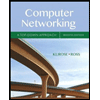
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
In C program. implement list_find( list_t* list, int targetElement ) function
/*
* dataStructure.h
*
* Provides a data structure made of a doubly-headed singly-linked list (DHSL)
* of unsorted elements (integers) in which duplicated elements are allowed.
*
* A DHSL list is comprised of a single header and zero or more elements.
* The header contains pointers to the first and last elements in the list,
* or NULL if the list is empty. The first element contains a pointer to
* the next element, and so on. The last element in the list has its
* "next" pointer set to NULL.
*
* The interface of this data structure includes several utility
* functions that operate on this data structure.
*
* Precondition: Functions that operate on such data structure
* require a valid pointer to it as their first argument.
*
* Do not change this dataStructure.h file.
*
*/
/*** Data structure ***/
/* List element (node): our DHSL list is a chain of these nodes. */
typedef struct element {
int val;
structelement*next;
} element_t;
/* List header: keeps track of the first and last list elements
as well as the number of elements stored in our data structure. */
typedef struct list {
element_t* head;
element_t* tail;
unsignedint elementCount;
} list_t;
/*** Data structure interface ***/
/* A type for returning status codes */
typedef enum {
OK,
ERROR,
NULL_PARAM
} result_t;
//dataStructure.c
/* Description: Returns a pointer to the element (i.e., to the node) that
* contains the first occurrence of "targetElement" in the data
* structure pointed to by "list". Returns NULL if "targetElement"
* is not found. Returns NULL if "list" is NULL.
* This function does not modify the data structure.
* Time efficiency: O(n)
*/
element_t* list_find( list_t* list, int targetElement ) {
element_t* anElement = NULL;
// Stubbing this function
// This stub is to be removed when you have successfully implemented this function, i.e.,
// ***Remove this call to printf!***
printf( "Calling list_find(...): find the first occurrence of targetElement %d in the list.\n", targetElement );
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- C++ Create a generic function add_bookends(ls, e) that adds a copy of element e to the front and back of list ls.arrow_forwardConsider the following function access_element_by_index that returns the iterator to the element at the index i in the list l. typedef std::list<int> int_list; int_list::iterator access_element_by_index(size_t I, int_list &) { assert( ? ); … } Assume the first element of the list is at the index 0, the second at the index 1, and so on. If the function is required to return an iterator that can be dereferenced, determine the precondition of the function, and write an assertion to validate it. (You don’t need to implement the functions).arrow_forwardWrite a C code that will search an int type target from a dynamic linked list. The linked list contains int type values inside each of the nodes. The “search” function will use following input-output arguments,Input: Pointer to the head of the list that you are searching Target value that you are searchingOutput: Pointer to the node that contains the target if found or, NULL if not found.arrow_forward
- In Python: Create a function Matrix_transpose() utilizing List comprehension techniques that takes a 2d list containing user generated/input elements of type int as its argument then returns the reference to a new 2d list that is the transpose of the original. Assume the matrix is rectangular. Show the funtion works with different values. Do not use any python packages to create the funtion.arrow_forwardGiven an IntNode struct and the operating functions for a linked list, complete the following functions to extend the functionality of the linked list(this is not graded).arrow_forwardPascal triangle! write a function(in OCaml) val nextpascalrow : int list -> int list = that given a list of integers that correspond to a row of the pascal triangle, it calculates the next row of the pascal triangle. # nextpascalrow [1; 6; 15; 20; 15; 6; 1];; - : int list = [1; 7; 21; 35; 35; 21; 7; 1]arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
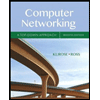
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
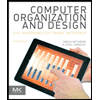
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
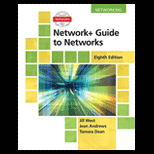
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
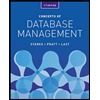
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
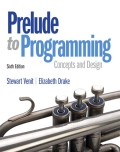
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
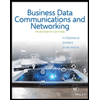
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY