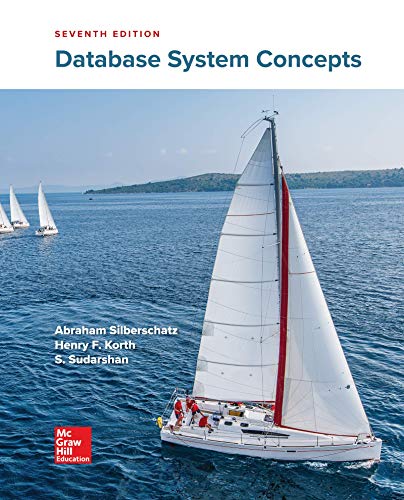
Implement in C Programing
7.10.1: LAB: Product struct
Given main(), build a struct called Product that will manage product inventory. Product struct has three data members: a product code (string), the product's price (double), and the number count of product in inventory (int). Assume product code has a maximum length of 20.
Implement the Product struct and related function declarations in Product.h, and implement the related function definitions in Product.c as listed below:
- Product InitProduct(char *code, double price, int count) - set the data members using the three parameters
- Product SetCode(char *code, Product product) - set the product code (i.e. SKU234) to parameter code
- void GetCode(char *productCode, Product product) - return the product code in productCode
- Product SetPrice(double price, Product product) - set the price to parameter product
- double GetPrice(Product product) - return the price
- Product SetCount(int count, Product product) - set the number of items in inventory to parameter num
- int GetCount(Product product) - return the count
- Product AddInventory(int amt, Product product) - increase inventory by parameter amt
- Product SellInventory(int amt, Product product) - decrease inventory by parameter amt
Ex. If a new Product struct is created with code set to "Apple", price set to 0.40, and the count set to 3, the output is:
Name: Apple Price: 0.40 Count: 3
Ex. If 10 apples are added to the Product struct's inventory, but then 5 are sold, the output is:
Name: Apple Price: 0.40 Count: 8
Ex. If the Product struct's code is set to "Golden Delicious", price is set to 0.55, and count is set to 4, the output is:
Name: Golden Delicious Price: 0.55 Count: 4
Product.c
#include <stdio.h>
#include <string.h>
#include "Product.h"
/* Type your code here */
Product.h
#ifndef PRODUCT_H_
#define PRODUCT_H_
//TODO: Create Product struct
/* Type your code here */
#endif
main.c

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- WRITE IN C++ A Date class is described by month(m), day(d), year(y). An Employee is described by a unique data structure having a name (e.g. “George”), salary(75000.25) and date_of_birth (06, 10, 1998). Calculate the number of days between his day of birth and (09, 20, 2023). Assume that the Employee object contains a Date sub-object)arrow_forwardWrite a C++ program that simulates a two-dimensional random walk along a grid that has M by N function parameters.The walker moves in one of four directions. The walker chooses the direction randomly. The walker cannot move off the grid. The function ends when the walker visits every grid position at least once. The function returns a dynamic structure that is an ordered record of the walker's positions over time. The first element of the structure is the walker's first position. The last element is the walker's last position.arrow_forwardImplement a C++ program:RESTAURANT that has multiple branches, and each branch has menus of food items, their stock and a list of customers. A branch may have for example a breakfast menu and lunch menu with different food items, and the stock (available quantity) of each food item in the branch. Also, the branch will have a list of regular customers and their contact information to contact them for offers and new food items. Class Names Data and Member Functions Food Data Members: ID, Name, Calories, Price Member Functions: getID, getName, getCalories, getPrice setID, setName, setCalories, setPrice Stock Data Members: ID, Food, Stock Member Functions: getID, getFood, getStock setID, setFood, setStock Customer Data Members: ID, Name, Phone Member Functions: getID, getName, getPhone setID, setName, setPhoe Menu Data Members: ID, Name, foodList Member Functions: getID, getName, getFoodList setID, setName Branch Data Members: ID, Address, menuList, stockList, customerList Member…arrow_forward
- Develop an algorithm and write a C++ program that counts the letter occurrence in the string; make sure you use call by reference to the array of a class with letter and count as private member attributes when return from parse function. For the class member methods, provide the public accessor and modifier as well as print functions for the private member attributes. Write a test program that reads a C-string and displays the number of letters [a-z] and number of numbers [0-9] in the string. In addition to that, you need to output the histogram of the letter and count array. Here is a sample run of the program: <Output> Enter a string: 2023 is coming The number of letters in 2023 is coming is 8 The number of numbers in 2023 is coming is 4 ---- Histogram ---- Char Count 0 1 2 2 3 1 c 1 g 1 i 2 m 1 n 1 /* not a completed output */ <End Output>arrow_forwardImplement a C++ program for a RESTAURANT that has multiple branches, and each branch has menus of food items, their stock and a list of customers. A branch may have for example a breakfast menu and lunch menu with different food items, and the stock (available quantity) of each food item in the branch. Also, the branch will have a list of regular customers and their contact information to contact them for offers and new food items. Class Names Data and Member Functions Food Data Members: ID, Name, Calories, Price Member Functions: getID, getName, getCalories, getPrice setID, setName, setCalories, setPrice Stock Data Members: ID, Food, Stock Member Functions: getID, getFood, getStock setID, setFood, setStock Customer Data Members: ID, Name, Phone Member Functions: getID, getName, getPhone setID, setName, setPhoe Menu Data Members: ID, Name, foodList Member Functions: getID, getName, getFoodList setID, setName Branch Data Members: ID, Address, menuList, stockList, customerList Member…arrow_forwardImplement in C Programming 7.9.1: LAB: BankAccount struct Given main(), build a struct called BankAccount that manages checking and savings accounts. The struct has three data members: a customer name (string), the customer's savings account balance (double), and the customer's checking account balance (double). Assume customer name has a maximum length of 20. Implement the BankAccount struct and related function declarations in BankAccount.h, and implement the related function definitions in BankAccount.c as listed below: BankAccount InitBankAccount(char* newName, double amt1, double amt2) - set the customer name to parameter newName, set the checking account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount) BankAccount SetName(char* newName, BankAccount account) - set the customer name void GetName(char* customerName, BankAccount account) - return the customer name in customerName BankAccount SetChecking(double amt, BankAccount…arrow_forward
- Consider the following structure definition. Write a C/C++ function that takes an array of struct student with its size and applies a curve system like if the student scored below 50, 1dd 10 points, if it is above 50, add 2 points. The lowest possible mark is 0 and the highest possible mark is 10. struct student{ int ID; int score; void applycurve (struct student a[ ], int size)arrow_forwardWrite a C++ program, you will create a class called Books to hold the information about the books in a library. The information required for each book is as follows Book Title Author name Publisher Publish year Subject Book ID (identical 5 digits’ number) Implement the following getBookInfo () member function that receives a pointer to a book and fills it up with user data. User input validation is necessary. The user should be properly prompted for each field. void getBookInfo (Books *b) Implement the following printBookInfo () member function. void printBookInfo (Books *b) Your function should print the book in the following format: Book Title : Object Oriented Programming Using C++ Author : Chris M. Szalwinski Publisher : Amazon Year : 2016 Subject : Computer Science Book Id : 00034 Note: You need to add required constructors/destructors, member functions or data members/variables in your program to complete its execution. //Missing lines of codes to be completed by the student }…arrow_forwardWrite a class marks with three data members to store three marks. Write three member functions, set_marks() to input marks, sum() to calculate and return the sum and avg() to calculate and return average marks. Code should be in C++arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
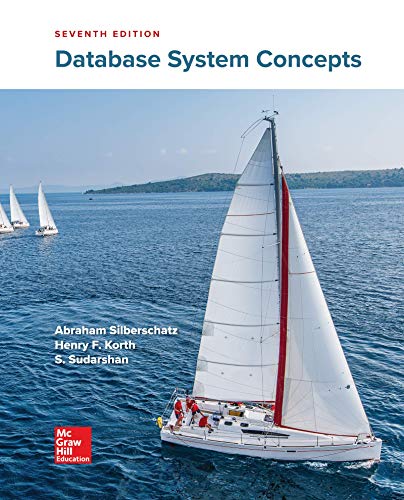
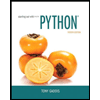
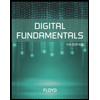
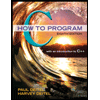
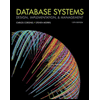
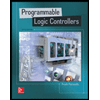