Exercise-3: Write a recursive and iterative methods to convert a decimal number to its binary equivalent string. • The iterative algorithm (in pseudo-code) for converting a decimal integer into a binary integer as follows: 1. If the integer is 0 or 1, its binary equivalent is 0 or 1. 2. If the integer is greater than or equal to 2 do the following: 3. Divide the integer by 2. 4. Separate the result into a quotient and remainder. 5. Divide the quotient again and repeat the process until the quotient is zero. 6. Write down all remainders in reverse order as a string. 7. This string is the binary equivalent of the given integer. • // Recursive decimal to binary method public static String dec2binRecursive(int n) { if (n<2) return n+ else return dec2binRecursive(n/2) + n%2; }
Exercise-3: Write a recursive and iterative methods to convert a decimal number to its binary equivalent string. • The iterative algorithm (in pseudo-code) for converting a decimal integer into a binary integer as follows: 1. If the integer is 0 or 1, its binary equivalent is 0 or 1. 2. If the integer is greater than or equal to 2 do the following: 3. Divide the integer by 2. 4. Separate the result into a quotient and remainder. 5. Divide the quotient again and repeat the process until the quotient is zero. 6. Write down all remainders in reverse order as a string. 7. This string is the binary equivalent of the given integer. • // Recursive decimal to binary method public static String dec2binRecursive(int n) { if (n<2) return n+ else return dec2binRecursive(n/2) + n%2; }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
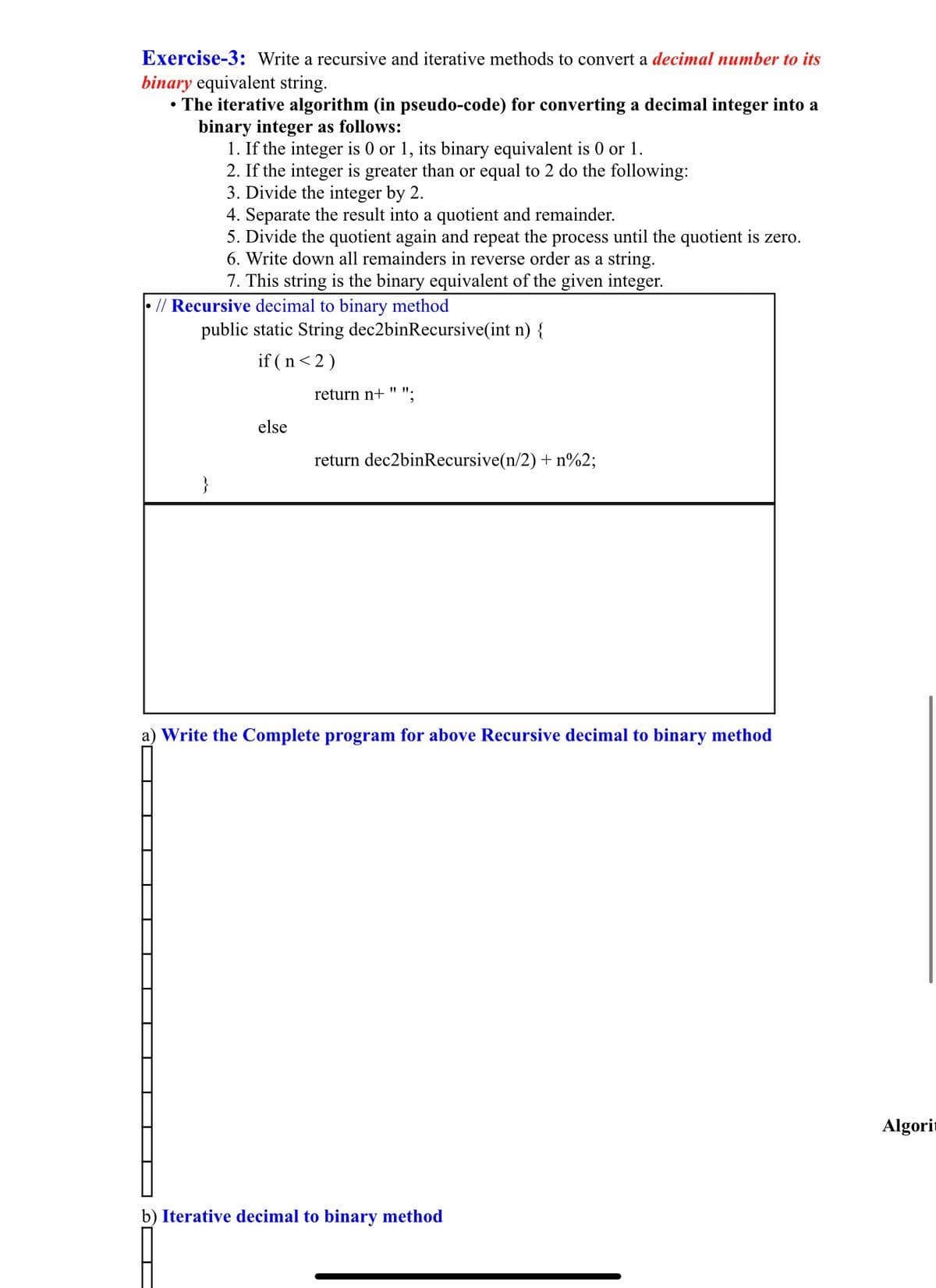
Transcribed Image Text:Exercise-3: Write a recursive and iterative methods to convert a decimal number to its
binary equivalent string.
