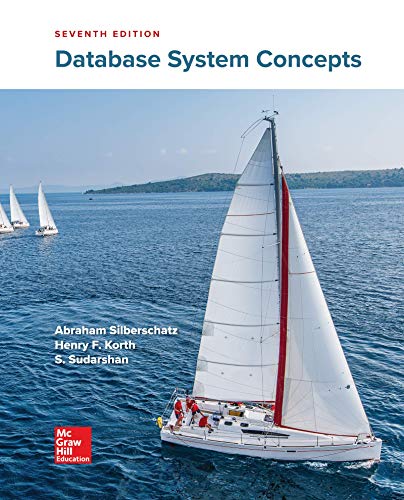
Code:
import java.util.Scanner;
public class ReceiptMaker {
public static final String SENTINEL = "checkout";
public final int MAX_NUM_ITEMS;
public final int TAX_RATE;
private String itemNames;
private double [] itemPrices;
private int numItemsPurchased;
public ReceiptMaker(){
MAX_NUM_ITEMS = 10;
TAX_RATE = .0875;
itemNames = new String[MAX_NUM_ITEMS];
itemPrices = new double[MIN_NUM_ITEMS];
numItemsPurchased = 0;
}
public ReceiptMaker(int maxNumItems, double taxRate){
if(isValid(maxNumItems)){
MAX_NUM_ITEMS = maxNumItems;
}
else{
MAX_NUM_ITEMS= 10;
}
if(isValid(taxRate)){
TAX_RATE = taxRate;
}
else{
TAX_RATE = .0875;
}
itemNames = new String[MAX_NUM_ITEMS];
itemPrices = new double[MAX_NUM_ITEMS];
numItemsPurchased = 0;
}
public void greetUser(){
System.out.println("Welcome to the "+MAX_NUM_ITEMS+" items or less checkout line");
}
public void promptUserForProductEntry(){
System.out.println("Enter item #"+(numItemsPurchased+1)+"'s name and price separated by a space, or enter ""+SENTINEL+"\" to end transaction early");
}
public void addNextPurchaseItemFromUser(String itemName, double itemPrice){
itemNames[numItemsPurchased] = itemName;
itemPrices[numItemsPurchased] = itemPrice;
numItemsPurchased++;
}
public double getSubtotal(){
double subTotal = 0;
for(int i=0; i<numItemsPurchased; i++){
subTotal += itemPrices[i];
}
return subTotal;
}
public double getMinPrice(){
double minPrice = Integer.MIN_VALUE;
for(int i=0; i<numItemsPurchased; i++){
if(itemPrices[i]<minPrice){
minPrice = itemPrices[i];
}
}
return minPrice;
}
public int getIndexOfMinPrice(){
int indexOfMin = 0;
for(int i=1; i<numItemsPurchased; i++){
if(itemPrices[i]< itemPrices[indexOfMin]){
indexOfMin = i;
}
}
return indexOfMin;
}
public double getMaxPrice(){
double maxPrice = Integer.MIN_VALUE;
for(int i=0; i<numItemsPurchased; i++){
if(itemPrices[i] > maxPrice){
maxPrice = itemPrices[i];
}
}
return maxPrice;
}
public int getIndexOfMaxPrice(){
int indexOfMax = 0;
for(int i=1; i<numItemsPurchased; i++){
if(itemPrices[i] > itemPrices[indexOfMax]){
indexOfMax = numItemsPurchased;
}
}
return indexOfMax;
}
public double getMeanPrice(){
return getSubtotal()/numItemsPurchased;
}
public double getTaxOnSubtotal(){
return getSubtotal() * TAX_RATE;
}
public double getTotal(){
return getSubtotal() + getTaxOnSubtotal();
}
public void displayReceipt(){
System.out.println("-------------------------------------------------");
System.out.printf("Subtotal: $ %04.2f | # of Items %02d\n", getSubtotal(),numItemsPurchased);
System.out.printf(" Tax: $ %05.2f \n",getTaxOnSubtotal());
System.out.printf(" Total: $ %04.2f \n", getTotal());
System.out.println("--------------------THANK YOU--------------------");
}
public void displayReceiptStats(){
System.out.println("\n-----------------RECEIPT STATS-----------------");
System.out.printf("Min Item Name: %12s | Price: $ %04.2f\n"+ itemNames[getIndexOfMinPrice()], getMinPrice());
System.out.print("Max Item Name: %12s | Price: $ %04.2f\n", itemNames[getIndexOfMaxPrice()], getMaxPrice());
System.out.printf("Mean price of %02d items purchased: $ %04.2f\n\n", numItemsPurchased, getMeanPrice());
}
public void displayAllItemsWithPrices(){
System.out.println("\n---------------RECEIPT BREAKDOWN---------------");
for(int i=0; i<numItemsPurchased; i++){
System.out.printf("Item #%02d Name: %3s | Price: $ %04.2f\n",(i+1), itemNames[i], itemPrices[i]);
}
}
private double getItemPriceFromUser(Scanner scanner){
