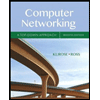
I need some help making this code more simple.
2)
tokenList = "{}()[]"
initialize stack to empty
Get file name from user: fileName
Open the file (fileName)
read line from fileName into strLine
while not EOF (end of file)
find tokenList items in strLine
for each token in strLine
if token = "[" then
push "]" into stack
else if token = "(" then
push ")" into stack
else if token = "{" then
push "}" into stack
else if token = "]" OR token = ")" OR token = "}" then
pop stack into poppedToken
if poppedToken not equal token
print "Incorrect Grouping Pairs"
exit
end if
end if
next token
end while
if stack is empty
print "Correct Grouping Pairs"
else
print "Incorrect Grouping Pairs"
end if
![6) Let's pop one more time
poppeditem = "]"
Design Strategy
We have 3 types of tokens we're looking for:
1) Parenthesis ( )
2) Brackets []
3) Curly Brackets {}
There's an open and closed version of each token.
Look at this line: x = [a, b(1,2,3], c] This is an unmatched pair, because the closed bracket after the
number 3, should have been a closed parenthesis, to complete the open parenthesis before the number
1. Here's what the correct version looks like: x = [a, b(1,2,3), c]
A stack is a perfect data structure to keep track of the tokens in the order they were encountered. For
the tokens to be matched, there always has to be a closed token the directly precedes the same version
of an open token. Anytime we come across a closed token we can just pop off the latest item in a stack
to see if it matches.
Algorithm
Let's read a file a line at a time and scan for tokens on each line. As we encounter an open token, push
it into the stack. When we encounter a closed token we pop an item from the stack and see if it
matches the open token. If it doesn't we print a message saying "Unmatched Pair". When we finish
reading the file we check to see if the queue is empty. If it is "Success" or else it's an unmatched pair.
You can use the partial algorithm below and fill in the rest of it if you'd like. Iľ'm not expecting you to
provide details for managing push and pop functions. You can assume that there's a pop and push
function built in that you can utilize.](https://content.bartleby.com/qna-images/question/c43e03e1-b353-4d50-a726-bab1a8232fef/b40ea67a-c4dc-424b-8dc5-1bde55af32cc/02mpv9_thumbnail.png)
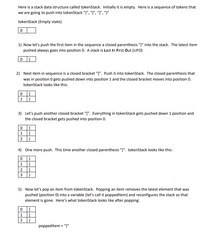

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- What will be the stack content after following operations: Push 5 Push 8 Pop Push 1 Push 12 Poparrow_forwardC++ Program This assignment consists of 2 parts. 1. Append Process: Create a Linked List that will store integers. Using the provided getData() method (See below), append 20 numbers to the Linked List. After loaded, display the data. Start with the Head of the list. Prompt for user to proceed. 2. Insert Process: Delete the contents of the Linked List in part 1. Using the provided getData() method, insert 20 numbers into the list and place them in the list in numerical order (1..X). Do not allow duplicates to be in the list. Hint: Do not allow a duplicate to count as a member of the 20. After loaded, display the data per line in order, starting with the Head of the list. Display the content with a leading increasing number 1- 20. i.e. 11 13 14 20 The result should be a naturally sorted. Do not use the STL list container for this exercise. Use the features described in section - Linked List Operations. Using a Class to manage the linked list as shown in section is…arrow_forwardWrite a python hangman program Modify your program so that the following functionality is added:1. Instead of selecting the word from a hard coded list, incorporate problem2 so that thelist is read from a file, then a word is randomly selected from this list.2. when the game ends, the following information should be outputted to a new file:● the word● the state of the hangman● if the user won or lostExample outputs:quokkaO\||You won!kangarooO\|/|/ \You lostarrow_forward
