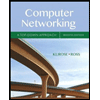
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
(Implement MyMap using open addressing with linear probing)
Create a new concrete class that implements MyMap using open addressing with linear probing. For simplicity, use f(key) = key % size as the hash function, where size is the hash-table size. Initially, the hash-table size is 4. The table size is doubled whenever the load factor exceeds the threshold (0.5).
Test your new MyHashMap class using the code at https://liveexample.pearsoncmg.com/test/Exercise27_01.txt
Class Name: Exercise27_01
If you get a logical or runtime error, please refer https://liveexample.pearsoncmg.com/faq.html
MyHashMap.java located here
https://liveexample.pearsoncmg.com/html/MyHashMapWithLineNumber.html?
IMPORTANT
The answer I require, To submit your code to Revel, you must only submit the code enclosed between
// BEGIN REVEL SUBMISSION
// END REVEL SUBMISSION
// BEGIN REVEL SUBMISSION
/** Return the first value that matches the specified key */ public V get(K key) {
// Perform linear probing
// WRITE YOUR CODE
}
/** Add an entry (key, value) into the map */ public V put(K key, V value) {
// WRITE YOUR CODE
}
}
// End of the MyHashMap class
// END REVEL SUBMISSION
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- can you please type the codeto the following questionarrow_forwardCreate a new concrete class that implements MyMap using open addressing with linear probing. For simplicity, use f(key) = key % size as the hash function, where size is the hash-table size. Initially, the hash-table size is 4. The table size is doubled whenever the load factor exceeds the threshold (0.5). Test your new MyHashMap class using the code at https://liveexample.pearsoncmg.com/test/Exercise27_01.txt Class Name: Exercise27_01 If you get a logical or runtime error, please refer https://liveexample.pearsoncmg.com/faq.html MyHashMap.java located here https://liveexample.pearsoncmg.com/html/MyHashMap.html? MyMap.java located here https://liveexample.pearsoncmg.com/html/MyMap.html IMPORTANT Please do not take answers from Chegg or the rest of the internet, they are all wrong (I checked them). Please only provide a solution if you know the answer. I need to submit the code to Revel, I do not need the entire code, I need to submit the code enclosed between // BEGIN…arrow_forward1. Show the ListStackADT<T> interface 2. Create a ListStackDataStrucClass<T> with the following methods: defaultconstructor, overloaded constructor, copy constructor, getTop, setTop,isEmpty, ifEmpty (if empty throw the exception), push, peek, pop, toString. 3. Create a private inner class of ListStack<T> called StackNode<T> with thefollowing methods: default constructor, overloaded constructor, copyconstructor, getValue, getNext, setValue, setNext 4. Create a BaseConverter class (non-generic) with the following methods: defaultconstructor, inputPrompt, convert [converts a BaseNumber to a convertedString], convertAll [instantiate a String object] , toString, processAndPrint 5. Create a private inner class BaseNumber. The inner class has the following methods: default constructor, overloaded constructor, getNumber, getBase,setNumber, setBase. [Make your private instance variables in the inner classLong type]. 6. Create a BaseConverterDemo class that only…arrow_forward
- Hello. Please answer the attached Hashing in Data Structures question correctly and follow all directions. Please give the solution for the problem. Do not copy the answers already given on Chegg or Bartleby. I just want your own personal answer. *If you solve the problem by yourself and it is correct I will provide a thumbs up for you. Thank you.arrow_forward//From what I can tell, the answer provided does not answer my question. Asking again. Please read. Write a Java program that implements both Linear Search and Binary Search. The program willtake a collection of objects (generic type data) as input and print the number of comparisons neededto find a target element within that collection.You will create the following two Java classes:1. SearchCombo.java : Code for both linearSearch and binarySearch will be in this class. Youmay take help from the textbook Chapter 9, Section 9.1. However, note that the design require-ments are different from the textbook code.•Both search methods must use the Comparable<T> interface and the compareTo() method.•Your program must be able to handle different data types, i.e., use generics.•For binarySearch, if you decide to use a midpoint computation formula that is different fromthe textbook, explain that formula briefly as a comment within your code. 2. Tester.java : This class will contain…arrow_forwardHello. Please answer the attached Hashing in Data Structures question correctly and follow all directions. Please give the solution for the problem. Do not copy the answers already given on Chegg or Bartleby. I just want your own personal answer. *If you would like for me to give you a thumbs up then just answer the question by yourself and do not copy from outside sources. Thank you.arrow_forward
- Create a newconcrete class that implements MyMap using open addressing with quadratic probing.For simplicity, use f(key) = key % size as the hash function, where sizeis the hash-table size. Initially, the hash-table size is 6. The table size is doubledwhenever the load factor exceeds the threshold (0.5).arrow_forwardassume you have a HashMap class that uses a threshold of 0.75 (75%),regardless of the collision resolution mechanism used, and has an initial array size of 13. You may assume the array is resized when the current item to be added will make the load factor greater than or equal to the threshold. Recall that the load factor is the fraction of a hash map that is full. If the array is to be resized, assume the array doubles in size and adds one (2 * size + 1).Table 1 contains a list of items and their associated hash codes that were computed with somehypothetical hash function. Assume the items are added to a newly created instance of the HashMap class in the same order in which they are listed in the table. Based on this information, show what the array of the HashMap would look like after all the items have been added using both of the followinghash collision resolution techniques.1. Separate chaining2. linear probingProvide 2 arrays(one for each problem). One that uses separate…arrow_forwardA) Write a generic Java queue class (a plain queue, not a priority queue). Then, call it GenericQueue, because the JDK already has an interface called Queue. This class must be able to create a queue of objects of any reference type. Consider the GenericStack class shown below for some hints. Like the Stack class below, the GenericQueue should use an underlying ArrayList<E>. Write these methods and any others you find useful: enqueue() adds an E to the queue peek() returns a reference to the object that has been in the queue the longest, without removing it from the queue dequeue() returns the E that has been in the queue the longest, and removes it from the queue contains(T t) returns true if the queue contains at least one object that is equal to t *in the sense that calling .equals() on the object with t the parameter returns true.* Otherwise contains returns false. size() and isEmpty() are obvious.arrow_forward
- Hello. Please answer the attached Hashing in Data Structures question correctly and follow all directions. Please give the solution for the problem. Do not copy the answers already given on Chegg or Bartleby. I just want your own personal answer. *If you would like for me to give you a thumbs up then just answer the question by yourself and do not copy from outside sources. Thank you.arrow_forwardWrite the full Java code for LabProgram.javaarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
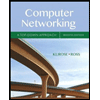
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
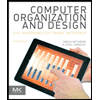
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
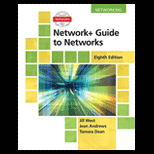
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
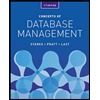
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
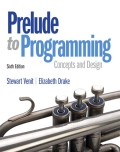
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
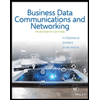
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY