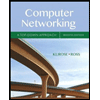
Hello! I am working my way through this problem for my Introduction to Java Programming Course. I think I understand the majority of it but I am have a very hard time understanding and developing the bounding box methods. I have developed the Point, Shape, Rectangle, Triangle and Circle classes but I am lost on how to calculate the bounding box coordinates for each of the hierarchy shapes. If someone would be kind enough to walk me through and explain this program, I would greatly appreciate it. Thank you kindly!
Problem #5:
Create a Point class to hold x and y values for a point. Create methods show(), add() and subtract() to display the Point x and y values, and add and subtract point coordinates.
Hint: Keep x and y separate in the calculation.
Create another class Shape, which will form the basis of a set of shapes. The Shape class will contain default functions to calculate area and circumference of the shape, and provide the coordinates (Points) of a rectangle that encloses the shape (a bounding box). These will be overloaded by the derived classes; therefore, the default methods for Shape will only print a simple message to standard output.
Create a display() function for Shape, which will display the name of the class and all stored information about the class (including area, circumference and bounding box).
Build the hierarchy by creating the Shape classes Circle, Rectangle and Triangle. Search the Internet for the rules governing these shapes, if necessary.
For these three Shape classes, create default constructors, as well as constructors whose arguments will initialize the shapes appropriately using the correct number of Point objects (i.e., Circle requires a Point center and a radius; Rectangle requires four Point vertices; and Triangle requires three Point vertices). Add error-checking to the constructors, such that they print a warning if the arguments do not conform to the appropriate shape.
Hint: Not all four-sided shapes are rectangles.
Also add a check to Rectangle, such that it tests for the special case of a “square” and prints an appropriate message if the test is true.
In main(), create several instances of each shape object and display the information for each object. Be sure to create at least one non-rectangle shape to demonstrate your error handling, and at least one square.

Step by stepSolved in 3 steps with 1 images

- Java is an OO language, which means that it focuses on objects. The existence of material things is generally accepted. You, as a student of computer science, have been tasked with differentiating between tangible items and their equivalents in object-oriented code. A project-oriented program is one that is designed with a particular end in mind.arrow_forwardAre you able to provide a definition of encapsulation as well as an explanation of its relevance in object-oriented programming?arrow_forwardThis next program is a little simpler, but it still illustrates some very critical program structures. It also illustrates an idea that is still used commonly, even if the mechanism has evolved with modern tools and alternative languages. We are referring to a menu-based help system. Modern help systems and menus are often represented using an integrated user environment; however, the core concepts behind the help system remain largely unchanged from this assignment. Create an interactive help system for Java programming. The system will incorporate a menu system that interacts with the user, and the appropriate help topic will be displayed, based on an input selection. This program is going to require some research on your part, as well as some programming in Java. Specifically, you will need to find definitions for the Java terms included in your help system. You can read the textbook or scour the Internet for the definitions. They should be no more than a few sentences each; nothing…arrow_forward
- Is there a big difference between the procedural and imperative programming styles and the declarative and functional programming styles?arrow_forwardJava is an OO language, which means that it focuses on objects. The existence of material things is generally accepted. As a student of computer science, you have been tasked with differentiating between tangible items and their equivalents in object-oriented code. Project-oriented programs are those that are designed to achieve a certain end result.arrow_forwardThe highlighted parts are to help me understand the assignment but it’s really challenging. And the Language that needs to be used is JAVAarrow_forward
- Is dynamic programming particularly helpful when it comes to picking a lot of options that are interrelated? Is there anything more that you think I need to be aware of?arrow_forwardWhen it comes to object-oriented programming, why is it required to put in more work and coding in order to avoid having classes that are needlessly linked? What are the three most important reasons for your stance?arrow_forwardWhich type of human-computer interface did you implement in your program in (a)above. Why the choice of such an interface?arrow_forward
- Use object-oriented design when it makes sense to do so. Structured design is a suitable option when the situation warrants it. Why is anything similar happening, etc.arrow_forwardDifferent opinions exist about object-oriented vs procedural programming. Each has its own benefits, drawbacks, and proponents. Discover and summarise the arguments on all sides of this subject. Which side do you agree with? Why?arrow_forwardLook at both the procedural and modular approaches to programming.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
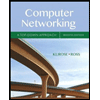
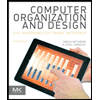
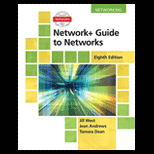
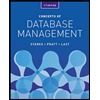
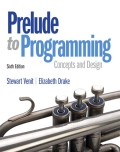
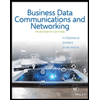