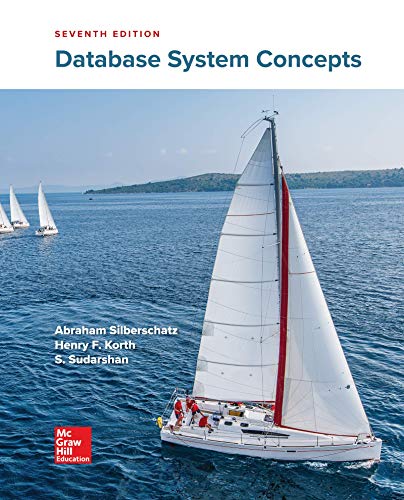
Concept explainers
9. using c++,
Implement a bubble sort that accepts an array of integers a, and an integer aSize, and sorts a in DECENDING order. Determine the execution speed for your bubble sort as the array length increases from 100 to 1000, 10000, 100000, and 250000 elements. Use the attached code fragment to time your bubbleSort function.
Place a brief, but well-written summary of your results in the .cpp file.
#include <iostream>
#include <ctime>
#include <thread>
using namespace std;
int main() {
int x = 0, y = 100000;
// get starting value of timer
clock_t start = std::clock();
//code to be timed goes here
do_something();
// get ending value of timer
clock_t end = std::clock();
std::cout << (double)(end - start) / CLOCKS_PER_SEC << std::endl;
}

Implement a bubble sort that accepts an array of integers a, and an integer aSize, and sorts a in DECENDING order. Determine the execution speed for your bubble sort as the array length increases from 100 to 1000, 10000, 100000, and 250000 elements. Use the attached code fragment to time your bubbleSort function.
Step by stepSolved in 2 steps

- PYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forwardIn c++, please! Thank you! Write a void function SelectionSortDescendTrace() that takes an integer array and sorts the array into descending order. The function should use nested loops and output the array after each iteration of the outer loop, thus outputting the array N-1 times (where N is the size). Complete main() to read in a list of up to 10 positive integers (ending in -1) and then call the SelectionSortDescendTrace() function. If the input is: 20 10 30 40 -1 then the output is: 40 10 30 20 40 30 10 20 40 30 20 10 The following code is given: #include <iostream> using namespace std; // TODO: Write a void function SelectionSortDescendTrace() that takes// an integer array and the number of elements in the array as arguments,// and sorts the array into descending order.void SelectionSortDescendTrace(int numbers [], int numElements) { } int main() { int input, i = 0; int numElements = 0; int numbers [10]; // TODO: Read in a list of up to 10 positive…arrow_forward2. Fill in the for-loop below with the correct parameters to make room for the new value. saved Let's say that you have to write a function to insert an element into an ordered array. It takes an array A, a size, an index and a value. The value needs to be inserted at Aſindex] without losing values that are already in the array. // Assume that the index is found to be within array bounds and there is room to insert. for (int i = ?; ?; ?) { A[i]=A[i-1]; } A[index]=value; size++; O 0; i 0; - O size; i> index; -- O size-1; i>= index; i--arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
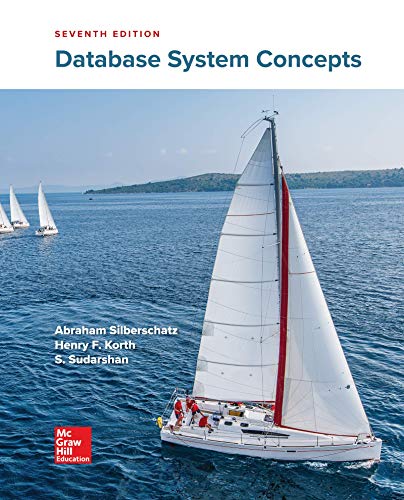
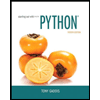
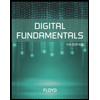
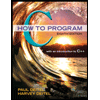
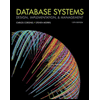
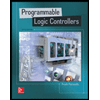