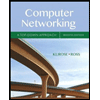
How do I make my queueLL.h into a template class and a decimation method?
////////////////////////////
#ifndef queueLL_H
#define queueLL_H
#include<iostream>
class queueLL
{
private:
//defining an inner node class
class node
{
public:
//has integer data
int data;
//reference to next node
node* next;
//constructor taking value for data field
node(int dat) {
data = dat;
next = NULL;
}
};
//references to front and rear nodes of the queue
node* front, * rear;
public:
//constructor to initialize empty queue
queueLL()
{
front = NULL;
rear = NULL;
}
~queueLL() // Deconstructor of queueLL to free space
{
//continuously dequeueing the queue until empty
while (!empty()) {
dequeue();
}
}
// Checks if the received queue is empty
bool empty() const
{
return front == NULL;
}
//Adds the enqueued item to back of queue
void enqueue(int x)
{
//if queue is empty, creating a new node and saving it as both front
//and rear
if (empty()) {
front = new node(x);
rear = front;
}
//otherwise appending new node as next of rear and updating rear
else {
rear->next = new node(x);
rear = rear->next;
}
}
//Removes and return first item from queue
int dequeue()
{
//taking a reference to front node
node* f = front;
//advancing front
front = front->next;
//if front is now NULL, setting rear to NULL too
if (front == NULL) {
rear = NULL;
}
//fetching data of f
int data = f->data;
//deleting f
delete f;
//returning removed data
return data;
}
};
#endif

Code:
#include <iostream>
using namespace std;
typedef int entrytype;
class node
{
public:
entrytype nodedata;
node *next;
node()
{
}
node(entrytype nodedata, node *next = nullptr)
{
this->nodedata = nodedata;
this->next = next;
}
};
class queue
{
private:
node *first;
node *last;
int Size;
public:
// constructor
queue()
{
first = last = nullptr;
Size = 0;
}
// destructor
~queue()
{
node *curr = first;
// iterate over the list and delete the nodes
while (curr != nullptr)
{
node *toDel = curr;
curr = curr->next;
delete toDel;
}
first = last = nullptr; // set the first AND LAST as null
Size = 0; // set the size to zero
}
// method to append a node to the end of the queue
bool append(entrytype value)
{
node *newNode = new node(value);
// return false in case of overflow
if (newNode == nullptr)
{
return false;
}
// if there is no node in the list
if (first == nullptr)
{
first = newNode; // make this the head node
last = first; // this is also the last node
}
// if there is atleast one node in the list
// add it to the end of the list and update the tail
else
{
last->next = newNode;
last = last->next;
}
// update the size
Size++;
return true;
}
// method to access the front element of the queue
bool front(entrytype &value)
{
// if queue is empty : return the underflow error
if (first == nullptr)
{
return false;
}
// else set the value as first element's value
value = first->nodedata;
return true;
}
// method to delete the first element of the queue
bool pop()
{
// if queue is empty : return underflow error code
if (first == nullptr)
{
return false;
}
// else remove the first element of the queue
node *toPop = first; // access the first element
// move first to next node
first = first->next;
// if first became null : it means there was a single node in the list
// so make last null as well.
