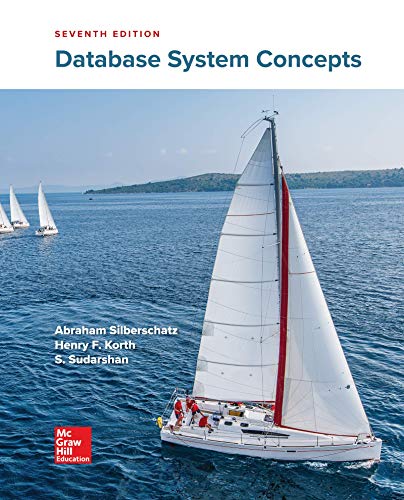
How do I fix the code in Java.
Error
FinalExamAnswers.java:3: error: class FinalExamAnswer is public, should be declared in a file named FinalExamAnswer.java
public class FinalExamAnswer{
^
Code:
import java.util.Scanner;
public class FinalExamAnswer{
public static void main(String [] args)
{
manipulateString(); //calls function
}
//your code here
public static void manipulateString()
{
Scanner sc=new Scanner(System.in); //create Scanner instance
System.out.print("Enter a sentence: ");
String sentence=sc.nextLine(); //input a sentence
String[] words=sentence.split(" "); //split the sentence at space and store it in array
for(int i=0;i<words.length;i++) //i from 0 to last index
{
if(i%2==0) //if even index
words[i]=words[i].toUpperCase(); //converted to upper case
else //if odd index
words[i]=words[i].toLowerCase(); //converted to lower case
}
for(int i=words.length-1;i>=0;i--) //i from last index to 0
{
System.out.print(words[i]+" "); //print word at index i and space
}
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- How do I make the code run properly? Code: import java.util.*; import java.util.Arrays; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; // default constructor public Movie() { this.movieName = "Flick"; this.numMinutes = 0; this.isKidFriendly = false; this.numCastMembers = 0; this.castMembers = new String[10]; } // overloaded parameterized constructor class movie{public static void main(String args[]){Movie obj=new Movie();Movie obj1=new Movie(obj.getMovieName(),obj.getNumMinutes(),obj.isKidFriendly(),obj.getCastMembers());System.out.println("Default Constructor: "+obj.getNumCastMembers());System.out.println("Overloaded Constructor: "+obj1.getNumCastMembers());}} // set the number of minutes public void setNumMinutes(int numMinutes) { this.numMinutes = numMinutes; } // set the movie name public void setMovieName(String movieName) { this.movieName = movieName; } // set if the…arrow_forwardimport java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forwardpreLabC.java 1 import java.util.Random; 2 import java.util.StringJoiner; 3 4- public class preLabC { 5 6- 7 8 9 10 11 12 13 14 15 16 17 18 19 20 } public static String myMethod(MathVector inputVector) { String vectorStringValue = new String(); // empty String object System.out.println("here is the contents object, inputVector: + inputVector); // get a String out of that MathVector object. Do it here. On the next line. // return the double value here. return vectorStringValue; "1 ▸ Compilation Description A MathVector object will be passed to your method. Return its contents as a String. If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector object as a String. This makes it useful for displaying to the user. /** * Returns a String representation of this vector. The String should be in the format "[1, 2, 3]" * * @return a String representation of this vector * @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method. */…arrow_forward
- 8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardJava code about Enrollment System Please help. So this is the code: import java.util.Scanner; class User { String username; String password; String role; public User(String username, String password, String role) { this.username = username; this.password = password; this.role = role; } public String getUsername() { return username; } public String getPassword() { return password; } public String getRole() { return role; } } class Course { String courseCode; String courseTitle; int units; public Course(String courseCode, String courseTitle, int units) { this.courseCode = courseCode; this.courseTitle = courseTitle; this.units = units; } public String getCourseCode() { return courseCode; } public String getCourseTitle() { return courseTitle; } public int getUnits() { return units; } } class EnrollmentSystem { User[] users = new User[10]; int userCount = 0; Course[] courses = new Course[10]; int courseCount = 0; Scanner sc = new Scanner(System.in); public void…arrow_forwardWrite a Fraction class that works with