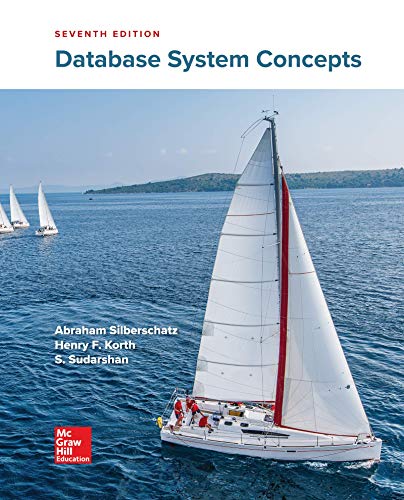
Concept explainers
PYTHON:
I am working on a problem using inheritance (OOP). The first question, Create a class named Circle that will inherit properties and methods from a class named Ellipse, and you do not want to add any other properties or methods to the class, my answer was:
class Circle(Ellipse):
pass
For the next problem, I need to write a workable code defining the class Ellipse with a reasonable number of properties and methods. Mostly it is a practice to understand how inheritance works. I am having trouble figuring out where to go from here so I have a program to print my answers out. This is what I have so far. Any help would be appreciated.
import math
class Ellipse:
def _init_(self, length, width):
self.length = length
self.width = width
def area(self):
return 3.14 * self.length * self.width
def perimeter(self):
return 3.14 * (self.length * self.width)
class Circle(Ellipse):
def _init_(self, radius):
super()._init_(radius, radius)

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 2 images

- Need it in the java language, doesn't have to be perfect. If you can do the whole program that would be best but if not an outline would suffice so I can get a good idea of how to finish the program. Thanks!arrow_forwardaccessor methods for each of the instance variables A local taxi company has decided to expand and not only have taxis that go on land (i.e., cars), but also taxis that go on water (i.e., boats). You have been asked to design a number of classes to represent the various taxi fares (trips with passengers) and their various fee structures, by using inheritance in Python. a computeCharges method that returns the total charges for the cab fare, using the instance variables and the defined constants (i.e., kms multiplied by the cost per km, plus minutes multiplied by the cost per minute) Please read all parts of this question below before starting the question. Include the completed code for all parts of this question in a file called taxi_company.py- NOTE: To implement encapsulation, all of the instance variables/fields should be considered private. Question 3 (a) Design and implement a class called TaxiFare that includes the following instance variable(s) and method(s) for an object.…arrow_forwardURGENT In python You just started your summer internship with old jalopy auto Rentals based in Lenior. North Carolina. You will be working in a large development team in the process of building the firm's first online reservation system. your first assignment is to build the components that will check the age of the customer and then assign them a car. you will be building the business logic classes, so you do not have to worry about the forms of communicating with the user or data access classes to communicate with the database. To test your classes, you will use them in a test application. The requirements for your classes and the testing application are as follows: -You will design and build 2 components of the online reservation system(ORS). There will be one class to represent customers and another to represent vehicles. -Each class should have at least 2 members and 2 methods -The ORS will need to be able to check to make sure the customer in question is at least 25 years old. If…arrow_forward
- {In Java Programming Please} Create a class hierarchy that represents different types of employees in a company. Start with a class called Employee that has two methods: calculateSalary() and displayDetails(). Then, create two subclasses of Employee: Manager and Engineer. Override the calculateSalary() method in both subclasses to calculate the salary based on their job role and experience. Override the displayDetails() method in both subclasses to print out a message indicating the details of the employee. Next, create another class called Salesperson that extends Employee and has a method called calculateCommission(). Create two subclasses of Salesperson: SeniorSalesperson and JuniorSalesperson. Override the calculateCommission() method in both subclasses to calculate the commission based on their sales performance. Override the displayDetails() method in both subclasses to print out a message indicating the details of the salesperson. In the main method, create objects of each class…arrow_forward// The language is java please do question #9arrow_forwardIn the class diagram below we have a parking charge class for an object-oriented parking system that is to be designed using java. Briefly explain any implementation decisions and the reasoning behind those without writing the complete code. N.B explain how the implementation will proceed instead of writing codearrow_forward