//does not handle InputMismatchException
double price = -1;
while( (price = scanner.nextDouble()) < 0 ){
System.out.println("Price \""+price+ "\" cannot be negative.\nReenter price");
}
return price;
}
public void scanCartItems(Scanner scanner){
while(numItemsPurchased < MAX_NUM_ITEMS){
promptUserForProductEntry();
String token1;
double token2=-1;
if(!(token1 = scanner.next()).equalsIgnoreCase(SENTINEL)){
token2 = getItemPriceFromUser(scanner);
addNextPurchaseItemFromUser(token1, token2);
}
else{
break;
}
}
}
private boolean isValid(int a) {
return (a >= 0);
}
private boolean isValid(double a) {
return (a >= 0);
}
public static void main(String [] args){
Scanner scanner = new Scanner(System.in);
ReceiptMaker rm = new ReceiptMaker();
rm.greetUser();
rm.scanCartItems(scanner);
rm.displayReceipt();
rm.displayReceiptStats();
rm.displayAllItemsWithPrices();
scanner.close();
}
}
Result:
-------------------------------------------------
Subtotal: $ 44.98 | # of Items 10
Tax: $ 03.94
Total: $ 48.92
--------------------THANK YOU--------------------
-----------------RECEIPT STATS-----------------
Min Item Name: ice | Price: $ 1.99
Max Item Name: hummus | Price: $ 7.63
Mean price of 10 items purchased: $ 4.50
---------------RECEIPT BREAKDOWN---------------
Item #01 Name: apples | Price: $ 5.32
Item #02 Name: bananas | Price: $ 3.78
Item #03 Name: cantaloupe | Price: $ 2.99
Item #04 Name: donuts | Price: $ 6.55
Item #05 Name: eggs | Price: $ 4.87
Item #06 Name: flour | Price: $ 3.21
Item #07 Name: grapes | Price: $ 5.89
Item #08 Name: hummus | Price: $ 7.63
Item #09 Name: ice | Price: $ 1.99
Item #10 Name: jello | Price: $ 2.75

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- class Player { protected: string name; double weight; double height; public: Player(string n, double w, double h) { name = n; weight = w; height = h; } string getName() const { return name; } virtual void printStats() const = 0; }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, double w, double h, int fg, int a) : Player(n, w, h) { fieldgoals = fg; attempts = a; } void printStats() const { cout << name << endl; cout << "Weight: " << weight; cout << " Height: " << height << endl; cout << "FG: " << fieldgoals; cout << " attempts: " << attempts; cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; a. What does = 0 after function printStats() do? b. Would the following line in main() compile: Player p; -- why or why not? c. Could…arrow_forwardFor the following four classes, choose the correct relationship between each pair. public class Room ( private String m type; private double m area; // "Bedroom", "Dining room", etc. // in square feet public Room (String type, double area) m type type; m area = area; public class Person { private String m name; private int m age; public Person (String name, int age) m name = name; m age = age; public class LivingSSpace ( private String m address; private Room[] m rooms; private Person[] m occupants; private int m countRooms; private int m countoccupants; public LivingSpace (String address, int numRooms, int numoccupants) m address = address; new int [numRooms]; = new int [numOceupants]; m rooms %3D D occupants m countRooms = m countOccupants = 0; public void addRoom (String type, double area)arrow_forwardPublic classTestMain { public static void main(String [ ] args) { Car myCar1, myCar2; Electric Car myElec1, myElec2; myCar1 = new Car( ); myCar2 = new Car("Ford", 1200, "Green"); myElec1 = new ElectricCar( ); myElec2 = new ElectricCar(15); } }arrow_forward
