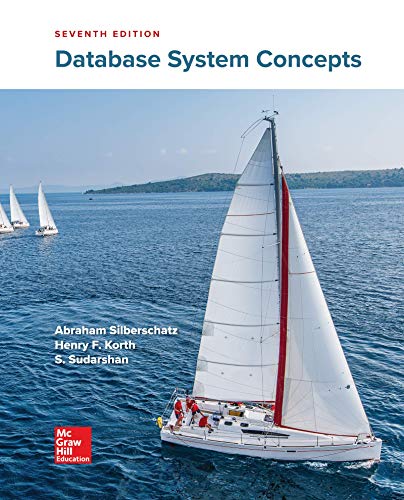
I need help with programming in MATLAB. The following code transforms cartesian coordinates to the kepler elements. Can you give me the code for transforming kepler orbital elements to cartesian coordinates. The following code gives the 6 kepler elements. Transform those elements into cartesian coordinate that match the values under the Example Usage part of the code.
% Example usage:
x = 1000;
y = 2000;
z = 3000;
vx = 4;
vy = -3;
vz = 2;
[a, ecc, inc, raan, argp, f] = cart2orb(x, y, z, vx, vy, vz);
% Display the results
disp(['Semi-Major Axis (a): ', num2str(a), ' km']);
disp(['Eccentricity (ecc): ', num2str(ecc)]);
disp(['Inclination (inc): ', num2str(inc), ' degrees']);
disp(['Right Ascension of Ascending Node (raan): ', num2str(raan), ' degrees']);
disp(['Argument of Perigee (argp): ', num2str(argp), ' degrees']);
disp(['True Anomaly (f): ', num2str(f), ' degrees']);
function [a, e, inc, raan, argp, f] = cart2orb(x, y, z, vx, vy, vz)
% Gravitational constant for Earth (μ⊕)
mu = 398600.4418; % km^3/s^2
% Calculate position and velocity
r = [x; y; z]; % Position vector
v = [vx; vy; vz]; % Velocity vector
% Calculate orbital parameters
h = cross(r, v); % Specific angular momentum vector
n = cross([0; 0; 1], h); % Nodal vector
% Eccentricity (ecc)
e_vec = (cross(v,h)/mu) - (r/norm(r));
%e_vec = ((norm(v)^2 - mu/norm(r)) * r - dot(r, v) * v) / mu;
e = norm(e_vec);
% Semi-major axis (a)
a = (dot(h,h)/mu) / (1-e^2);
%a = 1 / (2/norm(r) - norm(v)^2/mu_earth);
% Inclination (inc)
inc = acosd(h(3) / norm(h));
% Right Ascension of Ascending Node (raan)
raan = atan2d(n(2), n(1));
raan = mod(raan + 360, 360); % Ensure raan is in the range [0, 360)
% Argument of Perigee (argp)
argp = atan2d(dot(n, cross(e_vec, h)), dot(n, e_vec));
argp = mod(argp + 360, 360); % Ensure argp is in the range [0, 360)
% True Anomaly (f)
f = atan2d(dot(e_vec, cross(h, r)), dot(e_vec, r));
f = mod(f + 360, 360); % Ensure f is in the range [0, 360)
end

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Please help solve the python problemarrow_forwardIn this lab, you will write a MIPS program to convert Fahrenheit temperature to Celsius. A basic conversion program was taught in the class. You should utilize that code from the lecture slides. Your program will take the Fahrenheit temperature as user input (single precision floating point). The temperature conversion code will be a leaf procedure that will be called from the main program after the user input. The procedure also will print the single precision floating value of the Celsius temperature. After the program returns to the main program, the user will be asked if they want another conversion. A character will be taken as input to indicate the user’s preference. If the input character is ‘y’, the program will continue from the beginning by taking another input and calling the conversion procedure. Any other input character will make the program exit. You may need to learn a few syscall parameters that deal with character and single precision floating point numbers. You will…arrow_forwardPlease code in OOP C++, show example output to see if no errorsCrossword Puzzles An unlabeled crossword is given to you as an N by M grid (3 <= N <= 50, 3 <= M <= 50). Some cells will be clear (typically colored white) and some cells will be blocked (typically colored black). Given this layout, clue numbering is a simple process which follows two logical Steps: Step 1: We determine if a each cell begins a horizontal or vertical clue. If a cell begins a horizontal clue, it must be clear, its neighboring cell to the left must be blocked or outside the crossword grid, and the two cells on its right must be clear (that is, a horizontal clue can only represent a word of 3 or more characters). The rules for a cell beginning a vertical clue are analogous: the cell above must be blocked or outside the grid, and the two cells below must be clear. Step 2: We assign a number to each cell that begins a clue. Cells are assigned numbers sequentially starting with 1 in the same order that…arrow_forward
