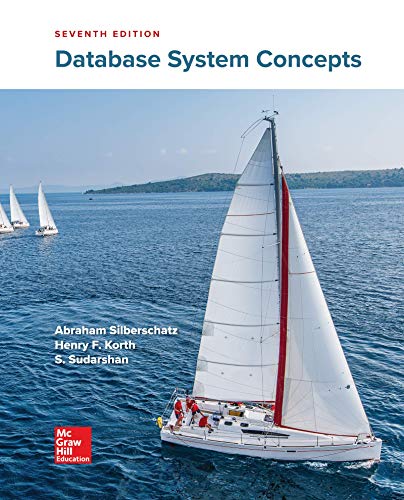
Concept explainers
in c++
Define another new class named “Item” that must inherit from the given Name
class. This Item class will manage the following info: name (string) and price
(double). It must prevent the creation of an Item object with either an empty or
all-blank name.
This class must at least complete the methods defined in the Name class:
toString method returns a string representation of the Item object in the format of
“ITEM( <name> ) PRICE($ <price> )
such as
ITEM(eraser) PRICE($1.05)
contains method that accepts a search string and returns true if only the name of
the item contains the search string as a substring. It returns false otherwise.
isTheSame method that compares two Item objects and returns true only if both
Item objects have the exact same name (regardless of the price) and false
otherwise. Please note that if the given object in the parameter is not an Item
object, it will return false.

Step by stepSolved in 3 steps with 1 images

- C++ Define an "Expression" class that manages expression info: operand1 (integer), operand2 (integer), and the expression operator (char). It must provide at least the following method:- toString() to return a string containing all the expression info such as 50 + 50 = 100 Write a command-driven program named "ListExpressions" that will accept the following commands:add, listall, listbyoperator, listsummary, exit "add" command will read the expression and save it away in the list "listall" command will display all the expressions "listbyoperator" command will read in the operator and display only expressions with that given operator "listsummary" command will display the total number of expressions, the number of expressions for each operator, the largest and smallest expression values "exit" command will exit the program Requirements:- It must be using the array of pointers (not vector) to Expression objects to manage the list of Expression…arrow_forwardC++ Create a class named Student that has three member variables:name – A string that stores the name of the studentnumClasses – An integer that tracks how many courses the student is currently enrolledinclassList – A dynamic array of strings used to store the names of the classes that thestudent is enrolled inWrite appropriate constructor(s), mutator, and accessor methods for the class along with thefollowing: A method that inputs all values from the user, including the list of class names. Thismethod will have to support input for an arbitrary number of classes. A method that outputs the name and list of all courses. A method that resets the number of classes to 0 and the classList to an empty list. An overloaded assignment operator that correctly makes a new copy of the list ofcourses. A destructor that releases all memory that has been allocated.Write a main method that tests all of your functions.arrow_forward2. The MyInteger Class Problem Description: Design a class named MyInteger. The class contains: n [] [] A private int data field named value that stores the int value represented by this object. A constructor that creates a MyInteger object for the specified int value. A get method that returns the int value. Methods isEven () and isOdd () that return true if the value is even or odd respectively. Static methods isEven (int) and isOdd (int) that return true if the specified value is even or odd respectively. Static methods isEven (MyInteger) and isOdd (MyInteger) that return true if the specified value is even or odd respectively. Methods equals (int) and equals (MyInteger) that return true if the value in the object is equal to the specified value. Implement the class. Write a client program that tests all methods in the class.arrow_forward
- Make Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardin c++ Define a class named “Variable” that manages a variable name (string)and a description (string). It should not provide the default constructor.The class must at least provide the following methods:- toString() method that can return a string in the following format: VAR(<var-name>) DESC(<var-desc>) such as VAR(isSuccessful) DESC(successful flag indicator)- contains() that accepts a search string and it will return true if its variablename or the description contains that string (case-insensitive) and false otherwise.Show how this class being used to create objects and call all its methods and showhow they work.arrow_forward
- C++ Define an "Expression" class that manages expression info: operand1 (integer), operand2 (integer), and the expression operator (char). It must provide at least the following method:- toString() to return a string containing all the expression info such as 50 + 50 = 100 Write a command-driven program named "ListExpressions" that will accept the following commands:add, listall, listbyoperator, listsummary, exit "add" command will read the expression and save it away in the list "listall" command will display all the expressions "listbyoperator" command will read in the operator and display only expressions with that given operator "listsummary" command will display the total number of expressions, the number of expressions for each operator, the largest and smallest expression values "exit" command will exit the program Requirements:- It must be using the array of pointers (not vector) to Expression objects to manage the list of Expression…arrow_forwardComputer programmingarrow_forwardIN C++ Lab #6: Shapes Create a class named Point. private attributes x and y of integer type. Create a class named Shape. private attributes: Point points[6] int howManyPoints; Create a Main Menu: Add a Triangle shape Add a Rectangle shape Add a Pentagon shape Add a Hexagon shape Exit All class functions should be well defined in the scope of this lab. Use operator overloading for the array in Shape class. Once you ask the points of any shape it will display in the terminal the points added.arrow_forward
- 6. Patient Charges Write a class named Patient that has attributes for the following data: • First name, middle name, and last name • Address, city, state, and ZIP code • Phone number ● The Patient class's init__ method should accept an argument for each attribute. The Name and phone number of emergency contact Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: • Name of the procedure • Date of the procedure • Name of the practitioner who performed the procedure • Charges for the procedure 107 The Procedure class's __init__ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three…arrow_forwardThe class definition for an Item is given in the image UML diagram below Note: getInfo() returns a string containing ALL of the state information neatly organized. Part 1: Create a class based on the specification above. Reminder: – means private and + means public. Part 2: a)Write a function/method called “addItem” that takes: An array of items The number of items in the array An integer representing a barcode A string representing the name of an item A string representing the description of an item A double value representing the price The function must create and add the item to the array if there is space. The function must return “true” if the addition was successful and “false” otherwise. b)Write a function/method called “listItems” that takes: An array of items The number of items in the array The function must return a string containing the information on each item in the array. c)Write a function called “pricelookup” that takes : An array of items The number of…arrow_forwardMake Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
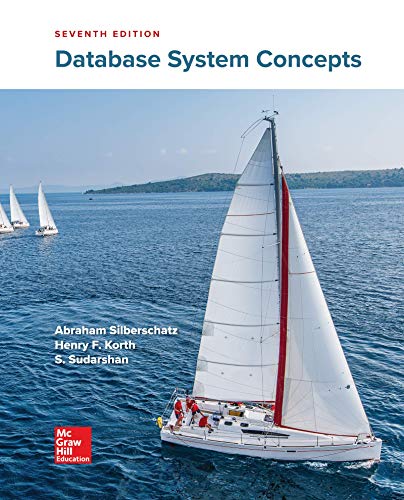
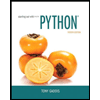
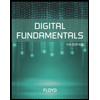
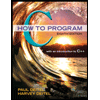
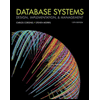
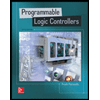