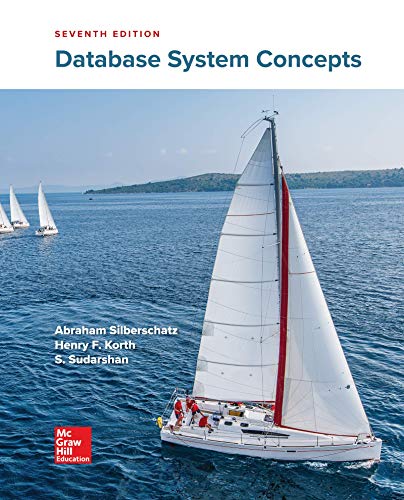
Concept explainers
Please help me fix my errors in Python.
def read_data(filename):
try:
with open(filename, "r") as file:
return file.read()
except Exception as exception:
print(exception)
return None
def extract_data(tags, strings):
data = []
start_tag = f"<{tags}>"
end_tag = f"</{tags}>"
while start_tag in strings:
try:
start_index = strings.find(start_tag) + len(start_tag)
end_index = strings.find(end_tag)
value = strings[start_index:end_index]
if value:
data.append(value)
strings = strings[end_index + len(end_tag):]
except Exception as exception:
print(exception)
return data
def get_names(string):
names = extract_data("name", string)
return names
def get_descriptions(string):
descriptions = extract_data("description", string)
return descriptions
def get_calories(string):
calories = extract_data("calories", string)
return calories
def get_prices(string):
prices = extract_data("price", string)
return prices
def display_menu(names, descriptions, calories, prices):
for i in range(len(names)):
print(f"{names[i]} - {descriptions[i]} - {calories[i]} - {prices[i]}")
def display_stats(names, calories, prices):
try:
total_items = len(names)
average_calories = (sum(map(int, calories)) / total_items if
total_items > 0 else 0)
average_price = (sum([float(price[1:]) for price in prices]) /
total_items if total_items > 0 else 0)
if names and calories and prices:
print(f"\n{total_items} items - {average_calories:.1f} average calories - ${average_price:.2f} average price")
except:
print("Please insert a valid number.")
def main():
filename = "simple.xml"
string = read_data(filename)
if string:
names = get_names(string)
descriptions = get_descriptions(string)
calories = get_calories(string)
prices = get_prices(string)
if names and prices and descriptions and calories:
display_menu(names, descriptions, calories, prices)
display_stats(names, calories, prices)
main()
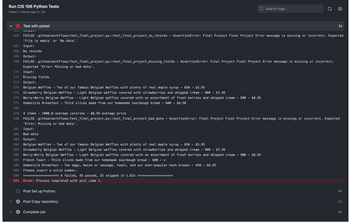

Step by stepSolved in 5 steps with 4 images

- Python S3 Get File In the Python file, write a program to get all the files from a public S3 bucket named coderbytechallengesandbox. In there there might be multiple files, but your program should find the file with the prefix and cb - then output the full name of the file. You should use the boto3 module to solve this challenge. You do not need any access keys to access the bucket because it is public. This post might help you with how to access the bucket. Example Output ob name.txt Browse Resources Search for any help or documentation you might need for this problem. For exampler array indexing, Ruby hash tables, etc.arrow_forwardCompliant/Noncompliant Solutions The following code segment was provided in Secure Coding Guidelines for Java SE: void readData() throws IOException{ BufferedReader br = new BufferedReader(new InputStreamReader( new FileInputStream("file"))); // Read from the file String data = br.readLine();} The code is presented as a noncompliant coding example. For this assignment, identify two compliant solutions that can be utilized to ensure the protection of sensitive data. Provide a detailed explanation of factors, which influence noncompliant code and specifically how your solutions are now compliant. Your explanation should be 2-3 pages in length. Submit the following components: Word document with appropriate program analysis for your compliant solutions and source code in the Word file. Submit your .java source code file(s). If more than 1 file, submit a zip file.arrow_forwardException in thread "main" java.lang.NumberFormatException: For input string: "x" for Java code public class Finder { //Write two recursive functions, both of which will parse any length string that consists of digits and numbers. Both functions //should be in the same class and have the following signatures. //use the if/else statement , Find the base case and -1 till you get to base case //recursive function that adds up the digits in the String publicstaticint sumIt(String s) { //if String length is less or equal to 1 retrun 1. if (s.length()<= 1){ return Integer.parseInt(s); }else{ //use Integer.praseInt(s) to convert string to Integer //returns the interger values //else if the CharAt(value in index at 0 = 1) is not equal to the last vaule in the string else {//return the numeric values of a char value + call the SumIt method with a substring = 1 return Character.getNumericValue(s.charAt(0) ) + sumIt(s.substring(1)); } } //write a recursion function that will find…arrow_forward
