =newScanner(infile); while(in.hasNext()){ // Read information form file and create a student object and print String name = in.nextLine(); Student student =newStudent(name, in.nextLine()); for(int i =1; i <=4;++i){ student.setQuiz(i, in.nextInt()); } student.setMidtrmExm(in.nextInt()); student.setFinalExm(in.nextInt()); in.nextLine(); // Calculate grade and letter grade; double overallQuizScore=0.0,score=0.0; for(int i=1;i<=student.NUM_QUIZZES;i++) { overallQuizScore+=(student.getQuiz(i)/student.QUIZ_MAX_POINTS)*100; } overallQuizScore = (overallQuizScore/4)*0.30; score =…arrow_forwardPrint the two strings, firstString and secondString, in alphabetical order. Assume the strings are lowercase. End with newline. Sample output: capes rabbits 365076.2342078.qx3zay7 1 import java.util.Scanner; 3 public class OrderStrings { public static void main (String 0 args) { Scanner scnr = new Scanner(System.in); String firstString; String secondString; 4 5 7 8 firstString secondString = scnr.next(); 9 scnr.next(); 10 11 12 * Your solution goes here 13 } 15 } 14arrow_forwardWrite a program that performs a survey on beverages. The program should prompt for the next person until a lookout value of -1 is entered to terminate the program. Each person participating in the survey should choose their favorite beverage from the following list? 1. Coffee 2. Tea 3. Coke 4. Orange Juice.arrow_forward
- Need help with 8,9, and 10 if possible. Java Codingarrow_forwardFill in the blank with the correct answer/output. int[] nums = {78, 94, 850, 425, 375, 67}; int[] numsTen = {1,2,3,4,5,6,7,8,9,10}; double[] dblNums = {3.4, 2.75, 3.8, 4.0, 1.5} System.out.print( dblNums [0]); // LINE 1 1. wor www w System.out.print (nums [3]-25); // LINE 2 2. System.out.print{ nums [5]); // LINE 3 3. System.out.print( dblNums [3]); // LINE 4 4. System.out.print (numTens.length) ; // LINE 5 5. System.out.print (numsTen.length/2); // LINE 6 6. System.out.print (numsTen [6]); // LINE 7 7. System.out.print nums [0]); // LINE 8 8. System.out.print nums [9]); // LINE 9 9. System.out.print nums [6/2] ); // LINE 1o 10. System.out.print{ numsTen [2] ); // LINE 11 11. System.out.print{ dblNums [db1Nums.length-1]); // LINE 12 12. System.out.print nums [5/4]); // LINE 13 13. System.out.print{ nums [3]); // LINE 14 14.arrow_forwardHere is the question: Write an application that counts the total number of spaces contained in a quote entered by the user. Here is the code given: import java.util.*; public class CountSpaces2 { public static void main(String[] args) { // Write your code here } }arrow_forward
- You have to use comment function to describe what each line does import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class PreferenceData { private final List<Student> students; private final List<Project> projects; private int[][] preferences; private static enum ReadState { STUDENT_MODE, PROJECT_MODE, PREFERENCE_MODE, UNKNOWN; }; public PreferenceData() { super(); this.students = new ArrayList<Student>(); this.projects = new ArrayList<Project>(); } public void addStudent(Student s) { this.students.add(s); } public void addStudent(String s) { this.addStudent(Student.createStudent(s)); } public void addProject(Project p) { this.projects.add(p); } public void addProject(String p) { this.addProject(Project.createProject(p)); } public void createPreferenceMatrix() { this.preferences = new…arrow_forwardWrite a second convertToInches() with two double parameters, numFeet and numInches, that returns the total number of inches. Ex: convertToInches(4.0, 6.0) returns 54.0 (from 4.0 * 12 + 6.0). import java.util.Scanner; public class FunctionOverloadToInches { public static double convertToInches(double numFeet) {return numFeet * 12.0;} /* Your solution goes here */ public static void main (String [] args) {double totInches; totInches = convertToInches(4.0, 6.0);System.out.println("4.0, 6.0 yields " + totInches); totInches = convertToInches(5.8);System.out.println("5.8 yields " + totInches);}}arrow_forwardUse Java pl DataFileWords.txt: lovegentlensslovepeacelovekindnesslovepeaceunderstandingkindnessjoypeaceunderstandingfriendshipgentlenssgoodnesslovepeacegoodnesslovegentlensscontrolunderstandingunderstandinggentlensslovepeacepatiencecontrolkindnessgentlensslovepeacejoygoodnesspeacefriendshipgoodnesskindnesslovepeacegoodnesscontrolpatiencejoygentlensspatiencegentlensspatiencekindnessgoodnessjoygentlenssgentlenssarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
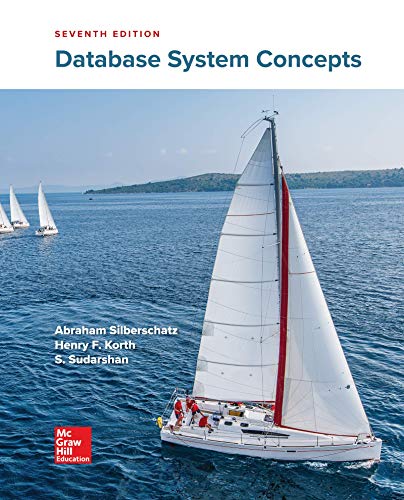
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
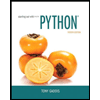
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
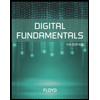
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
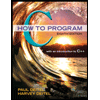
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
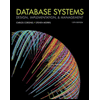
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
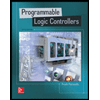
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education