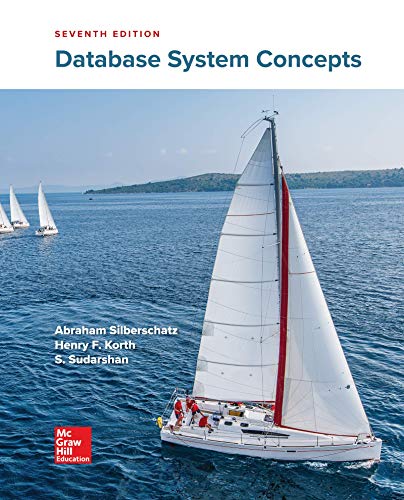
Concept explainers
How can I apply this python code wherein I have an input n. Then create a list from 2, n and remove the multiples of n.
Example:
Input: 10
Output: 2, 3, 4, 5, 6, 7, 8, 9
def createList(n):
#Base Case/s
#TODO: Add conditions here for your base case/s
#if <condition> :
#return <value>
#Recursive Case/s
#TODO: Add conditions here for your recursive case/s
#else:
#return <operation and recursive call>
#remove the line after this once you've completed all the TODO for this function
return []
def removeMultiples(x, arr):
#Base Case/s
#TODO: Add conditions here for your base case/s
#if <condition> :
#return <value>
#Recursive Case/s
#TODO: Add conditions here for your recursive case/s
#else:
#return <operation and recursive call>
#remove the line after this once you've completed all the TODO for this function
return []

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Lab Goal : This lab was designed to teach you more about recursion. Lab Description : Take a string and remove all occurrences of the word chicken and count how many chickens were removed. Keep in mind that removing a chicken might show a previously hidden chicken. You may find substring and indexOf useful. achickchickenen - removing the 1st chicken would leave achicken behindachicken - removing the 2nd chicken would leave a behindSample Data : itatfunitatchickenfunchchickchickenenickenchickchickfunchickenbouncetheballchickenSample Output : 01302arrow_forwardPart (1) Write a recursion method named CalculateSeries, that will receive one integer valuen as parameter. The method will calculate and return the term n in the following series: T(0)= 0 T(n)= 2 T(n-1) +1 Part (2) Write the main method to test your method. Enter n >> 5 T(5) = 31 %3Darrow_forwardWrite a recursive function that returns the largest integer in a list. Write a test program that prompts the user to enter a list of integers and displays the largest element.arrow_forward
- What makes HTML stand apart from the rest?arrow_forwardplease solve in python and give code. thankzzarrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forward
- Question in image Please explain the algorithm with the answer. Python programmingarrow_forwardParallel Lists JumpinJive.py >- Terminal Summary 1 # JumpinJava.py - This program looks up and prints t and prices of coffee orders. 2 # Input: In this lab, you use what you have learned about parallel lists to complete a partially completed Interactive Python program. 3 # Output: Name and price of coffee orders or error if add-in is not found 4 The program should either print the name and price for a coffee add-in from the Jumpin' Jive 5 # Declare variables. Coffee Shop or it should print the message "Sorry, we do not carry that.". 6 NUM_ITEMS = 5 # Named constant 8 # Initialized list of add-ins 9 addIns = ["Cream", "Cinnamon", "Chocolate", "Amarett "Whiskey"] Read the problem description carefully before you begin. The data file provided for this lab includes the necessary input statements. You need to write the part of the program that searches for the name of the coffee add-in(s) and either prints the name and price of the add-in or prints 10 the error message if the add-in is not…arrow_forwardComplete the recursive function remove_last which creates a new list identical to the input list s but with the last element in the sequence that is equal to x removed. Hint: Remember that you can use negative indexing on lists! For example 1st [-1] refers to the last element in a list 1st, lst [-2] refers to the second to last element... def remove_last(x, s): """Create a new list that is identical to s but with the last element from the list that is equal to x removed. >>> remove_last(1, []) [] >>> remove_last(1, [1]) [] >>> [1] >>> [2] remove_last(1, [1,1]) remove_last(1, [2,1]) >>> remove_last(1, [3,1,2]) [3, 2] >>> remove_last(1, [3,1,2,1]) [3, 1, 2] >>> remove_last (5, [3, 5, 2, 5, 11]) [3, 5, 2, 11] IIIII "*** YOUR CODE HERE ***" Illustrated here is a more complete doctest that shows good testing methodology. It is a little cumbersome as documentation, but you'll want to think about it for your projects. Test every condition that might come up. Then you won't be surprised when…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
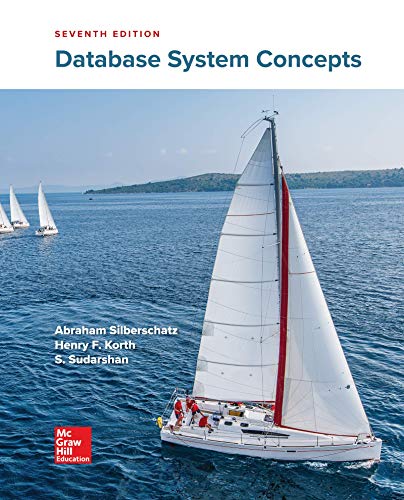
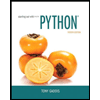
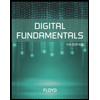
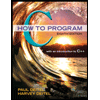
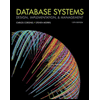
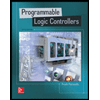