- C++ Code dynamicarray.h and dynamicarray.cpparrow_forwardcreateDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forwardFilename: runlength_decoder.py Starter code for data: hw4data.py Download hw4data.py Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:def decode(data : list) -> list: Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run. For example, using Python lists, the initial list: [‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’] would be encoded as: ['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2] So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the…arrow_forward
- Could you help me with this one too, please Regex, APIs, BeautifulSoup: python import requests, refrom pprint import pprintfrom bs4 import BeautifulSoup complete the missing bodies of the functions below: def group_chat(text_message, friend):"""Question 2- Your friends are blowing up your group chat. Given a string of text messagesfrom your friends and a specific friend's name, return the first text messagethey sent, excluding their name.- Each text message ends with either a ?, !, or .- Your code must be written in one line. Args:text_message (astr)friend (astr)Returns:str of first match>>> text_message = "Madison: How are you guys going today?" + \"Anna: I'm doing pretty well!" + \"Madison: That's good to hear. How is everyone else?">>> friend = "Madison">>>group_chat(text_message, friend)How are you guys going today?"""pass test code: # text_message = "Madison: How are you guys going today?" + \# "Anna: I'm doing pretty well!" + \#…arrow_forwardEvaluate the following functions: 1. prune [[[3],[1,2,4]],[[1,3],[3,4]]] 2. fix prune [[[5],[6,8]],[[7],[7,8]]] 3. blocked [[[3,4],[],[3]],[[1],[1,2],[1]]] 4. expand [[[1,2],[3]],[[4),[1,2]]arrow_forwardWrite a code in c++ to open a file called romania.txt for appending, only for a relevant code. You may assign any file objectarrow_forward
- Warning: Not copy code again and again. Narrow_forwardMilestone #1 - Ask Quiz Type In a file called main.py, implement a program that asks the user for a quiz type and prints the file name for that quiz type. The program should have one input prompt: the integer value of the personality quiz number. Your program should print the welcome message and the list of the personality quizzes, ask for the test number, and then print the file name. You are required to use the provided dictionary with the personality quizzes names. The list of the personality quizzes should be printed in alphabetical order. See example below. Welcome to the Personality Quiz! What type of Personality Quiz do you want to run? 1 BabyAnimals 2 Brooklyn99 3 Disney 4 Hogwarts 5 MyersBriggs 6 Sesame Street 7 - StarWars 8 - Vegetablesarrow_forward1-Write a JAVA program that reads an array from input file and invokes twodifferent methods Sort and Max ,that sorts the elements of the array and findsthe Max element and writes out the resulted array in to output file . Usetwo interfaces for methods and throw Exception Handling for Out Of Boundindex for the arrayarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
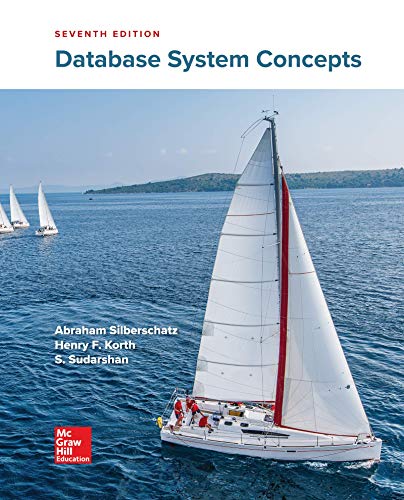
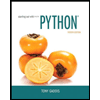
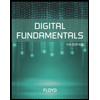
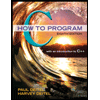
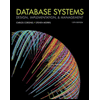
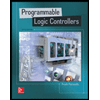