I need help with coding in C++ please: Write a program (in main.cpp) to do the following: a. Build a binary search tree T1. b. Do a postorder traversal of T1 and, while doing the postorder traversal, insert the nodes into a second binary search tree T2 . c. Do a preorder traversal of T2 and, while doing the preorder traversal, insert the node into a third binary search tree T3. d. Do an inorder traversal of T3. e. Output the heights and the number of leaves in each of the three binary search trees. The program should accept input and produce output similar to the example below: Enter numbers ending with -999: 10 5 7 -999 tree1 nodes in postorder: 7 5 10 tree2 nodes in preorder: 7 5 10 tree3 nodes in inorder: 5 7 10 tree1 height: 3 tree1 leaves: 1 tree2 height: 2 tree2 leaves: 2 tree3 height: 2 tree3 leaves: 2 The program has 3 tabs: main.cpp , binarysearchTree.h , and binaryTree.h
I need help with coding in C++ please:
Write a program (in main.cpp) to do the following:
a. Build a binary search tree T1.
b. Do a postorder traversal of T1 and, while doing the postorder traversal, insert the nodes into a second binary search tree T2 .
c. Do a preorder traversal of T2 and, while doing the preorder traversal, insert the node into a third binary search tree T3.
d. Do an inorder traversal of T3.
e. Output the heights and the number of leaves in each of the three binary search trees.
The program should accept input and produce output similar to the example below:
Enter numbers ending with -999: 10 5 7 -999 tree1 nodes in postorder: 7 5 10 tree2 nodes in preorder: 7 5 10 tree3 nodes in inorder: 5 7 10 tree1 height: 3 tree1 leaves: 1 tree2 height: 2 tree2 leaves: 2 tree3 height: 2 tree3 leaves: 2
The program has 3 tabs: main.cpp , binarysearchTree.h , and binaryTree.h

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

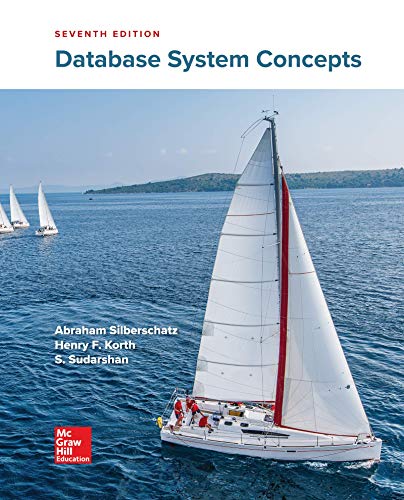
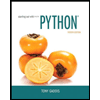
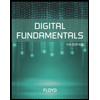
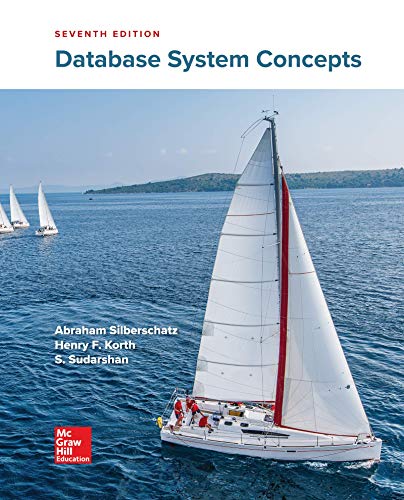
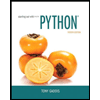
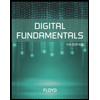
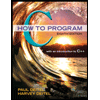
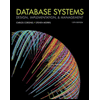
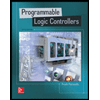