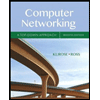
Concept explainers
import java.util.Scanner ; //This program enables the user enters 10 int between -100 and 100 //then a menu is presented to the user to choose an operation to be //performed on the entered numbers. //operations: add all numbers and display their total //display the average of the numbers //find and display all negative numbers //find and display odd numbers //display all numbers in reverse order //display all 5 multiples public class OPonArrays { public static void main (String [] main) { int SIZE = 10; int [] nums= new int [SIZE]; Scanner KB = new Scanner(System.in); // populate the array for(int i = 0; i < 10; i++) { System.out.println("please enter a number between -100 and 100"); nums[i] = KB.nextInt(); } //display all elments for(int i = 0; i < 10; i++) { System .out.println(nums[i]); }
fix the error

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 6 images

- Java - Data Visualization (this is not graded)arrow_forwardC - Right Facing Anglearrow_forwardpython multiple choice Code Example 4-1 def get_username(first, last): s = first + "." + last return s.lower() def main(): first_name = input("Enter your first name: ") last_name = input("Enter your last name: ") username = get_username(first_name, last_name) print("Your username is: " + username) main() 5. Refer to Code Example 4-1: What is the scope of the variable named s ?a. globalb. localc. global in main() but local in get_username()d. local in main() but global in get_username()arrow_forward
- Student name: CIS 232 Introduction to Programming Homework Assignment 10 Due Date: 11/23/2020 Instructor: Dr. Lomako Problem Statement: Write a Java program with the main method and a multiplication method (must be created, not the library method). Create “HW10_lastname.java” program that: Prints a program title Creates two n x n square matrices A and B and initializes them with random values Displays the matrices A and B Calls the multiplication method that multiplies two square matrices and returns a matrix AB that is the result of the multiplication. Prints AB matrixarrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardA dual-tone multi-frequency (DTMF) system is used to produce signals that represent the dialed digits in a telephone system. A combination of a horizontal frequency and a vertical frequency is produced whenever a button is pressed on the keypad. The figure below shows a telephone keypad and the different frequencies used. 697 Hz 770 Hz 852 Hz 941 Hz 1209 Hz 1 4 7 1336 Hz 2 1477 Hz 3 5 6 8 9 # 0 * Generate the DTMF tone decoder that will take an audio file the DTMF signal of your student number. The student number must be presented as one complete string (e.g. 2020123456)arrow_forward
- NumberFun.py # using multiple functions. # copy/paste this program to run it first, you are supposed to use Google Chrome as Internet Browser for this course. # 1. calculate sum of the first n natural odd numbers, e.g.:1,3,5,7,9... def totalN(n): totalN = 0 for i in range(1,2*n+1,2): totalN = totalN + i return totalN # 2. calculate sum of the square of the first m natural odd numbers, e.g.:1,9,25,49,81... ### After you define/write/complete the function totalMSquare(m), remove the # sign as below to ENABLE next line of code #def totalMSquare(m):### YOUR TURN TO DEFINE/WRITE/COMPLETE function totalMSquare(m) as below, based on totalN(n) Please based on the first function, add more statements for the second function. You can copy/paste the sample program to run and see how it works to make sense first. 2. Don't delete the statements/codes/comments for this program, but just add more statements for the second function. (if deleted, points will be deducted.)arrow_forwardJava Calculating the User's Sum Write a complete program that reads in numbers and calculates the sum of the numbers in that range. Code Specifications In the main method, read input from the user. Read in two integers from the user: a lower end of the range and an upper end of the range. Check if the numbers are valid: the lower number cannot be greater than the upper number. If the numbers are invalid, use a loop to ask for new numbers. Continue looping until you get two valid values. Write a method called calculateTheSum. The method takes in a lower and upper end of the range. The method calculates the sum of all values from lower (inclusive) to upper (inclusive). Invoke the method from main the output the result. Test Cases I recommend testing your code using the test cases below. I've listed the user inputs along with a sample of the result. User Inputs Result lower = 10, upper = 1 the program should ask for new input lower = 5, upper = -5 the program should ask…arrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward
- ShowCurrentTime.java an example program in Chapter, gives a program that displays the current time in GMT. Common old news shows would show clocks across many time zones, write a program that modifies ShowCurrent Time and will display the time in prominent business centers. London, Paris, New York was common. Write a program that displays the time in: Berlin, London, New York, Chicago, San Francisco, Tokyo and Hong Kong. ↓ HONG KONG ↓ LONDON L PARIS TOKYO MOSCOW 101 BERLIN DUBAI iStock Credit: Tetlana Muslyaka NEW YORKarrow_forwardSee the sample outputs for more clarification. Project Specifications Input for this project: Values of the grid (row by row) Output for this project: Whether or not the grid is magic square Programmer's full name Project number Project due date Processing Requirements Use the following template to start your project: #include using namespace std; // Global constants // The number of rows in the array // The number of columns in the array // The value of the smallest number // The value of the largest number const int ROWS = 3; const int COLS = 3; const int MIN = 1; const int MAX = 9; // Function prototypes bool isMagicsquare(int arrayRow1[], int arrayRow2 [], int arrayRow3[], int size); bool checkRange (int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max); bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size); bool checkRowSum (int arrayrow1[], int arrayrow2[], int arrayrow3[], int size); bool checkColiSum(int arrayrow1[], int…arrow_forwardDiscrete Mathematics: Assignment details: Replace all the 0 (Zero) digits in your ID by 4. Example: If your ID is 38104680, it becomes 38144684 Take the first 6 digits and substitute them in this expression (( A + B) / C) * ((D-E)/F)-2) according to the following table; Letter Replace by Digit Example Digit A 1st 3 B 2nd 8 C 3rd 1 D 4th 4 E 5th 4 F 6th 6 After substitution your expression will be similar to this (( 3 + 8) / 1) * ((4-4)/6)-2). Draw a rooted tree that represents your expression. What is the prefix form of this expression. 3.What is the value of the prefix expression obtained in step 2 above?arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
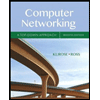
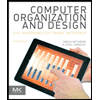
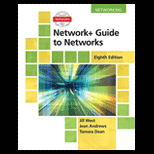
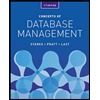
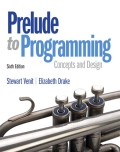
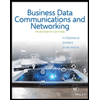