The iterative algorithm (in pseudo-code) for converting a decimal integer into a
binary integer as follows:
1. If the integer is 0 or 1, its binary equivalent is 0 or 1.
2. If the integer is greater than or equal to 2 do the following:
3. Divide the integer by 2.
4. Separate the result into a quotient and remainder.
5. Divide the quotient again and repeat the process until the quotient is zero.
6. Write down all remainders in reverse order as a string.
7. This string is the binary equivalent of the given integer.
// Recursive decimal to binary method
public static String dec2binRecursive(int n) {
if (n<2)
return n+ " ".
else
return dec2binRecursive(n/2) + n%2;
}
a) Write the Complete program for above Recursive decimal to binary method
Algorit
b) Iterative decimal to binary method
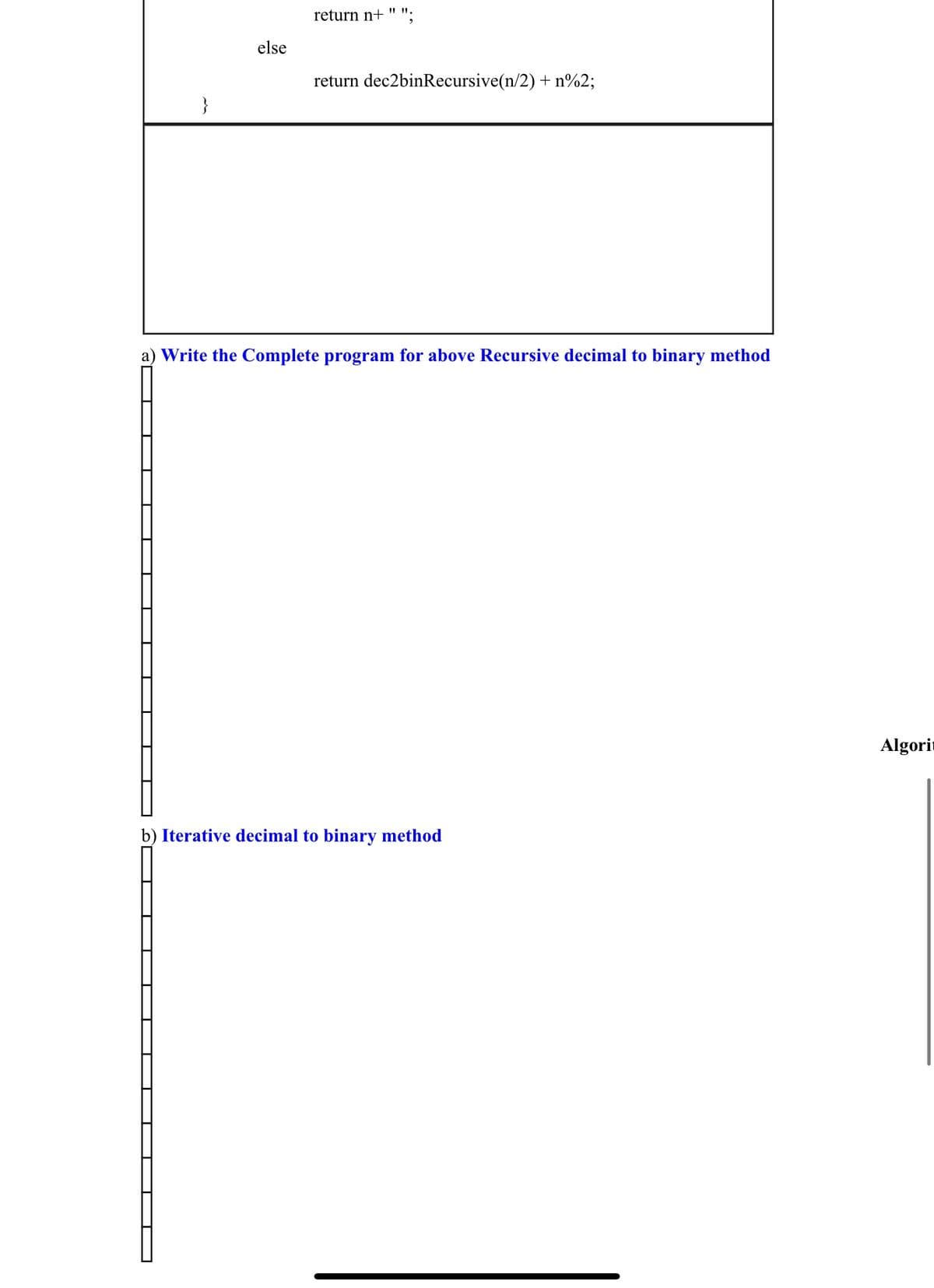
Transcribed Image Text:II II.
return n+ " ";
else
return dec2binRecursive(n/2) + n%2;
}
a) Write the Complete program for above Recursive decimal to binary method
Algorin
b) Iterative decimal to binary method
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
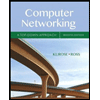
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
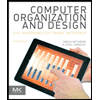
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
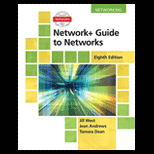
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
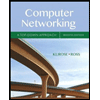
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
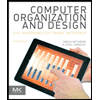
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
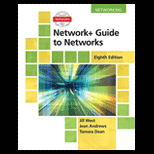
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
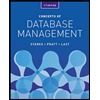
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
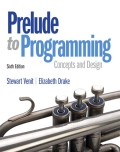
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
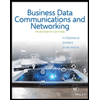
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY