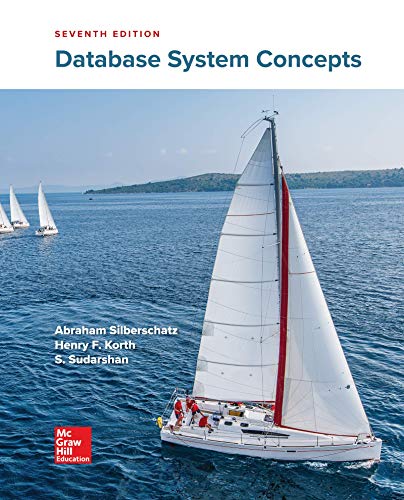
Concept explainers
Implement in C Programming
7.9.1: LAB: BankAccount struct
Given main(), build a struct called BankAccount that manages checking and savings accounts. The struct has three data members: a customer name (string), the customer's savings account balance (double), and the customer's checking account balance (double). Assume customer name has a maximum length of 20.
Implement the BankAccount struct and related function declarations in BankAccount.h, and implement the related function definitions in BankAccount.c as listed below:
- BankAccount InitBankAccount(char* newName, double amt1, double amt2) - set the customer name to parameter newName, set the checking account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount)
- BankAccount SetName(char* newName, BankAccount account) - set the customer name
- void GetName(char* customerName, BankAccount account) - return the customer name in customerName
- BankAccount SetChecking(double amt, BankAccount account) - set the checking account balance to parameter amt
- double GetChecking(BankAccount account) - return the checking account balance
- BankAccount SetSavings(double amt, BankAccount account) - set the savings account balance to parameter amt
- double GetSavings(BankAccount account) - return the savings account balance
- BankAccount DepositChecking(double amt, BankAccount account) - add parameter amt to the checking account balance (only if positive)
- BankAccount DepositSavings(double amt, BankAccount account) - add parameter amt to the savings account balance (only if positive)
- BankAccount WithdrawChecking(double amt, BankAccount account) - subtract parameter amt from the checking account balance (only if positive)
- BankAccount WithdrawSavings(double amt, BankAccount account) - subtract parameter amt from the savings account balance (only if positive)
- BankAccount TransferToSavings(double amt, BankAccount account) - subtract parameter amt from the checking account balance and add to the savings account balance (only if positive)
BankAccount.h
/* TODO: Type your header file guards and include directives here. */
/* Type your code here. */
BankAccount.c
/* TODO: Type your header file guards and include directives here. */
/* Type your code here. */
main.c
#include <stdio.h>
#include <string.h>
#include "BankAccount.h"
int main() {
BankAccount account = InitBankAccount("Mickey", 500.00, 1000.00);
char name[20];
account = SetChecking(500, account);
account = SetSavings(500, account);
account = WithdrawSavings(100, account);
account = WithdrawChecking(100, account);
account = TransferToSavings(300, account);
GetName(name, account);
printf("%s\n", name);
printf("$%.2f\n", GetChecking(account));
printf("$%.2f\n", GetSavings(account));
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 5 images

- WRITE IN C++ A Date class is described by month(m), day(d), year(y). An Employee is described by a unique data structure having a name (e.g. “George”), salary(75000.25) and date_of_birth (06, 10, 1998). Calculate the number of days between his day of birth and (09, 20, 2023). Assume that the Employee object contains a Date sub-object)arrow_forwardWrite a C#program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string) ClassRegistration -…arrow_forwardWrite a C++ program that simulates a two-dimensional random walk along a grid that has M by N function parameters.The walker moves in one of four directions. The walker chooses the direction randomly. The walker cannot move off the grid. The function ends when the walker visits every grid position at least once. The function returns a dynamic structure that is an ordered record of the walker's positions over time. The first element of the structure is the walker's first position. The last element is the walker's last position.arrow_forward
