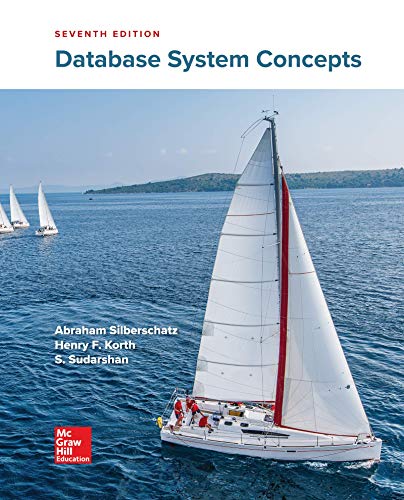
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
java
Create a static method that:
- is called repeatAll
- returns ArrayList of Booleans
- takes in a single parameter - an ArrayList of Booleans
This method should modify its ArrayList parameter by repeating its ArrayList values.
For example, if the parameter is
(true, false, false)
The modified ArrayList should be
(true, false, false, true, false, false)
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int size = in.nextInt();
ArrayList<Boolean> list = new ArrayList<>();
for(int i=0; i < size; i++) {
list.add(in.nextBoolean());
}
System.out.println(repeatAll(list));
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please use java! Thanks :Darrow_forward1. Write a program that calls a method that takes an integer array as a parameter (you can initialize an array in the main method) and find the minimum value from the list and returns it. Partial program is given below. public class Min{ public static void main(String[] args){ int arr= System.out.print(minimum(arr)); }arrow_forwardclass ArrayStackException extends Exception { ArrayStackException(String m) { super(m); }} public class ArrayStack { private int s[] = new int[100]; private int top=0; public void push(int data) throws ArrayStackException { if (top>=s.length) throw new ArrayStackException("Push overflow: " + data); s[top]=data; top++; } public int pop() throws ArrayStackException { if (top<=0) throw new ArrayStackException("Pop underflow: "); int value = s[top-1]; top--; return value; } public int peek() { int value = s[top-1]; return value; } public void clear() { top=0; } public int size() { return top; } public void display() { System.out.print( "size =" +top + ": "); for(int i =0; i<top;i++) { System.out.print( s[i] + " "); } System.out.println( ); } public static void…arrow_forward
- public static ArrayList findExactString(String s, ArrayList myArray) { return null; } // Use the above method, findExactString, as a helper method to implement addWithoutDuplication method. The addWithoutDuplication method has the following properties: 1- It takes two arguments, a string s and an ArrayList myArray of type string. 2- It returns an integer which represents the number of occurrences of the string s in myArray while attempting to add it. 3- If myArray is null, it returns -1 4- If adds the string s to the end of the ArrayList myArray if the string s is not already there. public static int addwithout Duplication(String s, ArrayList myArray) { return -1;arrow_forwardJava program pleasearrow_forwardjava This method gets an Arraylist of Integers and a number(Integer). It returns an Arraylist. It removes any instance of the given number from the Arraylist. Example: romoveInst([1,1,2,3,1,4],1) returns: [2,3,4] romoveInst([3,4,3,3],4) returns: [3,3,3] public static ArrayList<Integer> removeInst(ArrayList<Integer> r,Integer n) public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); int n = in.nextInt(); ArrayList<Integer> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.nextInt()); } System.out.println(removeInst(list, n)); } }arrow_forward
- Warmup Write a method called countSuit) that takes an array of Cards and a String suit as parameters and counts how many of the cards have the given suit. Complete the definition of the class Warmup and method definition countSuit shown below. public class Warmup { public static int countSuit(Card [] cards, String suit) { // fill in code here } } For example, if we ran the following code in the main method: Card[] cards = new Card[4]; cards [0] = new Card (4, "clubs"); cards [1] = new Card (12, "diamonds"); cards [2] = new Card (1, "hearts"); cards [3] new Card(10, "diamonds"); System.out.println(countSuit(cards, "diamonds")); the output printed would be 2.arrow_forwardimport java.util.Scanner;import java.util.ArrayList;import java.util.StringTokenizer; public class PlantArrayListExample { // TODO: Define a printArrayList method that prints an ArrayList of plant (or flower) objects public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String input; // TODO: Declare an ArrayList called myGarden that can hold object of type plant // TODO: Declare variables - plantName, plantCost, flowerName, flowerCost, colorOfFlowers, isAnnual input = scnr.next(); while(!input.equals("-1")){ // TODO: Check if input is a plant or flower // Store as a plant object or flower object // Add to the ArrayList myGarden input = scnr.next(); } // TODO: Call the method printArrayList to print myGarden }}arrow_forwardWrite a Java method that takes an array of type int and returns a boolean value. The method returns true if the number of positive elements in the parameter array is larger than the number of negative elements. The method returns false if any of the following conditions is satisfied: • The parameter array is of length of 0 or 1 • The number of positive elements of the parameter array is less than or equal to the number of negative elements • The number of positive elements or negative elements is equal to zero The method's header is as follows. public static boolean verify (int[] x) Sample run Result int[] x = (1, 2, 3}; verify(x); {5}; verify(x); int[] x = {1, 2, 3, -5, -1}; boolean b = verify(x); int[] x = new int[0]; verify(x); false boolean b = int[] x = boolean b = false true false boolean b = BIU A Paragraph +varrow_forward
- package edu.umsl.iterator;import java.util.ArrayList;import java.util.Arrays;import java.util.Collection;import java.util.Iterator;public class Main {public static void main(String[] args) {String[] cities = {"New York", "Atlanta", "Dallas", "Madison"};Collection<String> stringCollection = new ArrayList<>(Arrays.asList(cities));Iterator<String> iterator = stringCollection.iterator();while (iterator.hasNext()) {System.out.println(/* Fill in here */);}}} Rewrite the while loop to print out the collection using an iterator. Group of answer choices iterator.toString() iterator.getClass(java.lang.String) iterator.remove() iterator.next()arrow_forwardWrite a Java program to implement the following: Ask the user to enter the names of 3 Books and store them in an ArrayList called Book Remove the second book from the list Add the user to enter a new book name and store to be the first book of the list Ask the user to enter a book name, search for it in list, and print “exist” or “not exist” Print the book name at index 2 Print all list items using loop Print the size of the listarrow_forwardWrite an application that displays the strings in the provided array alphabetically in ascending order. import java.util.*; public class StringSort { publicstaticvoidmain(String[] args) { String[] values = {"mouse","dog","cat","horse","cow", "moose","tiger","lion","elephant","bird","hamster", "guinea pig","leopard","aardvark","hummingbird"}; }arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
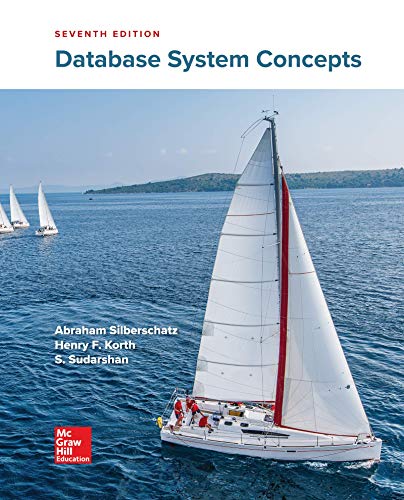
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
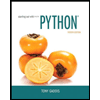
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
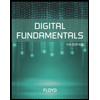
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
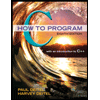
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
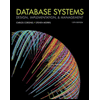
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
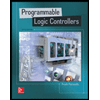
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education