if (!first)
last = nullptr;
delete toPop; // delete the first node
// update the Size
Size--;
return true; // return true if operation was successful
}
// method to get the current size of the queue
int size()
{
return Size;
}
// method to check if given element exists in the queue
bool find(entrytype target)
{
for (node *curr = first; curr != nullptr; curr = curr->next)
{
if (curr->nodedata == target) // if value is found return true
return true;
}
return false; // return false
}
};
int main()
{
queue *q = new queue; // create a new queue
// add the even elements to the queue
for (entrytype x = 8; x <= 398; x += 2)
{
q->append(x);
}
entrytype frontEl; // to store the front of the queue
// get the front of the queue
q->front(frontEl);
cout << "Front of the queue: " << frontEl << endl;
// remove the first two elements of the queue
cout << "Popped 2 elements from the queue\n";
q->pop();
q->pop();
// check if 8 exists in the queue
cout << "Does 8 exists in the queue? " << q->find(8) << endl;
// check if 200 exists in the queue
cout << "Does 200 exists in the queue? " << q->find(200) << endl;
// report the size of the queue
cout << "Current size of the queue: " << q->size() << endl;
// remove 10 elements from the queue
for (int i = 0; i < 10; i++)
q->pop();
cout << "Popped 10 elements from the queue\n";
// get the new size of the queue
cout << "Updated size of the queue: " << q->size() << endl;
// get the front of the queue
q->front(frontEl);
cout << "Front of the queue: " << frontEl << endl;
return 0;
}
Step by stepSolved in 3 steps with 2 images

- 1. Complete the function evaluate_postfix(String exp): Input: "10 23 - 5 15 + +" Retur: 7 Node.java public class Node {Object info;Node next;Node(Object info, Node next){this.info=info;this.next=next;}}// "((1+2)"// ['(','(','1','+','2',')']// stack : ')' -> '2' -> '1' -> '(' -> '(' -> null Stack.java public class Stack {private Node top;public Stack() {top = null;}public boolean isEmpty() {return (top == null);}public void push(Object newItem) {top = new Node(newItem, top);}public Object pop() {if (isEmpty()) {System.out.println("Trying to pop when stack is empty");return null;} else {Node temp = top;top = top.next;return temp.info;}}void popAll() {top = null;}public Object peek() {if (isEmpty()) {System.out.println("Trying to peek when stack is empty");return null;} else {return top.info;}}} Runner.java public class Runner {public static void main(String[] args) {String expression1 = "((1+2)))))+(2+2))(1/2)";boolean res =…arrow_forward/** * This class will use Nodes to form a linked list. It implements the LIFO * (Last In First Out) methodology to reverse the input string. * **/ public class LLStack { private Node head; // Constructor with no parameters for outer class public LLStack( ) { // to do } // This is an inner class specifically utilized for LLStack class, // thus no setter or getters are needed private class Node { private Object data; private Node next; // Constructor with no parameters for inner class public Node(){ // to do // to do } // Parametrized constructor for inner class public Node (Object newData, Node nextLink) { // to do: Data part of Node is an Object // to do: Link to next node is a type Node } } // Adds a node as the first node element at the start of the list with the specified…arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forward
- 1. Write code to define LinkedQueue<T> and CircularArrayQueue<T> classes which willimplement QueueADT<T> interface. Note that LinkedQueue<T> and CircularArrayQueue<T>will use linked structure and circular array structure, respectively, to define the followingmethods.(i) public void enqueue(T element); //Add an element at the rear of a queue(ii) public T dequeue(); //Remove and return the front element of the queue(iii) public T first(); //return the front element of the queue without removing it(iv) public boolean isEmpty(); //Return True if the queue is empty(v) public int size(); //Return the number of elements in the queue(vi) public String toString();//Print all the elements of the queuea. Create an object of LinkedQueue<String> class and then add some names of your friendsin the queue and perform some dequeue operations. At the end, check the queue sizeand print the entire queue.b. Create an object from CircularArrayQueue<T> class and…arrow_forwardDefine each of the following data mining functionalities: characterization, discrimination, association and correlation analysis, classification, regression, clustering, and outlier analysis. Give examples of each data mining functionality, using a real-life database that you are familiar with.arrow_forwardIn Java. The following is a class definition of a linked list Node:class Node{int info;Node next;}Show the instructions required to create a linked list that is referenced by head and stores in order, the int values 13, 6 and 2. Assume that Node's constructor receives no parameters.arrow_forward
- Redesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardcomplete all the implementation of the member functions listed in the class interface for the following header file. #ifndef _LINKEDSTACK#define _LINKEDSTACK #includeusing namespace std;templateclass LinkedStack{private:Node *top; Node *getCurrentTop() { return top; }public: Stack(); bool isEmpty(); bool push(ItemType newItem); bool pop(); ItemType peek(); void clean(); bool display();};#endifarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
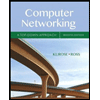
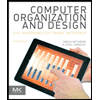
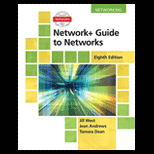
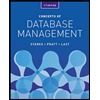
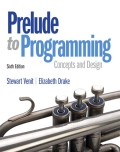
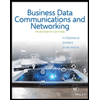