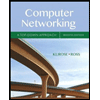
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Given the following class declaration (with prototypes):
class NumberArray
{
private:
double *aPtr; // pointer to a dynamic array of floating-point numbers
int arraySize; // size of the array
{
private:
double *aPtr; // pointer to a dynamic array of floating-point numbers
int arraySize; // size of the array
public:
NumberArray(int size, double value); // 2-parameter constructor
// allocates array and sets all numbers to value
NumberArray(int size, double value); // 2-parameter constructor
// allocates array and sets all numbers to value
void print() const;
void setValue(double value);
};
void setValue(double value);
};
Assume NumberArray has been implemented.
Consider the following program segment and rewrite the class declaration to address the issues indentified in a-d.
1: NumberArray first(3,10.5), second(5,6.9);
2: NumberArray third = second;
3: second = first;
4: NumberArray array[10];
2: NumberArray third = second;
3: second = first;
4: NumberArray array[10];
Rewrite the entire class declaration. Add the necessary member prototypes to correct the following problems:
a) In Line 2 the behavior is not what we expect. Identify what needs to be added to the class for the code to behave properly and add the prototype to the class declaration with comment.
b) In Line 3 the behavior is not what we expect. Identify what needs to be added to the class for the code to behave properly and add the prototype to the class declaration with comment.
c) In Line 4 a compile-time error will occur. Identify what needs to be added to the class for the code to compile and add the prototype to the class declaration with comment.
d) Finally, identify what needs to be added to the class to free dynamic memory and add the prototype to the class declaration with comment.
b) In Line 3 the behavior is not what we expect. Identify what needs to be added to the class for the code to behave properly and add the prototype to the class declaration with comment.
c) In Line 4 a compile-time error will occur. Identify what needs to be added to the class for the code to compile and add the prototype to the class declaration with comment.
d) Finally, identify what needs to be added to the class to free dynamic memory and add the prototype to the class declaration with comment.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- C#arrow_forwardAjva.arrow_forwardclass TenNums {private: int *p; public: TenNums() { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = i; } void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }};int main() { TenNums a; a.display(); TenNums b = a; b.display(); return 0;} Continuing from Question 4, let's say I added the following overloaded operator method to the class. Which statement will invoke this method? TenNums TenNums::operator+(const TenNums& b) { TenNums o; for (int i = 0; i < 10; i++) o.p[i] = p[i] + b.p [i]; return o;} Group of answer choices TenNums a; TenNums b = a; TenNums c = a + b; TenNums c = a.add(b);arrow_forward
- class Main { // this function will return the number elements in the given range public static int getCountInRange(int[] array, int lower, int upper) { int count = 0; // to count the numbers // this loop will count the numbers in the range for (int i = 0; i < array.length; i++) { // if element is in the range if (array[i] >= lower && array[i] <= upper) count++; } return count; } public static void main(String[] args) { // array int array[] = {1,2,3,4,5,6,7,8,9,0}; // ower and upper range int lower = 1, upper = 9; // throwing an exception…arrow_forwardSultan Qaboos University Department of Computer Science COMP2202: Fundamentals of Object Oriented Programming Assignment # 2 (Due 5 November 2022 @23:59) The purpose of this assignment is to practice with java classes and objects, and arrays. Create a NetBeans/IntelliJ project named HW2_YourId to develop a java program as explained below. Important: Apply good programming practices: 1- Provide comments in your code. 2- Provide a program design 3- Use meaningful variables and constant names. 4- Include your name, university id and section number as a comment at the beginning of your code. 5- Submit to Moodle the compressed file of your entire project (HW1_YourId). 1. Introduction: In crowded cities, it's very crucial to provide enough parking spaces for vehicles. These are usually multistory buildings where each floor is divided into rows and columns. Drivers can park their cars in exchange for some fees. A single floor can be modeled as a two-dimensional array of rows and columns. A…arrow_forwardPLEASE CODE IN PYTHON PLEASE USE NESTED CLASS FUNCTION Design a Point Class with attributes X and Y coordinates. The Class should have following functions: a) change the coordinates, b) return a 2 element list [x,y] c) print a Point object. d) return distance from this instance to a given [x,y] Also design a Line Class which has 2 Point attributes. The Line class should have functions for following behaviours: a) Return the length of the line. b) Print the equation of the line c) Find if this instance is equal in length to another line.arrow_forward
- Javaarrow_forwardFIX THE CODE PROVIDED BELOW Part 2 - Syntax Errors and Troubleshooting The code below has many syntax (and other) errors. Tasks:Compile the code and fix the errors one at a time. // Lab1a.java // This short class has several bugs for practice. // Authors: Carol Zander, Rob Nash, Clark Olson, you public class Lab1a { public static void main(String[] args) { compareNumbers(); calculateDist(); } publicstatic void compareNumbers() { int firstNum = 5; int secondNum; System.out.println( "Sum is: + firstNum + secondNum ); System.out.println( "Difference is: " + (firstNum - secondNum ); System.out.println( "Product is: " + firstNun * secondNum ); } public static void calculateDistance() { int velocity = 10; //miles-per-hour int time = 2, //hoursint distance = velocity * timeSystem.out.println( "Total distance is: " distanace); } } Part 3 - Print Statements and Simple Methods Use "print" and "println" statements, but use method calls to reduce the number of repeated "print"and "println"…arrow_forwardC++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
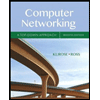
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
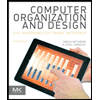
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
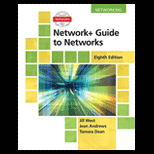
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
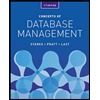
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
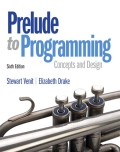
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
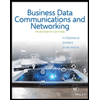
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY