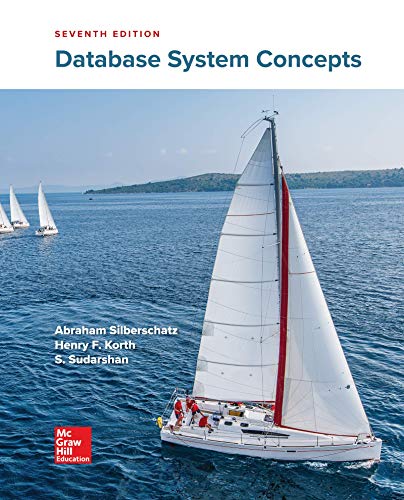
In Data Structures, you studied binary heaps. Binary heaps support the insert and
extractMin
functions in O(lgn), and getMin in O(1). Moreover, you can build a heap of n elements in
just O(n). Refresh your knowledge of heaps from chapter no. 6 of your algorithms text
book.
Now implement Merge Sort, Heap Sort, and Quick Sort in C++ and perform the following
experiment:
1. Generate an Array A of 107 random numbers. Make its copies B and C. Sort A using
Merge Sort, B using Heap Sort, and C using Quick Sort.
2. During the sorting process, count the total number of comparisons between array
elements made by each
to
function to compare numbers, which increments a count variable each time it is called.
3. Repeat this process 5 times to compute the average number of comparisons made by
each algorithm.
4. Present these average counts in a table. These counts give you an indication of how the
different algorithms compare asymptotically (in big-O terms) for a large value of n.
(b) Now compare the same algorithms in terms of practical time, i.e. the actual running time.
Simply, repeat the previous example but use the chrono library to compute the actual times
taken by each algorithm, and report the average value of the time for each algorithm.

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 1 images

- USING C++, Implement a TEMPLATED Binary Search Tree (RECURSIVE) class, that is minimal & complete. Provide the following recursive operations: Insert, Delete tree, Search, inOrder traversal, preOrder traversal, postOrder traversal. Methods essential for correct operation of tree in terms of managing memory must be provided. Any additional methods can be added. Use function pointers in the traversal routines. cannot use std::function (or any other similar routine from std namespace. Test BST to make sure all methods work. In particular make sure that you can pass the tree to other routines by value, const reference and reference. Check that data values are as expected on pass/return to/from routines. Do not use GUI interaction. Input/Output (user): Sample output formats shown below use made up data for the year 1905. Menu options are: The average wind speed and sample standard deviation for this wind speed given a specified month and year. (print on screen only) Eg: January…arrow_forwardImplement the pre-order tree traversal algorithm in C++. This program should use the following binary tree (see image) and display the pre-order tree traversal of the nodes’ values. Feel free to write this iteratively or recursively. Also, explain how it works and how a new tree could be provided to the code. PS: make sure that the logic of the program is correct, meaning that it can work well with different binary trees. I would like to change the tree input to the pre-order traversal function. The program should work errorless and display the result correctly. No need to code it to read the user input, however, provide instructions on how a new tree can be defined in this program to check if the program works fine with different trees.arrow_forwardWrite a java class named First_Last_Recursive_Merge_Sort that implements the recursive algorithm for Merge Sort. You can use the structure below for your implementation. public class First_Last_Recursive_Merge_Sort { //This can be used to test your implementation. public static void main(String[] args) { final String[] items = {"Zeke", "Bob", "Ali", "John", "Jody", "Jamie", "Bill", "Rob", "Zeke", "Clayton"}; display(items, items.length - 1); mergeSort(items, 0, items.length - 1); display(items, items.length - 1); } private static <T extends Comparable<? super T>> void mergeSort(T[] a, int first, int last) { //<Your implementation of the recursive algorithm for Merge Sort should go here> } // end mergeSort private static <T extends Comparable<? super T>> void merge(T[] a, T[] tempArray, int first, int mid, int last) { //<Your implementation of the merge algorithm should go here> } // end merge //Just a quick method to display the whole array. public…arrow_forward
- Please help me with this Principles of programming language homework questionarrow_forwardCan you implement bucket sort algorithm in c++ for me and run a test program for it.arrow_forwardJava Quick Sort but make it read the data 10, 7, 8, 9, 1, 5 from a file not an array // Java implementation of QuickSort import java.io.*; class GFG { // A utility function to swap two elements static void swap(int[] arr, int i, int j) { int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } /* This function takes last element as pivot, places the pivot element at its correct position in sorted array, and places all smaller (smaller than pivot) to left of pivot and all greater elements to right of pivot */ static int partition(int[] arr, int low, int high) { // pivot int pivot = arr[high]; // Index of smaller element and // indicates the right position // of pivot found so far int i = (low - 1); for (int j = low; j <= high - 1; j++) { // If current element is smaller // than the pivot if…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
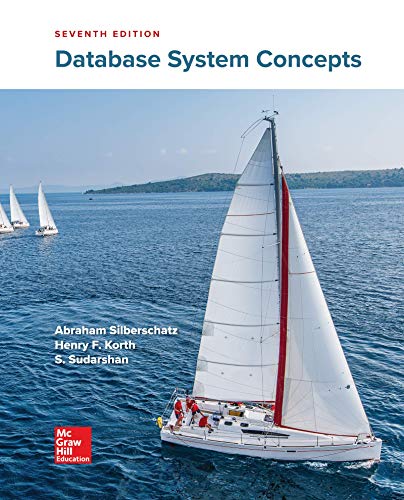
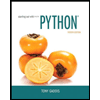
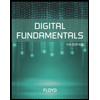
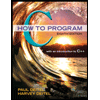
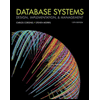
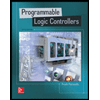