I have a question for the following program: #include #include using namespace std; int rolldice(){ int r1, r2, s = 0; r1 = rand() % 6 + 1; cout << "After rolling: " << r1 << endl; r2 = rand() % 6 + 1; cout << "After rolling: " << r2 << endl; s = r1 + r2; if(r1 == r2){ r1 = rand() % 6 + 1; cout << "After rolling: " << r1 << endl; r2 = rand() % 6 + 1; cout << "After rolling: " << r2 << endl; s += r1 + r2; if(r1 == r2) s = 1; } return s; } int findfirst(int pos[]){ int i, j = 0, m = pos[0]; for(i = 1; i < 4; i++){ if(m < pos[i]){ m = pos[i]; j = i; } } return j; } int findlast(int pos[]){ int i, j = 0, m = pos[0]; for(i = 1; i < 4; i++){ if(m > pos[i]){ m = pos[i]; j = i; } } return j; } void calposition(int pos[], int sum[]){ int i, p, temp, j; for(i = 0; i < 4; i++){ if(sum[i] == 1 || sum[i] == 12) pos[i] = 0; else if(sum[i] == 4 && pos[i] > 1) pos[i] -= 1; else if(sum[i] == 7){ p = findfirst(pos); if(pos[p] != pos[i]){ temp = pos[i]; pos[i] = pos[p]; pos[p] = temp; } } else if(sum[i] == 11){ p = findlast(pos); if(pos[p] != pos[i]){ temp = pos[i]; pos[i] = pos[p]; pos[p] = temp; } } else{ if((pos[i] + sum[i]) <= 50) pos[i] += sum[i]; } for(j = 0; j < 4; j++){ if(i != j){ if(pos[i] == pos[j]) pos[j] = 0; } } } } void display(int pos[]){ int i,j, k = 1, m; for(i = 1; i <= 51; i++) cout << "_"; cout << endl; for(i = 1; i <= 5; i++){ for(j = 1; j <= 10; j++){ if(k == pos[0]) cout << "|_P" << 1 << "_"; else if(k == pos[1]) cout << "|_P" << 2 << "_"; else if(k == pos[2]) cout << "|_P" << 3 << "_"; else if(k == pos[3]) cout << "|_P" << 4 << "_"; else cout << "|____"; k++; } cout << "|" << endl; } } int findwinner(int pos[]){ int i; for(i = 0; i < 4; i++){ if(pos[i] == 50){ break; } } if(i == 4) return 0; else return (i + 1); } int main() { int pos[4]; int sum[4]; int i, temp, winner; char choice = 'y'; cout << "Lets play, sorry!" << endl; while(choice == 'y' || choice == 'Y'){ pos[0] = pos[1] = pos[2] = pos[3] = 0; while(true){ for(i = 0; i < 4; i++){ cout << "Player " << i + 1 << " is rollong." << endl; sum[i] = rolldice(); } calposition(pos, sum); display(pos); winner = findwinner(pos); if(winner > 0){ cout << "Player " << winner << " is winner!!" << endl; break; } } cout << "Wanna play again? (Y/N)" << endl; cin >> choice; } return 0; } How did it display the player's position after every roll?
I have a question for the following program:
#include <iostream>
#include <stdlib.h>
using namespace std;
int rolldice(){
int r1, r2, s = 0;
r1 = rand() % 6 + 1;
cout << "After rolling: " << r1 << endl;
r2 = rand() % 6 + 1;
cout << "After rolling: " << r2 << endl;
s = r1 + r2;
if(r1 == r2){
r1 = rand() % 6 + 1;
cout << "After rolling: " << r1 << endl;
r2 = rand() % 6 + 1;
cout << "After rolling: " << r2 << endl;
s += r1 + r2;
if(r1 == r2)
s = 1;
}
return s;
}
int findfirst(int pos[]){
int i, j = 0, m = pos[0];
for(i = 1; i < 4; i++){
if(m < pos[i]){
m = pos[i];
j = i;
}
}
return j;
}
int findlast(int pos[]){
int i, j = 0, m = pos[0];
for(i = 1; i < 4; i++){
if(m > pos[i]){
m = pos[i];
j = i;
}
}
return j;
}
void calposition(int pos[], int sum[]){
int i, p, temp, j;
for(i = 0; i < 4; i++){
if(sum[i] == 1 || sum[i] == 12)
pos[i] = 0;
else if(sum[i] == 4 && pos[i] > 1)
pos[i] -= 1;
else if(sum[i] == 7){
p = findfirst(pos);
if(pos[p] != pos[i]){
temp = pos[i];
pos[i] = pos[p];
pos[p] = temp;
}
}
else if(sum[i] == 11){
p = findlast(pos);
if(pos[p] != pos[i]){
temp = pos[i];
pos[i] = pos[p];
pos[p] = temp;
}
}
else{
if((pos[i] + sum[i]) <= 50)
pos[i] += sum[i];
}
for(j = 0; j < 4; j++){
if(i != j){
if(pos[i] == pos[j])
pos[j] = 0;
}
}
}
}
void display(int pos[]){
int i,j, k = 1, m;
for(i = 1; i <= 51; i++)
cout << "_";
cout << endl;
for(i = 1; i <= 5; i++){
for(j = 1; j <= 10; j++){
if(k == pos[0])
cout << "|_P" << 1 << "_";
else if(k == pos[1])
cout << "|_P" << 2 << "_";
else if(k == pos[2])
cout << "|_P" << 3 << "_";
else if(k == pos[3])
cout << "|_P" << 4 << "_";
else
cout << "|____";
k++;
}
cout << "|" << endl;
}
}
int findwinner(int pos[]){
int i;
for(i = 0; i < 4; i++){
if(pos[i] == 50){
break;
}
}
if(i == 4)
return 0;
else
return (i + 1);
}
int main() {
int pos[4];
int sum[4];
int i, temp, winner;
char choice = 'y';
cout << "Lets play, sorry!" << endl;
while(choice == 'y' || choice == 'Y'){
pos[0] = pos[1] = pos[2] = pos[3] = 0;
while(true){
for(i = 0; i < 4; i++){
cout << "Player " << i + 1 << " is rollong." << endl;
sum[i] = rolldice();
}
calposition(pos, sum);
display(pos);
winner = findwinner(pos);
if(winner > 0){
cout << "Player " << winner << " is winner!!" << endl;
break;
}
}
cout << "Wanna play again? (Y/N)" << endl;
cin >> choice;
}
return 0;
}
How did it display the player's position after every roll?

Step by step
Solved in 3 steps

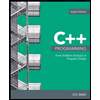
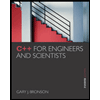
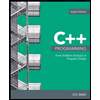
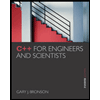