- Subclass toString should call include super.toString(); re-submit these codes. public class Vehicle { private String registrationNumber; private String ownerName; private double price; private int yearManufactured; Vehicle [] myVehicles = new Vehicle[100]; public Vehicle() { registrationNumber=""; ownerName=""; price=0.0; yearManufactured=0; } public Vehicle(String registrationNumber, String ownerName, double price, int yearManufactured) { this.registrationNumber=registrationNumber; this.ownerName=ownerName; this.price=price; this.yearManufactured=yearManufactured; } public String getRegistrationNumber() { return registrationNumber; } public String getOwnerName() { return ownerName; } public double getPrice() { return price; } public int getYearManufactured() { return yearManufactured; }…arrow_forwardpublic class LabProgram { public static void main(String args[]) { Course course = new Course(); String first; // first name String last; // last name double gpa; // grade point average first = "Henry"; last = "Cabot"; gpa = 3.5; course.addStudent(new Student(first, last, gpa)); // Add 1st student first = "Brenda"; last = "Stern"; gpa = 2.0; course.addStudent(new Student(first, last, gpa)); // Add 2nd student first = "Jane"; last = "Flynn"; gpa = 3.9; course.addStudent(new Student(first, last, gpa)); // Add 3rd student first = "Lynda"; last = "Robison"; gpa = 3.2; course.addStudent(new Student(first, last, gpa)); // Add 4th student course.printRoster(); } } // Class representing a student public class Student { private String first; // first name private String last; // last name private double gpa; // grade point average…arrow_forwardpublic class Book { protected String title; protected String author; protected String publisher; protected String publicationDate; public void setTitle(String userTitle) { title = userTitle; } public String getTitle() { return title; } public void setAuthor(String userAuthor) { author = userAuthor; } public String getAuthor(){ return author; } public void setPublisher(String userPublisher) { publisher = userPublisher; } public String getPublisher() { return publisher; } public void setPublicationDate(String userPublicationDate) { publicationDate = userPublicationDate; } public String getPublicationDate() { return publicationDate; } public void printInfo() { System.out.println("Book Information: "); System.out.println(" Book Title: " + title); System.out.println(" Author: " + author); System.out.println(" Publisher: " + publisher); System.out.println(" Publication…arrow_forward
- class TenNums {private: int *p; public: TenNums() { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = i; } void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }};int main() { TenNums a; a.display(); TenNums b = a; b.display(); return 0;} Continuing from Question 4, let's say I added the following overloaded operator method to the class. Which statement will invoke this method? TenNums TenNums::operator+(const TenNums& b) { TenNums o; for (int i = 0; i < 10; i++) o.p[i] = p[i] + b.p [i]; return o;} Group of answer choices TenNums a; TenNums b = a; TenNums c = a + b; TenNums c = a.add(b);arrow_forwardinterface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forward// This class discounts prices by 10% public class DebugFour4 { public static void main(String args[]) { final double DISCOUNT_RATE = 0.90; int price = 100; double price2 = 100.00; tenPercentOff(price DISCOUNT_RATE); tenPercentOff(price2 DISCOUNT_RATE); } public static void tenPercentOff(int p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println("Ten percent off " + p); System.out.println(" New price is " + newPrice); } public static void tenPercentOff(double p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println"Ten percent off " + p); System.out.println(" New price is " + newprice); } }arrow_forward
