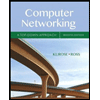
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Hi,
Im trying complete my lab for my
The purpose of this code is to simulate a coin flip and you can determine how many times you want the coin to be filped.
import random
def heads_or_tails(n):
for n in range(n):
if random.randint(0,1) == 1:
print("tails")
else:
print ("heads")
if __name__=="__main__":
random.seed(1)
number_of_flips = int(input())
heads_or_tails(number_of_flips)
i get this error when submitting
TypeError: heads_or_tails() missing 1 required positional argument: 'n'
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

Knowledge Booster
Similar questions
- Question #4: Re-write the program of Question #3 using data files. The input data numbers.txt contains (positive) integer numbers. Each time the program reads a number, check whether it is a prime and stores the result in the output file Results.txt. 373 373 is Prime!! 11 11 is Prime!! 2552 is NOT prime!! 5 is Prime!! 3561 is NOT prime!! 2552 3561 numbers.txt Results.txtarrow_forward2. A pentagonal number is defined as n(3n-1)/2 for n = 1, 2, ..., etc.. Therefore, the first few numbers are 1, 5, 12, 22, ... . Write a method with the following header that returns a pentagonal number: %3D public static int getPentagonalNumber(int n) For example, getPentagonalNumber(1) returns 1 and getPentagonalNumber(2) returns 5. Write a test program that uses this method to display the first 100 pentagonal numbers with 10 numbers on each line. Numbers are separated by exactly one space.arrow_forwardYou will build a program to manage a race car in this assessment. The code will consist of a for-loop method that will run a car around a race track and cause “wear and tear” on the car. The car will perform x number of laps and will have to make pitstops when it either runs out of gas or needs a tire replacement. The for loop will be simple def wear_tires(car): tire -=1 def use_gas(car): gas -=1 c = Car() # You need to make this part for i in range(100): if the car needs a pitstop: perform pitstop else: wear_tires(c) use_gas(c) # Display the current cars wear in a much better format print(c.tire) print(c.gas) print(“Current Lap is: “ , i) For this task, you will have to use this for loop and build out the two methods in the main Python program. To use the above code, you must build two pieces of information. An abstract Vehicle class A Car class A RaceCar class Each with its methods and variables. This programming will involve using inheritance and abstraction to make a race car…arrow_forward
- Random Numbers: Most computer programs do the same thing every time they run; programs like that are called deterministic. Usually, determinism is a good thing, since we expect the same calculation to yield the same result. But for some applications, we want the computer to be unpredictable. Games are an obvious example, but there are many others, like scientific simulations. We have already seen java.util.Random, which generates pseudorandom numbers. The method nextInt takes an integer argument, n, and returns a random integer between 0 and n - 1. Assignment: For this assignment you will write a program in java to simulate a guessing game. Your program will generate a random number in a certain range (let the user define the range) and ask the user to guess what number it is. Give the user a certain number of tries while you narrow down the possibilities. For example, let's say the number generated is 67 and the user has 5 tries, this could be your program's output: This is a guessing…arrow_forwardWrite code that outputs variable numDogs as follows. End with a newline. Ex: If the input is: 3 the output is: Dogs: 3 I tried to get the output of 6 by its wrong everytime.arrow_forwardPseudocode for this Python code (I wrote one but it does not match): import random def rollDice(): num1 = random.randint(1, 6) num2 = random.randint(1, 6) return num1, num2 def determine_win_or_lose(num1, num2): total = num1 + num2 print(f"You rolled {num1} + {num2} = {total}") if total == 2 or total == 3 or total == 12: return 0 elif total == 7 or total == 11: return 1 else: print(f"Point is {total}") return determinePointValueResult(total) def determinePointValueResult(pointValue): total = 0 result = -1 while total != 7 and total != pointValue: num1, num2 = rollDice() total = num1 + num2 print(f"You rolled {num1} + {num2} = {total}") if total == pointValue: result = 1 elif total == 7: result = 0 return result def main(): n = int(input("Enter the number of games to play: ")) i = 0 winCounter = 0 loseCounter = 0 while i < n: num1,…arrow_forward
- public static int test(int n, int k) { if (k == 0 || k == n) { return 1; } else if (k>n) { return 0; } else { return test(n-1, k-1) + test(n-1, k); } ww For each of the following calls, indicate the output that is produ test(7,5); test(6,4); test(4,5); test(8,3); test(5,5);arrow_forwardRandom Numbers: Most computer programs do the same thing every time they run; programs like that are called deterministic. Usually, determinism is a good thing, since we expect the same calculation to yield the same result. But for some applications, we want the computer to be unpredictable. Games are an obvious example, but there are many others, like scientific simulations. We have already seen java.util.Random, which generates pseudorandom numbers. The method nextint takes an integer argument, n, and returns a random integer between 0 and n - 1. Assignment For this assignment you will write a program in java to simulate a guessing game. Your program will generate a random number in a certain range (let the user define the range) and ask the user to guess what number it is. Give the user a certain number of tries while you narrow down the possibilities. For example, let's say the number generated is 67 and the user has 5 tries, this could be your program's output: This is a guessing…arrow_forwardUsing the Python language answer the following questions below. Please tell me what program you use if it is IDLE or Atom or a python website please provide the website you use.arrow_forward
- Understanding ifStatements Summary In this lab, you complete a prewritten Java program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: The charge for all signs is a minimum of $35.00. The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. If the sign is made of oak, add $20.00. No charge is added for pine. Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering. Instructions 1. Ensure the file named HouseSign.java is open. 2. You need to declare variables for the following, and initialize them where specified: A variable for the cost of the sign initialized to 0.00 (charge). A variable for the number of characters initialized to 8 (numChars). A variable for the color of the characters initialized to "gold" (color). A variable for the…arrow_forwardONLY IF STATEMENTS ALLOWED!!!! NO RANGE FUNCTION, NO SETS, NO LISTS, NO LOOPS, ETC Angela loves reading books. She recently started reading an AI generated series called “Harry Trotter”. Angela is collecting books from the series at her nearest bookstore. Since the series is AI generated, the publishers have produced an infinite collection of the books where each book is identified by a unique integer. The bookstore has exactly one copy of each book. Angela wants to buy the books in the range [l,r], where l ≤ r. As an example, the range [−3,3] means that Angela wants to buy the books − 3, − 2, − 1, 0, 1, 2, and 3. Dan also loves the series (or maybe annoying Angela – who knows, really), and he manages to sneak into the bookstore very early to buy all of the books in the range [d,u], where d ≤ u. When Angela later visits, sadly she will not find those books there anymore. For example, if Angela tries to buy books [−2,3] and Dan has bought books [0,2], Angela would only receive books…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
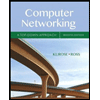
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
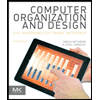
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
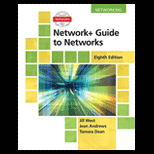
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
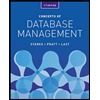
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
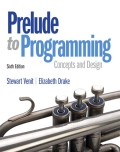
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
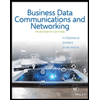
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY