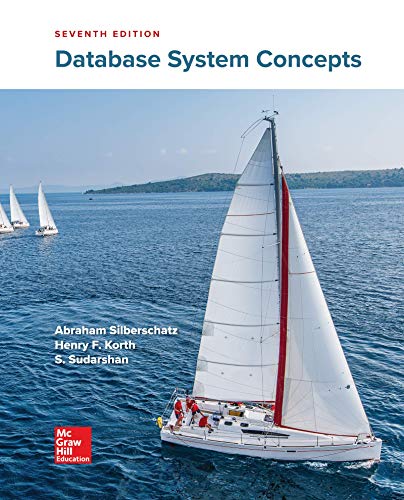
I need help passing the unit test.
My code(python):
n = int(input())
user_values =[]
def get_user_values():
for i in range(n):
num = int(input())
user_values.append(num)
upper_threshold=user_values[-1]
return user_values, upper_threshold
def output_ints_less_than_or_equal_to_threshold(user_values, upper_threshold):
user_values = [i for i in user_values if i <= upper_threshold]
print(*user_values, sep = "\n")
if __name__ == '__main__':
user_values, upper_threshold = get_user_values()
output_ints_less_than_or_equal_to_threshold(user_values, upper_threshold)
Write a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, and output all integers less than or equal to that value.
Ex: If the input is:
5 50 60 140 200 75 100
the output is:
50 60 75
The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75.
Such functionality is common on sites like Amazon, where a user can filter results.
Your code must define and call the following two functions:
def get_user_values()
def output_ints_less_than_or_equal_to_threshold(user_values, upper_threshold)
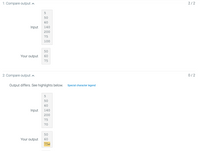
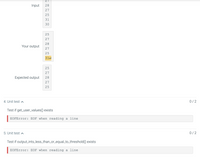

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Explain each line in main and explain why the output looks how it looks. #include <iostream> using namespace std; class A { public: A() { n=0; } A(int x) { n=x; } void f() { n++; } void g() { f(); n=n*2; f(); } int k() { return n; } void p() { cout<<n<<endl; } private: int n; }; void main() { A a; A b(2); A c=b; A d=A(3); a.f(); b.g(); c.f(); d.g(); d.p(); A e(a.k() + b.k() + c.k() + d.k()); e.p(); }arrow_forwardWrite a program that takes in four positive integers and outputs the number of odd numbers. (Hint: use the modulo operator to determine if a number is odd) Ex: If the input is: 1 2 3 4 the output is: 2 Below is my code so far and I'm getting back unexpected EOF while parsing. I can't figure out what I'm doing wrong. odd_number=[]number_1=int(input())number_2=int(input())number_3=int(input())number_4=int(input())if number_1%2!=0: odd_number.append(number_1)else: passif number_2%2!=0: odd_number.append(number_2)else: passif number_3%2!=0: odd_number.append(number_3)else: passif number_4%2!=0: odd_number.append(number_4)else: passprint(len(odd_number)arrow_forwarddef hailstone(n): h.append(int(n)) if n == 1: return h while n != 1: if n % 2 == 0: return hailstone(int(n/2)) else: return hailstone(int(3*n + 1))y=int(input("N: "))if y <= 0: print("N must be positive!")else: print("Hailstone sequences for 1 to " + str(y) + ":") m=0; i=1 for x in range(1, y+1): n = i h = [] h = hailstone(n) print(h) s=len(h) if s > m: m=s flag=n i=i+1 print("First longest sequence found in n =", + flag ) If i don't one the output have the square braket? How can I fix this code? Can you pleasw help me?arrow_forward
- in python3. can you help with turning output into the desired output of dictionaries. Same as the picture down below.arrow_forwardExplain what the code does step by step.arrow_forwardWhat is the value of the variable ss after the following code is executed? int x[ 7 ] = (5,10,3,0,-10, 4); int ss=0; for (int i=1;i<=6; i++) { ss+=x[i]; cout<arrow_forward
- def hailstone(n): h.append(int(n)) if n == 1: return h while n != 1: if n % 2 == 0: return hailstone(int(n/2)) else: return hailstone(int(3*n + 1))y=int(input("N: "))if y <= 0: print("N must be positive!")else: print("Hailstone sequences for 1 to " + str(y) + ": ") m=0; i=1 for x in range(1, y+1): n = i h = [] h = hailstone(n) print(*h) print(",") s=len(h) if s > m: m=s flag=n i=i+1 print("First longest sequence found in n =", + flag ) How can I fix this code if I want a comma between each number, not each line?arrow_forwardWhat is the value of the variable ss after the following code is executed? int x[ 7 ] = (5,10,3,0,-10, 4); int ss=0; for (int i=1;i<=6; i++) { ss+=x[i]; cout<arrow_forwardIn Java: Forms often allow a user to enter an integer. Write a program that takes in a string representing an integer as input, and outputs yes if every character is a digit 0-9. Ex: If the input is: 1995 the output is: yes Ex: If the input is: 42,000 or 1995! the output is: no Hint: Use a loop and the Character.isDigit() function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
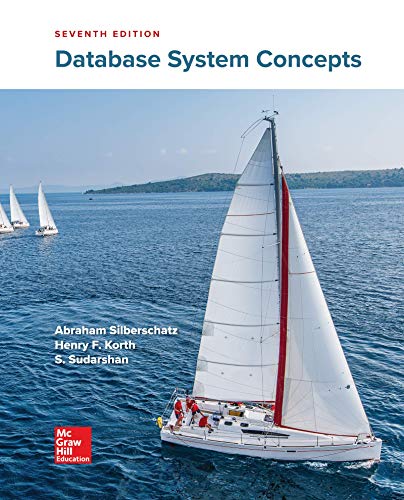
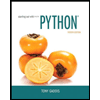
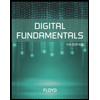
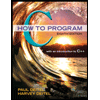
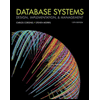
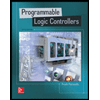