- public class Author2 {3 private String name;4 private int numberOfAwards;5 private boolean guildMember;6 private String[] bestsellers;78 public String Author()9 {10 name = "Grace Random";11 numberOfAwards = 0;12 guildMember = false;13 bestsellers = {"Minority Report", "Ubik", "The Man in the HighCastle"};14 }1516 public void setName(String n)17 {18 name = n;19 }20 public String getName()21 {22 return name;23 }2425 public void winsAPulitzer()26 {27 System.out.println(name + " gave a wonderful speech!");28 }29 } 1. This class includes a constructor that begins on line 8; however, it contains an error. Describe the error. 2. Rewrite the constructor header on line 8 with the error identied in part c of this question corrected. 3. Demonstrate how you might overload the constructor for this class. Write only the header. 4. Write a line of Java code to create an instance (object) of this class. Name it someAuthor. 5. Write a line of code to demonstrate how you would use the someAuthor object…arrow_forwardpublic class test{ private static final int MAX = 100; private int[] sco; public test(int[] sco){ this.sco = sco; } private int comAvg() throws IllegalArgumentException{ int sum = 0; for (int i = 0; i < sco.length; i++){ int sc = sco[i]; if (sc < 0 || sc > MAX){ throw new IllegalArgumentException("Score (" + sc + ") is not in the range 0-" + MAX); } sum += sco[i]; } int av = sum / sco.length; return av; } public static void main(String[] args){ test test = new test(new int[] { 50, 70, 81 }); try{ int avgSc = test.comAvg(); char letG; if (avgSc < 60) letG = 'F'; else if (avgSc < 70) letG = 'D'; else if (avgSc < 80) letG = 'C'; else if (avgSc < 90) letG = 'B'; else letG = 'A'; System.out.println("\nAVG Score is " +…arrow_forwardTrace through the following program and show the output. Show your work for partial credit. public class Employee { private static int empID = 1111l; private String name , position; double salary; public Employee(String name) { empID ++; this.name 3 пате; } public Employee(Employee obj) { empID = obj.empĪD; пате %3D оbj.naте; position = obj.position; %3D public void empPosition(String empPosition) {position = empPosition;} public void empSalary(double empSalary) { salary = empSalary;} public String toString() { return name + " "+empID + " "+ position +" $"+salary; public void setName(String empName){ name = empName;} public static void main(String args[]) { Employee empOne = new Employee("James Smith"), empTwo; %3D empOne.empPosition("Senior Software Engineer"); етрOпе.етpSalary(1000); System.out.println(empOne); еmpTwo empTwo.empPosition("CEO"); System.out.println(empOne); System.out.println(empTwo); %3D етpОпе empOne ;arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
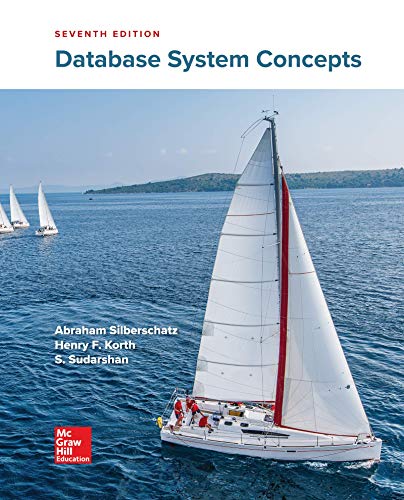
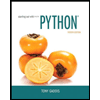
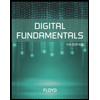
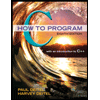
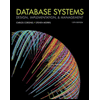
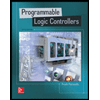