Hello, I need help programming a python code for my homework assignement. Any help would be appreciated. Thank you 1. Create a class called Account that a. includes one instance variable represents the account balance b. does initialize the account balance c. does provide two methods. Method Credit should add an amount to the current balance. Method Debit should withdraw money from the Account d. does provide a string representation when we call print on it, i.e., when print(account1) is called. 2. Define class SavingsAccount that inherits from Account class and a. includes an instance variable indicating the interest rate (percentage) assigned to the Account. b. does initialize its property, inherited from Account and defined in SavingsAccount, to the values given at the time of instantiation. c. provide method CalculateInterest that returns the amount of interest earned by an account. Method CalculateInterest should determine this amount by multiplying the interest rate by the account balance. d. does provide a string representation when we call print on it 3. Write a test class named AccountTest that demonstrates class Account’s capabilities and a. has a method "main" to test the Account and SavingsAccount classes b. The main method must instantiate the class into objects and test all of its methods in to get all instructions to run. c. call AccountTest.main() to run the program
Hello, I need help programming a python code for my homework assignement. Any help would be appreciated. Thank you
1. Create a class called Account that
a. includes one instance variable represents the account balance
b. does initialize the account balance
c. does provide two methods. Method Credit should add an amount to the current
balance. Method Debit should withdraw money from the Account
d. does provide a string representation when we call print on it, i.e., when
print(account1) is called.
2. Define class SavingsAccount that inherits from Account class and
a. includes an instance variable indicating the interest rate (percentage) assigned to
the Account.
b. does initialize its property, inherited from Account and defined in SavingsAccount, to
the values given at the time of instantiation.
c. provide method CalculateInterest that returns the amount of interest earned by an
account. Method CalculateInterest should determine this amount by multiplying the
interest rate by the account balance.
d. does provide a string representation when we call print on it
3. Write a test class named AccountTest that demonstrates class Account’s capabilities and
a. has a method "main" to test the Account and SavingsAccount classes
b. The main method must instantiate the class into objects and test all of its methods
in to get all instructions to run.
c. call AccountTest.main() to run the program

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

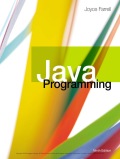
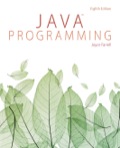
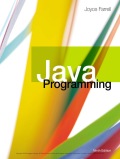
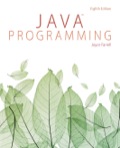