In your workshop project create a new Java class (BasicArrayList) and convert the above code to work for ArrayLists. 1. You will need to import at least one package, but you do not need to import java.lang, which is the fundamental Java package (the classes you automatically get when you write Java programs). 2. Convert the first for-loop first. Don't forget you cannot use the ArrayList method to control the for-loop (so just use the number 4). 3. Convert the last for-loop next, to view the contents of your ArrayList. 4. Convert the middle for-loop last. You may want to convert the one line of array code to two lines of ArrayList code. 5. Finally, before the last for-loop, add a new element (0) to position 0 of the ArrayList. You should print the contents of the modified ArrayList. Here is a quick review of how array elements are modified and accessed: public class BasicArray{ 2. public static void main(String[] args) int] basic new int [4]; for (int i=0; i
In your workshop project create a new Java class (BasicArrayList) and convert the above code to work for ArrayLists. 1. You will need to import at least one package, but you do not need to import java.lang, which is the fundamental Java package (the classes you automatically get when you write Java programs). 2. Convert the first for-loop first. Don't forget you cannot use the ArrayList method to control the for-loop (so just use the number 4). 3. Convert the last for-loop next, to view the contents of your ArrayList. 4. Convert the middle for-loop last. You may want to convert the one line of array code to two lines of ArrayList code. 5. Finally, before the last for-loop, add a new element (0) to position 0 of the ArrayList. You should print the contents of the modified ArrayList. Here is a quick review of how array elements are modified and accessed: public class BasicArray{ 2. public static void main(String[] args) int] basic new int [4]; for (int i=0; i
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![In your workshop project create a new Java class (BasicArrayList) and convert the above code to work for ArrayLists.
1. You will need to import at least one package, but you do not need to import java.lang, which is the fundamental Java package (the classes you automatically get
when you write Java programs).
2. Convert the first for-loop first. Don't forget you cannot use the ArrayList method to control the for-loop (so just use the number 4).
3. Convert the last for-loop next, to view the contents of your ArrayList.
4. Convert the middle for-loop last. You may want to convert the one line of array code to two lines of ArrayList code.
5. Finally, before the last for-loop, add a new element (0) to position 0 of the ArrayList. You should print the contents of the modified ArrayList.
Here is a quick review of how array elements are modified and accessed:
1.
public class BasicArray{
2.
public static void main(String[] args)
int[] basic = new int[4];
for (int i=0; i<basic.length; i++) {
basic[i]=i+1;
5.
6.
}
7.
for (int i=0; i<basic.length; i++) {
basic[i] = basic[i]*5;
8.
9.
}
10.
for (int i=0; i<basic.length; i++) {
System.out.println(basic[i]);
11.
12.
}
13.
14. }
Because ArrayList is a Java class, we will need set and get methods to modify the ArrayList. The E
type refers to the base type of the ArrayList:
Return
Method
Description
(Parameters)
Value
Type
add(E element)
boolean Appends the specified element to the end of this list.
add(int index, E
element)
void
get(int index)
E
set(int index, E
element)
E
size()
int
Inserts the specified element at the specified position in this list.
Returns the element at the specified position in this list.
Replaces the element at the specified position in this list with the
specified element. Returns the element previously at the specified
position.
Returns the number of elements in this list.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6cf78908-a1f9-4330-9ece-f4021df10613%2F84388ddc-9fd8-49ee-8ac7-4fd7a0b77ae7%2Fnv7dkk_processed.png&w=3840&q=75)
Transcribed Image Text:In your workshop project create a new Java class (BasicArrayList) and convert the above code to work for ArrayLists.
1. You will need to import at least one package, but you do not need to import java.lang, which is the fundamental Java package (the classes you automatically get
when you write Java programs).
2. Convert the first for-loop first. Don't forget you cannot use the ArrayList method to control the for-loop (so just use the number 4).
3. Convert the last for-loop next, to view the contents of your ArrayList.
4. Convert the middle for-loop last. You may want to convert the one line of array code to two lines of ArrayList code.
5. Finally, before the last for-loop, add a new element (0) to position 0 of the ArrayList. You should print the contents of the modified ArrayList.
Here is a quick review of how array elements are modified and accessed:
1.
public class BasicArray{
2.
public static void main(String[] args)
int[] basic = new int[4];
for (int i=0; i<basic.length; i++) {
basic[i]=i+1;
5.
6.
}
7.
for (int i=0; i<basic.length; i++) {
basic[i] = basic[i]*5;
8.
9.
}
10.
for (int i=0; i<basic.length; i++) {
System.out.println(basic[i]);
11.
12.
}
13.
14. }
Because ArrayList is a Java class, we will need set and get methods to modify the ArrayList. The E
type refers to the base type of the ArrayList:
Return
Method
Description
(Parameters)
Value
Type
add(E element)
boolean Appends the specified element to the end of this list.
add(int index, E
element)
void
get(int index)
E
set(int index, E
element)
E
size()
int
Inserts the specified element at the specified position in this list.
Returns the element at the specified position in this list.
Replaces the element at the specified position in this list with the
specified element. Returns the element previously at the specified
position.
Returns the number of elements in this list.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
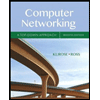
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
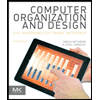
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
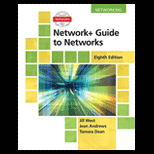
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
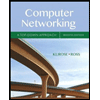
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
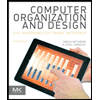
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
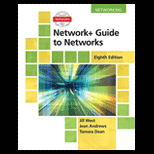
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
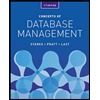
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
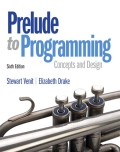
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
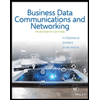
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY