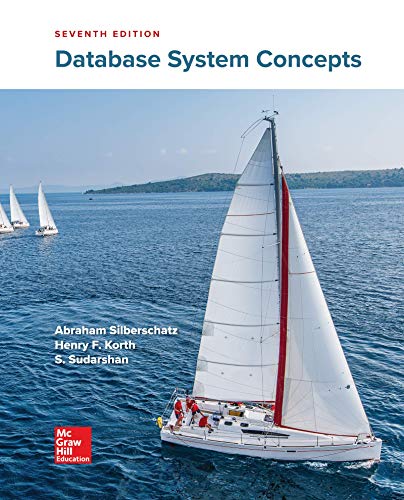
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Needed:
This method subtracts num2 from num1 (both represented as CharStacks), and saves the result on a stack.
// Do not create any arrays! Do not use any Java libraries to do the calculation.
// Doing so will result in points deduction.
public String subtractBigInteger(CharStack num1, CharStack num2)
{
CharStack stackResult = new CharStack(64); // stack used to store the result of the multiplication
char borrow = 0;
// TODO: complete this method
return stackResult.toString(); // return a string representation of the stack
}
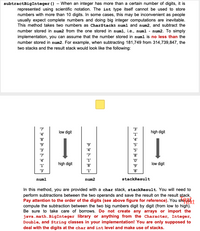
Transcribed Image Text:subtractBigInteger () – When an integer has more than a certain number of digits, it is
represented using scientific notation. The int type itself cannot be used to store
numbers with more than 10 digits. In some cases, this may be inconvenient as people
usually expect complete numbers and doing big integer computations are inevitable.
This method takes two numbers as Charstacks numl and num2, and subtract the
number stored in num2 from the one stored in numl, i.e., num1 - num2. To simply
implementation, you can assume that the number stored in numl is no less than the
number stored in num2. For example, when subtracting 181,749 from 314,739,847, the
two stacks and the result stack would look like the following:
'7'
'3'
low digit
high digit
'4'
'1'
'8'
'4'
'9'
'9'
'5'
'3'
'4'
'5'
יךי
'7'
'8'
'4'
'
'O'
'1'
high digit
'8'
'9'
low digit
'3'
'1'
'8'
numl
num2
stackResult
In this method, you are provided with a char stack, stackResult. You will need to
perform subtractions between the two operands and save the result on the result stack.
Pay attention to the order of the digits (see above figure for reference). You shoud t
compute the subtraction between the two big numbers digit by digit (from low to high).
Be sure to take care of borrows. Do not create any arrays or import the
java.math.BigInteger library or anything from the Character, Integer,
Double, and String classes in your implementation! You are only supposed to
deal with the digits at the char and int level and make use of stacks.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Creat a void fuction void myUpdate(string dictionary[], int wordCount, string oldWord, string newWord); Where it will replace the oldWord with the newWord, if the search is successfularrow_forwardThe following code prints out all permutations of the string generate. Insert the missing statement. public class PermutationGeneratorTester { public static void main(String[] args) { PermutationGenerator generator = new PermutationGenerator("generate"); ArrayList<String> permutations = generator.getPermutations(); for (String s : permutations) { ____________________ } } }arrow_forwardData Structure & Algorithm: Describe and show by fully java coded example how a hash table works. You should test this with various array sizes and run it several times and record the execution times. At least four array sizes are recommended. 10, 15, 20, 25. It is recommended you write the classes and then demonstrate/test them using another classarrow_forward
- maxLength Language/Type: Related Links: Java Set collections Set Write a method maxLength that accepts as a parameter a Set of strings, and that returns the length of the longest string in the set. If your method is passed an empty set, it should return 0. 1 9 10 Method: Write a Java method as described, not a complete program or class. N345678 2arrow_forward2 = 0 ) { numList [ i ] i } } } [1, 2, 3, 4, 5] [0, 2, 4, 6, 8] [2, 4, 6, 8, 10] [1, 3, 5, 7, 9]arrow_forwardWrite the following method that returns the sum of all numbersin an ArrayList:public static double sum(ArrayList<Double> list)Write a test program that prompts the user to enter five numbers, stores them inan array list, and displays their sum.arrow_forward
- Pythong help!arrow_forwardPROBLEM STATEMENT: In this problem you will need to insert 5 at the frontof the provided ArrayList and return the list. import java.util.ArrayList;public class InsertElementArrayList{public static ArrayList<Integer> solution(ArrayList<Integer> list){// ↓↓↓↓ your code goes here ↓↓↓↓return new ArrayList<>(); Can you help me with this question The Language is Javaarrow_forwardFor any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forward
- Java - This project will allow you to compare & contrast different 4 sorting techniques, the last of which will be up to you to select. You will implement the following: Bubble Sort (pair-wise) Bubble Sort (list-wise) [This is the selection sort] Merge Sort Your choice (candidates are the heap, quick, shell, cocktail, bucket, or radix sorts) [These will require independent research) General rules: Structures can be static or dynamic You are not allowed to use built in methods that are direct or indirect requirements for this project – You cannot use ANY built in sorting functions - I/O (System.in/out *) are ok. All compare/swap/move methods must be your own. (You can use string compares) Your program will be sorting names – you need at least 100 unique names (you can use the 50 given in project #3) – read them into the program in a random fashion (i.e. not in any kind of alpha order). *The more names you have, the easier it is to see trends in speed. All sorts will be from…arrow_forward6. the grade is under 20 which is outlier, remove it from the array list. 7. Print array list using System.out.println() 8. Use indexOf to print index of 80. 9. Use get function. 10. What is the difference between get and index of? 11. Print the values of the array list using Iterator class. 12.. Delete all the values of the array list using clear function. 13. Print all the values of the array after you execute clear using System.out.println(). what is the result of using clear function? 14. What is the shortcoming of using array List?arrow_forward#Steps: Create a Main class. Declare an ArrayList in the main() method and store the given values in it. Create an array called output of the size of the ArrayList. Iterate through for-loop to get each element from the array List and load it into the array using the get() method of the list. Print the array to see the loaded elements. import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { List<String> nameList = new ArrayList<String>(); nameList.add("Robert"); nameList.add("Samson"); nameList.add("Alex"); nameList.add("William"); System.out.println("Elements of List: " + nameList); // Create an array of the same size as nameList Hint: use size() method // Fetch the elements from the nameList and insert into newly created array // Hint: Use get() method to fetch the elements from…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
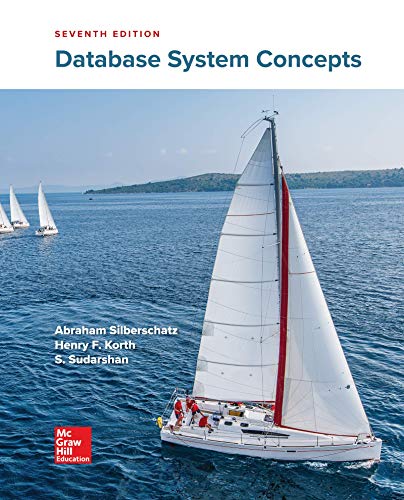
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
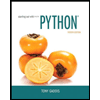
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
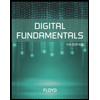
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
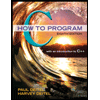
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
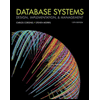
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
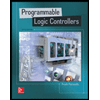
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education