- This code gets an error for going out of range in the last for loop. What can I do to fix this? # Lucas Conklin# 5772707import csvimport statistics def readCSVIntoDictionary(f_name): data = [] with open(f_name) as f: reader = csv.reader(f) for row in reader: if not data: for index in range(len(row)): data.append([]) for index in range(len(row)): data[index].append(float(row[index])) f.close() return data features = readCSVIntoDictionary("C:\\Users\\lucas\\Downloads\\pima.csv")print(features) def find_median_and_SD(data, feature): med = statistics.median(data[feature]) rounded_med = round(med, 4) st_dev = statistics.stdev(data[feature]) rounded_st_dev = round(st_dev, 5) return rounded_med, rounded_st_dev for i in range(0, len(features)): (median, st_dev) = find_median_and_SD(features, i) print(f'Feature {i} Median: {median} Standard Deviation: {st_dev}')…arrow_forwardGiven the following linked lists: Trace the following codeon these two linked lists and show what will be printed.arrow_forwardclass SLNode: """ Singly Linked List Node class DO NOT CHANGE THIS CLASS IN ANY WAY """ def __init__(self, value: object, next=None) -> None: self.value = value self.next = next from SLNode import *class SLLException(Exception): """ Custom exception class to be used by Singly Linked List DO NOT CHANGE THIS CLASS IN ANY WAY """ passclass LinkedList: def __init__(self, start_list=None) -> None: """ Initialize new linked list DO NOT CHANGE THIS METHOD IN ANY WAY """ self._head = SLNode(None) # populate SLL with initial values (if provided) # before using this feature, implement insert_back() method if start_list is not None: for value in start_list: self.insert_back(value) def __str__(self) -> str: """ Return content of singly linked list in human-readable form DO NOT CHANGE THIS METHOD IN ANY WAY """ out =…arrow_forward
- starter code //Provided imports, feel free to use these if neededimport java.util.Collections;import java.util.ArrayList; /** * TODO: add class header */public class Sorts { /** * this helper finds the appropriate number of buckets you should allocate * based on the range of the values in the input list * @param list the input list to bucket sort * @return number of buckets */ private static int assignNumBuckets(ArrayList<Integer> list) { Integer max = Collections.max(list); Integer min = Collections.min(list); return (int) Math.sqrt(max - min) + 1; } /** * this helper finds the appropriate bucket index that a data should be * placed in * @param data a particular data from the input list if you are using * loop iteration * @param numBuckets number of buckets * @param listMin the smallest element of the input list * @return the index of the bucket for which the particular data should…arrow_forwardWrite a recursive function to recursively print the file list returned by os.listdir().arrow_forward**Cenaage Python** Question: A sequential search of a sorted list can halt when the target is less than a given element in the list. Modify the program to stop when the target becomes less than the current value being compared. In a sorted list, this would indicate that the target is not in the list and searching the remaining values is unnecessary. CODE: def sequentialSearch(target, lyst): """Returns the position of the target item if found, or -1 otherwise. The lyst is assumed to be sorted in ascending order.""" position = 0 while position < len(lyst): if target == lyst[position]: return position position += 1 return -1 def main(): """Tests with three lists.""" print(sequentialSearch(3, [0, 1, 2, 3, 4])) print(sequentialSearch(3, [0, 1, 2])) # Should stop at second position. print(sequentialSearch(3, [0, 4, 5, 6])) if __name__ == "__main__": main()arrow_forward
- What is the operation that adds items to a stack? Get Set Pop Pusharrow_forwardWhich variable is appropriate to track the insertion position to a stack?arrow_forwardAll files are included below, seperated by the dashes. "----" //driver.cpp #include <iostream> #include <string> #include "stackLL.h" #include "queueLL.h" #include "priorityQueueLL.h" using namespace std; int main() { /////////////Test code for stack /////////////// stackLLstk; stk.push(5); stk.push(13); stk.push(7); stk.push(3); stk.push(2); stk.push(11); cout<<"Popping: "<<stk.pop() <<endl; cout<<"Popping: "<<stk.pop() <<endl; stk.push(17); stk.push(19); stk.push(23); while( ! stk.empty() ) { cout<<"Popping: "<<stk.pop() <<endl; } // output order: 11,2,23,19,17,3,7,13,5 stackLLstkx; stkx.push(5); stkx.push(10); stkx.push(15); stkx.push(20); stkx.push(25); stkx.push(30); stkx.insertAt(-100, 3); stkx.insertAt(-200, 7); stkx.insertAt(-300, 0); //output order: -300,30,25,20,-100,15,10,5,-200 while( ! stkx.empty() ) cout<<"Popping: "<<stkx.pop() <<endl; ///////////////////////////////////////…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
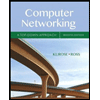
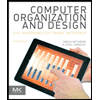
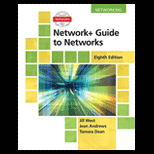
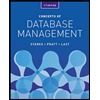
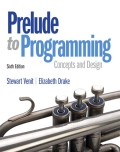
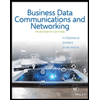