the FractionMath program above. It should use your Fraction.java file to run.Main Program:public class FractionMath{public static void main(String[] arguments){// create four fractions accountsFraction w = new Fraction();Fraction x = new Fraction(3,4);Fraction y = new Fraction(2,5);Fraction z = new Fraction(6,0);System.out.println("*** Does the toStringMethod work?"); System.out.println("First fraction : " + w); System.out.println("Second fraction : " + x); System.out.println("Third fraction : " + y); System.out.println("Forth fraction : " + z); System.out.println(); // Does the get() method work? System.out.println("*** Does the get() methods work?"); System.out.println("w = " + w.getNumerator() + " over " + w.getDenominator()); System.out.println();// Can we change the values correctly nameSystem.out.println("*** Does the set() methods work?");System.out.println("w fraction Before : " + w); w.setNumerator(22);System.out.println("w fraction After…arrow_forward
- PlayerRoster.java:29: error: class PlayerRosterApp is public, should be declared in a file named PlayerRosterApp.java public class PlayerRosterApp { ^ 1 error import java.util.Scanner; class Player { private int jerseyNumber; private int playerRating; public Player(int jerseyNumber, int playerRating) { this.jerseyNumber = jerseyNumber; this.playerRating = playerRating; } public int getJerseyNumber() { return jerseyNumber; } public void setJerseyNumber(int jerseyNumber) { this.jerseyNumber = jerseyNumber; } public int getPlayerRating() { return playerRating; } public void setPlayerRating(int playerRating) { this.playerRating = playerRating; }} public class PlayerRosterApp { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int rosterSize = 5; Player[] roster = new Player[rosterSize]; // Prompt the…arrow_forwardInheritanceDemo.java: // StudentName Today's Date public class InheritanceDemo { public static void main(String[] args) { KleenexBox kb1 = new KleenexBox(); KleenexBox kb2 = new KleenexBox(100); Box justABox = new Box(); System.out.println("justABox has area " + BoxArea(justABox)); System.out.println("kb1 has area " + BoxArea(kb1)); System.out.println("kb2 has this many square inches of tissue: " + KleenexArea(kb2)); } public static double BoxArea (Box b) { return b.width * b.length; } public static double KleenexArea (KleenexBox a) { return a.width * a.length * a.numTissues; } } Box.java: // Student Name Today's Date public class Box { public double width; public double length; public String label; } KleenexBox.java: // Student's Name Today's Date public class KleenexBox extends Box { public int numTissues; // A new Kleenex box public KleenexBox() { numTissues = 50; length = 8.0; width = 5.0; } // A new Kleenex box with a specific number of tissues public…arrow_forwardWhats the output?arrow_forward
- I need this debugged. Thanks in advance. // Application looks up home price// for different floor plans// allows upper or lowercase data entryimport java.util.*;public class DebugEight3{public static void main(String[] args){Scanner input = new Scanner(System.in);String entry;char[] floorPlans = {'A','B','C','a','b','c'};int[] pricesInThousands = {145, 190, 235};char plan;int x, fp = 99;String prompt = "Please select a floor plan\n" +"Our floorPlanss are:\n" + "A - Augusta, a ranch\n" +"B - Brittany, a split level\n" +"C - Colonial, a two-story\n" +"Enter floorPlans letter";System.out.println(prompt);entry = input.next();plan = entry.charAt(1);for(x = 0; x < floorPlans.length; ++x)if(plan == floorPlans[x])x = fp;if(fp == 99)System.out.println("Invalid floor plan code entered");else{if(fp >= 3)fp = fp - 3;System.out.println("Model " +plan + " is priced at only $" +pricesInThousands[fp] + ",000");}}}arrow_forwardjava 1 error with my code pls help correct my codearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
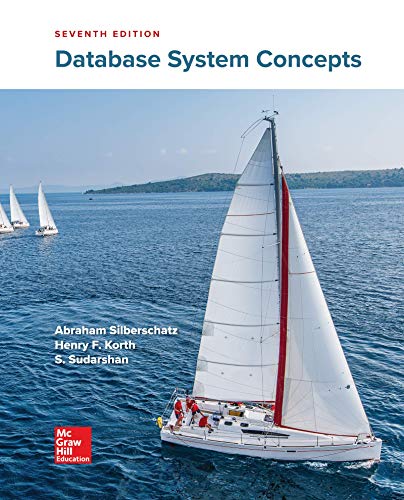
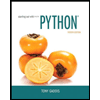
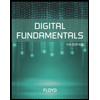
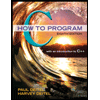
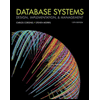
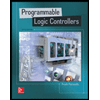