- Implement a C++ program:RESTAURANT that has multiple branches, and each branch has menus of food items, their stock and a list of customers. A branch may have for example a breakfast menu and lunch menu with different food items, and the stock (available quantity) of each food item in the branch. Also, the branch will have a list of regular customers and their contact information to contact them for offers and new food items. Class Names Data and Member Functions Food Data Members: ID, Name, Calories, Price Member Functions: getID, getName, getCalories, getPrice setID, setName, setCalories, setPrice Stock Data Members: ID, Food, Stock Member Functions: getID, getFood, getStock setID, setFood, setStock Customer Data Members: ID, Name, Phone Member Functions: getID, getName, getPhone setID, setName, setPhoe Menu Data Members: ID, Name, foodList Member Functions: getID, getName, getFoodList setID, setName Branch Data Members: ID, Address, menuList, stockList, customerList Member…arrow_forwardUsing C++ Without Using linked lists: Create a class AccessPoint with the following: x - a double representing the x coordinate y - a double representing the y coordinate range - an integer representing the coverage radius status - On or Off Add constructors. The default constructor should create an access point object at position (0.0, 0.0), coverage radius 0, and Off. Add accessor and mutator functions: getX, getY, getRange, getStatus, setX, setY, setRange and setStatus. Add a set function that sets the location coordinates and the range. Add the following member functions: move and coverageArea. Add a function overLap that checks if two access points overlap their coverage and returns true if they do. Add a function signalStrength that returns the wireless signal strength as a percentage. The signal strength decreases as one moves away from the access point location. Represent this with bars like, IIIII. Each bar can represent 20% Test your class by writing a main function that…arrow_forwardWrite a C# program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string)arrow_forward
- Write Java code Objectives •Use an abstract data type for a list •Use a method that contains an Object type as a formal parameter •Use methods in an existing class •Access data members within a class Required Files: •ListInterface.java •ListException.java •ListOutOfBoundsException.java •ListArrayBased.java Description A list is an abstract data type used to store ordered data, and contains operations that fall into the following categories: (1) add data to a data collection, (2) remove data from a data collection, and (3) ask questions about data in a data collection. The operations which ask questions about the data in a data collection may include operations that determine if a data collection is empty, determine the size of a data collection, and retrieve the item at a given position in a data collection. A list should be able to contain any type of object. The methods for the List support any object as specified in the interface. In this assignment, the objects will be S trings.…arrow_forwardWrite a C++ program, you will create a class called Books to hold the information about the books in a library. The information required for each book is as follows Book Title Author name Publisher Publish year Subject Book ID (identical 5 digits’ number) Implement the following getBookInfo () member function that receives a pointer to a book and fills it up with user data. User input validation is necessary. The user should be properly prompted for each field. void getBookInfo (Books *b) Implement the following printBookInfo () member function. void printBookInfo (Books *b) Your function should print the book in the following format: Book Title : Object Oriented Programming Using C++ Author : Chris M. Szalwinski Publisher : Amazon Year : 2016 Subject : Computer Science Book Id : 00034 Note: You need to add required constructors/destructors, member functions or data members/variables in your program to complete its execution. //Missing lines of codes to be completed by the student }…arrow_forwardcode this in RUBY programming languagearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
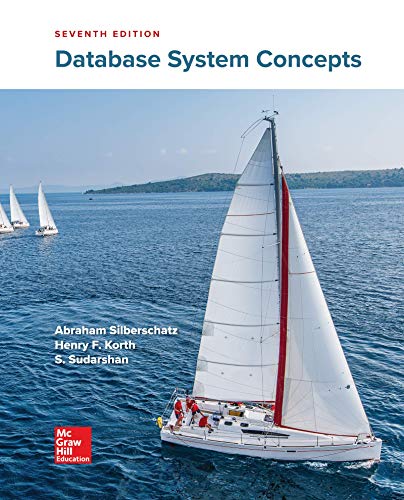
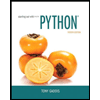
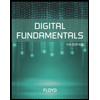
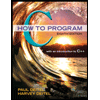
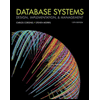
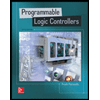