how to fix error: java: unreported exception java.io.IOException; must be caught or declared to be thrown import java.io.*; public class FileOperator { private File m_file; public FileOperator() { String fileName = "employeeData.txt"; m_file = new File(fileName); } public void writeFile(Employee[] employees) { FileWriter filewriter = new FileWriter (m_file, true); try { for(int i=0; i < employees.length ; i++) if (employees[i] instanceof Faculty) { String str = "F-" + employees[i].getPaycheck(); filewriter.write(str); } else { String str = "S-" + employees[i].getPaycheck(); filewriter.write(str); } filewriter.close(); } catch(IOException e) { System.out.println("File not found"); } } }
import java.io.*;
public class FileOperator
{
private File m_file;
public FileOperator()
{
String fileName = "employeeData.txt";
m_file = new File(fileName);
}
public void writeFile(Employee[] employees)
{
FileWriter filewriter = new FileWriter (m_file, true);
try
{
for(int i=0; i < employees.length ; i++)
if (employees[i] instanceof Faculty)
{
String str = "F-" + employees[i].getPaycheck();
filewriter.write(str);
}
else
{
String str = "S-" + employees[i].getPaycheck();
filewriter.write(str);
}
filewriter.close();
}
catch(IOException e)
{
System.out.println("File not found");
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

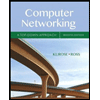
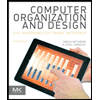
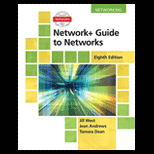
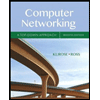
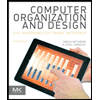
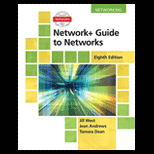
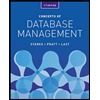
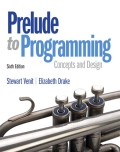
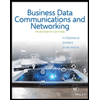