- Farrell, Joyce. Microsoft Visual C#: An Introduction to Object-Oriented Programming (p. 479). Kindle Edition. 9. Write a program named SalespersonDemo that instantiates objects using classes named RealEstateSalesperson and GirlScout. Demonstrate that each object can use a SalesSpeech() method appropriately. Also, use a MakeSale() method two or three times with each object, and display the final contents of each object’s data fields. First, create an abstract class named Salesperson. Fields include first and last names; the Salesperson constructor requires both these values. Include properties for the fields. Include a method that returns a string that holds the Salesperson’s full name—the first and last names separated by a space. Then perform the following tasks: • Create two child classes of Salesperson: RealEstateSalesperson and GirlScout. The RealEstateSalesperson class contains fields for total value sold in dollars and total commission earned (both of which are…arrow_forwardPYTHONCreate a class named Circle that will inherit properties and methods from a class namedEllipse, and you do not want to add any other properties or methods to the class. 2. Explain the purpose of inheritance. And then explain why the Circle class can inheritfrom the Ellipse class.Write Python code to define the Ellipse class with reasonablenumber of properties and methods.arrow_forwardA complex number has two parts: real value and imaginary value. In mathematics, we learnt how to add and multiply two complex numbers. Write a class Complex which will have two fields for real and imaginary values. The constructor will set the values in these fields (there could be multiple overloaded constructors). The class should also have method like this: Complex add (Complex c) This add() method takes a complex object and return a new complex object after adding the complex number c with itself(Use general complex number addition rule).arrow_forward
- Object Oriented Programming: 213COMP, 214COMP (Feb-2022) Assignment- I [10 marks] Academic honesty: O Only pdf file accepted & student ID, will be your upload file. O Student who submit copied work will obtain a mark of zero. O Late work or attach file by email message not allowed. Q1: Write the signature for a method that has one parameter of type String, and does not return a value. Q2: Write the signature for a method that has two parameters, both of type Student, and returns an int value. Q3: Write the constructor's headers of the followings? new Student (202101156, “Ahmed"); new Address(51, "jazan university","CS&IT" ); new Grade(true, 505235600, 4.5); Q4: a) Write a class Student that define the following information: name, stid , age. b) Explain a mutators (setters) and accessors(getters) methods for each attributes(fields). c) Add three constructors: • with empty constructor. one parameter constructor (name of student) two parameters constructor (name and stid) d) Create two…arrow_forwardHello, I have written my random bear generator but for some reason the code isn't running in my command terminal. Please have a look at it and let me know what I am missing. I followed my teachers instructors and lectures, but it doesn't run on another compiler either. class Bear { constructor(type, color, weight, favoriteFood) { this.type = type; this.color = color; this.weight = weight; this.favoriteFood = favoriteFood; if (this.weight === 700 || this.type === "Little Bear" || this.type === "Baby Bear") { this.isAggressive = false; } else if (this.weight === 1200 || this.type === "Papa Bear" || this.type === "Black Bear") { this.isAggressive = true; } else { this.isAggressive = Math.random() < 0.5; } if (this.type === "Winnie the Pooh Bear" && this.favoriteFood !== "Honey") { throw new Error("Winnie the Pooh Bear must have Honey as his favorite food."); } }} class BearGenerator { get types() { return [ "Winnie…arrow_forwardUse the class diagram below to create a Parking Charge class for an object-oriented parking system. Write your code using java. Classes should contain properties and method implementations. N.B. Try to make your code readable.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
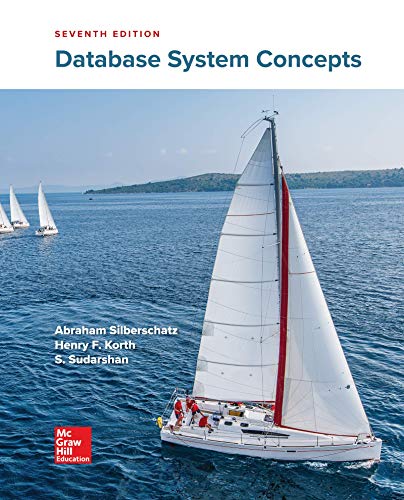
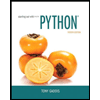
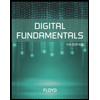
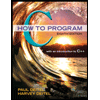
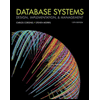
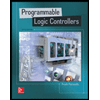