Im trying to create a MIPS program that corrects bad data using Hamming codes. The program is to request the user to enter a 12-bit Hamming code and determine if it is correct or not. If correct, it is to display a message to that effect. If incorrect, it is to display a message saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex. I will be testing only with single bit errors, so the program should be able to correct my tests just fine. You do not need to worry about multiple bit errors.
The following MIPS assembly language
# MIPS program to correct bad data using Hamming codes
.data
prompt: .asciiz "Enter a 12-bit Hamming code (in hex): "
result: .asciiz "The entered code is correct!"
error: .asciiz "The entered code is incorrect. The correct code is: "
newline: .asciiz "\n"
.text
.globl main
main:
# Display prompt and read input from user
li $v0, 4 # syscall code for printing a string
la $a0, prompt # load address of prompt string into $a0
syscall # print prompt
li $v0, 5 # syscall code for reading an integer
syscall # read 12-bit Hamming code into $v0
# Calculate the parity bits
move $t0, $v0 # move the code into temporary register $t0
andi $t1, $t0, 0x924 # compute parity bit P1
andi $t2, $t0, 0x492 # compute parity bit P2
andi $t3, $t0, 0x249 # compute parity bit P3
andi $t4, $t0, 0x3f # compute data bits D1-D6
xor $t5, $t1, $t2 # compute parity bit P4
xor $t6, $t3, $t4 # compute parity bit P5
xor $t7, $t5, $t6 # compute parity bit P6
# Check if any bit is incorrect and correct it if necessary
beqz $t7, correct # if all parity bits are 0, the code is correct
li $t8, 0 # initialize a counter for the incorrect bit
beqz $t5, check_p2 # if P1 is correct, check P2
addi $t8, $t8, 2 # bit 2 is incorrect (P1)
check_p2:
beqz $t6, check_d1 # if P2 is correct, check D1
addi $t8, $t8, 1 # bit 1 is incorrect (P2)
check_d1:
beqz $t4, check_p4 # if D1 is correct, check P4
addi $t8, $t8, 4 # bit 4 is incorrect (D1)
check_p4:
beqz $t5, check_p3 # if P4 is correct, check P3
addi $t8, $t8, 8 # bit 8 is incorrect (P4)
check_p3:
beqz $t3, check_d2 # if P3 is correct, check D2
addi $t8, $t8, 16 # bit 16 is incorrect (P3)
check_d2:
beqz $t2, check_d3 # if D2 is correct, check D3
addi $t8, $t8, 32 # bit 32 is incorrect (D2)
check_d3:
beqz $t1, check_d4 # if D3 is correct, check D4
addi $t8,$t8, 64 # bit 64 is incorrect (D3)
check_d4:
addi $t8, $t8, 128 # bit 128 is always incorrect (D4)
li $t9, 1 # set the correction bit to 1
sll $t9, $t9, $t8 # shift the correction bit to the correct position
xor $t0, $t0, $t9 # correct the bit
# Display result
li $v0, 4 # syscall code for printing a string
beqz $t7, print_result # if the code is correct, print result message
la $a0, error # load address of error message into $a0
syscall # print error message
li $v0, 34 # syscall code for printing an integer in hex
move $a0, $t0 # load the corrected code into $a0
syscall # print the corrected code in hex
j end # exit the program
print_result:
la $a0, result # load address of result message into $a0
syscall # print result message
end:
li $v0, 10 # syscall code for terminating the program
syscall # terminate the program
Here's the error:
Error in C:\Users\supre\Documents\Downloads\Assignment10.asm line 56 column 15: "$t8": operand is of incorrect type
Assemble: operation completed with errors.
Im trying to create a MIPS program that corrects bad data using Hamming codes. The program is to request the user to enter a 12-bit Hamming code and determine if it is correct or not. If correct, it is to display a message to that effect. If incorrect, it is to display a message saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex. I will be testing only with single bit errors, so the program should be able to correct my tests just fine. You do not need to worry about multiple bit errors.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

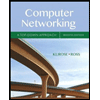
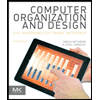
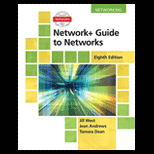
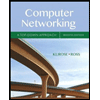
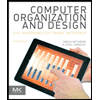
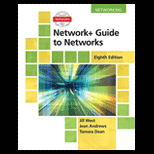
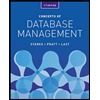
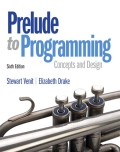
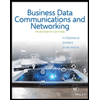