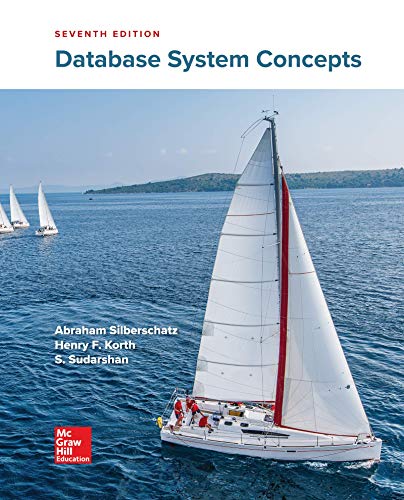
Hi, I need assistance please with my java code to implement the below problem. The Java program uses Genetic
The program is intended to work as follows:
- The user can input the string to guess.
- The user can enter the number or organisms in the population.
- The user can enter the number of generations to create.
- The user can enter the mutation probability.
- The user can observe the progress of the GA, for example print the best answer in every 10th generation.
- After the prescribed number of generations, the program should print best/final answer.
The program should “guesses” the string “We the people of the United States in order to perform a perfect union” and print result
import java.util.Scanner;
public class GATest {
public static void main(String[] arg) {
boolean repeat = true;
while(repeat) {
Scanner myScanner = new Scanner(System.in);
System.out.print("\nEnter string to guess--»");
String goal = myScanner.nextLine();
System.out.print("Enter number of organisms per generation--»");
int popSize = Integer.parseInt(myScanner.next());
System.out.print("Enter number of generations--»");
int generations = Integer.parseInt(myScanner.next());
System.out.print("Enter mutation probability--»");
double mutateProb = Double.parseDouble(myScanner.next());
System.out.println();
Population aPopulation = new Population(goal, popSize, generations, mutateProb);
aPopulation.iterate();
System.out.println("Repeat? y/n");
String answer = myScanner.next();
if (answer.toUpperCase().equals("Y"))
repeat = true;
else
repeat = false;
}
}
}
import java.util.Random;
public class Organism implements Comparable<Organism> {
String value, goalString;
double fitness;
int n;
Random myRandom = new Random();
public Organism(String goalString) {
value="";
this.goalString=goalString;
this.n= goalString.length();
for(int i=0; i<n; i++) {
int j = myRandom.nextInt(27);
if(j==26)
value=value+" ";
int which = myRandom.nextInt(2);
if(which==0)
j=j+65;
else
j=j+97;
value=value+ (char)j;
}
}
public Organism(String goalString, String value, int n) {
this.goalString=goalString;
this.value = value;
this.n=n;
}
public Organism() {
}
public String getValue() {
return this.value;
}
public void setValue(String value) {
this.value = value;
}
public String toString() {
return value +" "+ goalString+" "+getFitness(goalString);
}
public int getFitness(String aString) {
int count =0;
for(int i=0; i< this.n; i++)
if(this.value.charAt(i)== aString.charAt(i))
count++;
return count;
}
public int compareTo(Organism other) {
int thisCount, otherCount; thisCount=getFitness(goalString); otherCount=other.getFitness(goalString);
if (thisCount == otherCount)
return 0;
else if (thisCount < otherCount)
return 1;
else
return -1;
}
public Organism[] mate(Organism other) {
Random aRandom = new Random();
int crossOver = aRandom.nextInt(n);
String child1="", child2="";
for (int i=0; i< crossOver; i++) {
child1=child1+this.value.charAt(i);
child2 = child2+other.value.charAt(i);
}
for (int i= crossOver; i<n; i++) {
child1=child1+other.value.charAt(i);
child2=child2+this.value.charAt(i);
}
Organism[] children= new Organism[2];
children[0] = new Organism(goalString, child1,n);
children[1] = new Organism(goalString, child2, n);
return children;
}
public void mutate(double mutateProb) {
String newString="";
for (int i=0; i< n; i++) {
int k = myRandom.nextInt(100);
if (k/100.0 >mutateProb)
newString = newString+value.charAt(i);
else {
int j = myRandom.nextInt(27);
if (j==26)
newString=newString+" ";
else {
int which = myRandom.nextInt(2);
if (which ==0)
j=j +65;
else
j=j+97;
newString = newString+(char)j;
}
}
}
this.setValue(newString);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forwardMad Libs are activities that have a person provide various words, which are then used to complete a short story in unexpected (and hopefully funny) ways. Write a program that takes a string and an integer as input, and outputs a sentence using the input values as shown in the example below. The program repeats until the input string is quit and disregards the integer input that follows. Ex: If the input is: apples 5 shoes 2 quit 0 the output is: Eating 5 apples a day keeps the doctor away. Eating 2 shoes a day keeps the doctor away.arrow_forwardJava Programming: Question 12 Not sure how to do this question. Help of any input and output would be appreciated.arrow_forward
- Please &-. Write a Java program to check if a given string is a palindrome or not. A palindrome is a word, phrase, number, or other sequence of characters that reads the same backward as forward, ignoring spaces, punctuation, and capitalization. For example, "racecar" and "Madam" are palindromes, while "hello" and "Java" are not. Your program should take a string as input and return true if it's a palindrome, and false otherwise. Ik.?arrow_forwarda. Write a program that reads your id and full name and display. And also display the index of first letter and last letter of your full name. b. Modify above program, Declare a string course, let your program read course from user, check if course is not equal to comp2002, clear the string otherwise concatenate course and " Computer Science Department". C. Modify above program declare a string college="". If string college is empty then assign a new string "College of Science".arrow_forwardIn programming you work with text, sometimes you need to determine if a given string starts with or ends with certain characters. You can use two string methods to solve this problem: .startswith() and .endswith()?arrow_forward
- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT #2 so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #8. 8. Sum of Numbers in a String (page 610) Write a program that asks the user to enter a series of numbers separated by commas. Here are two examples of valid input: 7,9,10,2,18,6 8.777,10.9,11,2.2,-18,6 The program should calculate and display the sum of all the numbers. Input Validation 1. Make sure the string only contains numbers and commas (valid symbols are '0'-'9', '-', '.', and ','). 2. If the string is empty, a messages should be displayed, saying that the string is empty. Test Case 1 Enter a series of numbers separated only by commas or type QUIT to exit:\n7,9,10,2,18,6ENTERThe sum of those numbers is 52.00\nEnter a series of numbers separated only by commas or type QUIT to exit:\nENTEREmpty string.\nEnter a series of numbers separated only by…arrow_forwardthe function returns the result as a string if the expression is legal. the expression returns an empty string and then put the function public class Ez1MathEval {public String heavywtEval (String exprn) {return ""}}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
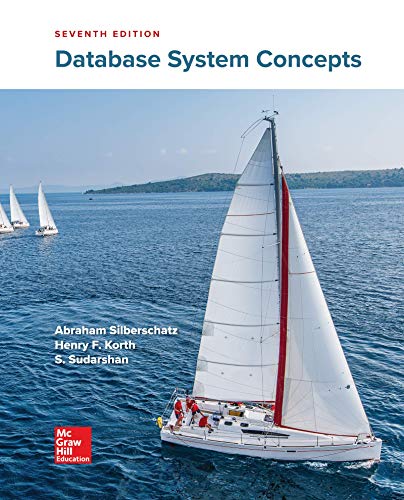
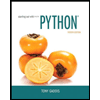
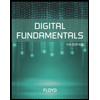
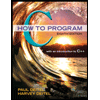
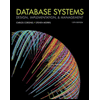
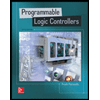