- Write a program named stars.py that has two functions for generating star polygons. One function should be implemented using an iteration (a for loop), and another function should be a recursion. It is optional to fill in your shapes. The functions for generating stars should be named star (size, n, d=2) and star_recursive (size, n, level, d=2), where size is the size of the polygon side (edge), n is the number of sides (or angles), d is a density or winding number that should be set to default value 2, and level is the level of recursion initially equal to n.arrow_forwardWrite a Better version for this code using any preferred programming language. You should follow the high-quality code concepts and practices to achieve the high-quality version.arrow_forwardmake mathlab codearrow_forward
- The following MATLAB code plots the location of a building and the orbit of a satellite. How much time, in hours, does the building have coverage from this satellite every day. % At the initial time, the true anomaly is equal to zero,% and the Greenwich meridian points to the Aries point. % Initial conditionsomega_earth = rad2deg(7.2921151467e-5); % deg/ssemi_major_axis = 3096.7363; % kmeccentricity = 0.74;inclination = 63.4349; % degreesRAAN = -86.915798; % degreesargument_of_perigee = 270; % degreesn_points = 100; % number of pointscone_angle = 10; % degrees % Building coordinatesbuilding_latitude = 40.43094; % degreesbuilding_longitude = -86.915798; % degrees % Calculate time vector over one orbital periodorbital_period = 360 / omega_earth;time_vector = linspace(0, orbital_period, n_points); % Preallocate arrays for orbital elementstrue_anomaly = zeros(1, n_points);longitude = zeros(1, n_points);latitude = zeros(1, n_points); % Calculate orbital elements at each time stepfor i =…arrow_forwardFunction scale multiples its first argument (a real number) by 10 raised to the power indicated by its second argument (an integer). For example, the function call scale (2.5, 2) double scale (double x, int n) double scale_factor; scale factor - pow (10, n); return x*scale_factor; Returns the value 250.0 (2.5 x 102). The function call scale (2.5, -2) Return the value 0.025 (2.5 x 10²) Write only the main function, create and initialize a double array and then call the function scale for each elements of the array. At the end, the contents of the array must consist of scaled values. Create and use a separate integer array as the powers of each corresponding value. Eg. If double array has the following contents: 1.0 2.0 3.0 4.0 and the Integer array of powers have the following: 4 3. 2 -2, After function calls, the new contents of the double array must be updated as in seen in the following: 1.0x104 2.0x10³ 3.0x10² 4.0x10²arrow_forwardCan you help me write the code for this MATLAB assignment?arrow_forward
- The area of a triangle is area = ½ base * height. Write MATLAB code that will find the area of a group of triangles whose base varies from 0 to 10 m, and whose height varies from 2 to 6 m. Both dimensions should be in increments of 1. Your answer should be a matrix of area values. Include your answers in comments using %.arrow_forwardI'm trying to write a program in java that takes user input for the size of the sun in inches and outputs a table of solar system values based on the size of the sun given in inches. Camilla was asked by her niece, to help her with her project for the 7th grade Science Fair. She was overjoyed to have been asked to help. Her niece wanted to build a scale model of the solar system. Her niece, remembers having received a planisphere in 4th grade science class. But, she had lost it. But, she remembered that there were instructions on the planisphere for how to build a scale model of the solar system based on the Sun being the same size as the star circle. Her niece had always wanted to build that model and she thought that this would be the perfect Science Fair project. Camilla remembers having seen that planisphere. She and her niece guessed that the star circle in the planisphere was about 8 inches in diameter. Camilla was excited because she had always been interested in…arrow_forwardSo long as ptr is a reference to an integer, what happens if we add 4 to it?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
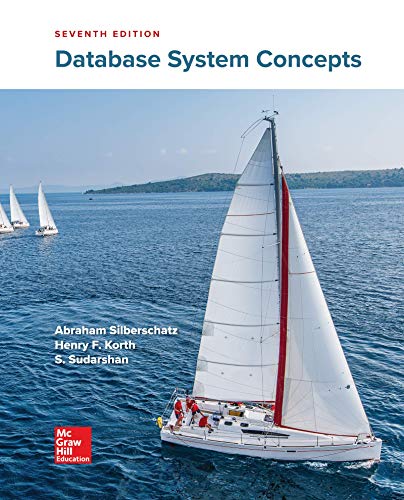
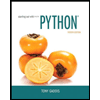
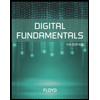
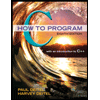
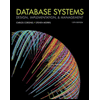
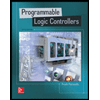