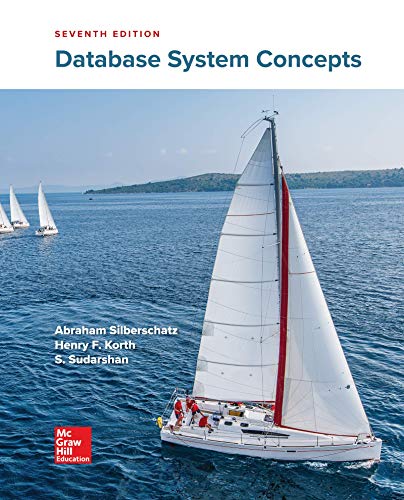
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Make a copy of an ArrayList<String> with an explicit loop. Complete the following code.// Use a for loop to copy the contents of words
*my solution (for loop) says incompatible types, not sure what to do
![Make a copy of an ArrayList<String> with an explicit loop. Complete the following code.
ArrayListCopy.java
1 import java.util.ArrayList;
2 import java.util.Scanner;
3
4 public class ArrayListCopy
5 {
public static void main(String[] args)
{
ArrayList<String> words = new ArrayList<String>();
Scanner in = new Scanner(System.in);
while (in.hasNext()) words.add(in.next());
7
8
10
11
12
13
ArrayList<String> copy0fWords = new ArrayList<String>();
// Use a for loop to copy the contents of words
for (int i = 0; i < copy0fWords.size(); i++)
{
words =
14
15
16
17
copy0fWords.get (i);
18
19
20
}
words.remove(0); // Shouldn't affect the copy
System.out.println(words);
System.out.println(copy0fWords);
}
21
22
23 }](https://content.bartleby.com/qna-images/question/d772767f-fd91-4ca5-b737-b5766e3fef0a/c77aecbf-fde9-4f72-b8d1-deeb1a8f9431/27n1hye_thumbnail.png)
Transcribed Image Text:Make a copy of an ArrayList<String> with an explicit loop. Complete the following code.
ArrayListCopy.java
1 import java.util.ArrayList;
2 import java.util.Scanner;
3
4 public class ArrayListCopy
5 {
public static void main(String[] args)
{
ArrayList<String> words = new ArrayList<String>();
Scanner in = new Scanner(System.in);
while (in.hasNext()) words.add(in.next());
7
8
10
11
12
13
ArrayList<String> copy0fWords = new ArrayList<String>();
// Use a for loop to copy the contents of words
for (int i = 0; i < copy0fWords.size(); i++)
{
words =
14
15
16
17
copy0fWords.get (i);
18
19
20
}
words.remove(0); // Shouldn't affect the copy
System.out.println(words);
System.out.println(copy0fWords);
}
21
22
23 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- This code gets an error for going out of range in the last for loop. What can I do to fix this? # Lucas Conklin# 5772707import csvimport statistics def readCSVIntoDictionary(f_name): data = [] with open(f_name) as f: reader = csv.reader(f) for row in reader: if not data: for index in range(len(row)): data.append([]) for index in range(len(row)): data[index].append(float(row[index])) f.close() return data features = readCSVIntoDictionary("C:\\Users\\lucas\\Downloads\\pima.csv")print(features) def find_median_and_SD(data, feature): med = statistics.median(data[feature]) rounded_med = round(med, 4) st_dev = statistics.stdev(data[feature]) rounded_st_dev = round(st_dev, 5) return rounded_med, rounded_st_dev for i in range(0, len(features)): (median, st_dev) = find_median_and_SD(features, i) print(f'Feature {i} Median: {median} Standard Deviation: {st_dev}')…arrow_forward// Only few methods are mentioned here //Do remaining methods to pass the test methods /*** Removes the specified (num) of the given element from the internal ArrayList of elements. * If num <= 0, don't remove anything. * If num is too large, remove all instances of the given element from the internal ArrayList of elements.* Example:* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 1, 2, 1* - Calling remove(2, 1) would remove the first 2 instances of 1 * - The CustomIntegerArrayList will then contain the integers: 2, 2, 1* - For a defined CustomIntegerArrayList containing the integers: 100, 100, 100 * - Calling remove(4, 100) would remove all instances of 100 * - The CustomIntegerArrayList will then be empty* - For a defined CustomIntegerArrayList containing the integers: 5, 5, 5, 5, 5 * - Calling remove(0, 5) would remove nothing* * @param num number of instances of element to remove * @param element to remove*/ public void remove(int num, int element) {//…arrow_forwardModify the quick sort implementation in the textbook to sort the array using pivot as the median of the first, last, and middle elements of the array. Add the modified quick sort implementation to the arrayListType class provided (arrayListType.h). Ask the user to enter a list of positive integers ending with -999, sort the integers, and display the pivots for each iteration and the sorted array. Main Function #include <iostream>#include "arrayListType.h"using namespace std; int main(){ arrayListType<int> list;int num;cout << "Line 8: Enter numbers ending with -999" << endl;cin >> num; while (num != -999){list.insert(num);cin >> num;} cout << "Line 15: The list before sorting:" << endl;list.print();cout << endl; list.selectionSort();cout << "Line 19: The list after sorting:" << endl;list.print();cout << endl; return 0;} Header File (arrayList.h) Including images #include <iostream>#include <cassert>…arrow_forward
- Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, then backwards. End each loop with a newline. Ex: If courseGrades = {7, 9, 11, 10}, print:7 9 11 10 10 11 9 7 Hint: Use two for loops. Second loop starts with i = NUM_VALS - 1. Note: These activities may test code with different test values. This activity will perform two tests, both with a 4-element array (int courseGrades[4]). Also note: If the submitted code tries to access an invalid array element, such as courseGrades[9] for a 4-element array, the test may generate strange results. Or the test may crash and report "Program end never reached", in which case the system doesn't print the test case that caused the reported message. #include <iostream>using namespace std; int main() { const int NUM_VALS = 4; int courseGrades[NUM_VALS]; int i; for (i = 0; i < NUM_VALS; ++i) { cin >> courseGrades[i]; } /* Your solution goes…arrow_forwardHow can I evaluate an ArrayList's efficiency?arrow_forwardHow can | iterate over the ArrayList made up of integers using the while loops in the java programming language?arrow_forward
- Define a collection “ArrayList” to store the students' names. Use a loop to take 5 students’ names from the user and store them in the collection. Add two student names to the collection and remove the last name in the list. Insert a student name in third place in the collection. Sort the names alphabetically. Ask the user to enter a student name and search for it in the collection and prints the result as “Found” or “Not Found”. Print the whole collection using the enhanced for loop. (java)arrow_forwardpublic static void reverse(ArrayList<Integer> alist){}public static ArrayList<Integer> reverseList(ArrayList<Integer> alist){} How to use these two headers method and a main method to create the output of [1,2,3] [3,2,1]arrow_forwardTo declare an array of String called myStrings, we would code: ArrayList<Blank 1> myStrings = Blank 2 ArrayList<String>();arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
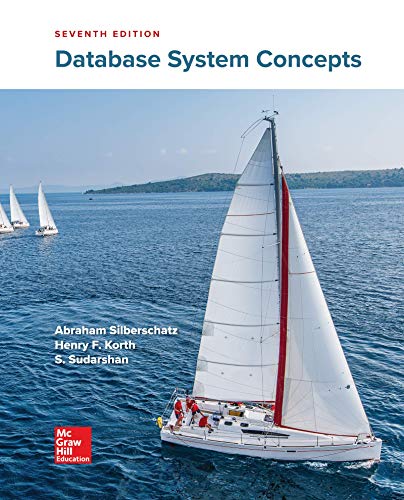
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
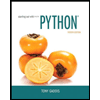
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
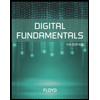
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
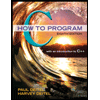
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
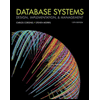
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
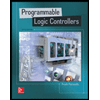
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education