Please help solve this Java problem. Source code for MessageEncoder.java and MessageDecoder.Java is below. Filename: MessageEncoder.java public interface MessageEncoder { /** * The method that encodes text * @param plainText the text to encode * @return the encoded text */ public abstract String encode(String plainText); } Filename: MessageDecoder.Java public interface MessageDecoder { /** * This method will decode the cypher text * @param cipherText the encoded message * @return the decoded text */ public abstract String decode(String cipherText); }
Please help solve this Java problem. Source code for MessageEncoder.java and MessageDecoder.Java is below. Filename: MessageEncoder.java public interface MessageEncoder { /** * The method that encodes text * @param plainText the text to encode * @return the encoded text */ public abstract String encode(String plainText); } Filename: MessageDecoder.Java public interface MessageDecoder { /** * This method will decode the cypher text * @param cipherText the encoded message * @return the decoded text */ public abstract String decode(String cipherText); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Please help solve this Java problem. Source code for MessageEncoder.java and MessageDecoder.Java is below.
Filename: MessageEncoder.java
public interface MessageEncoder {
/**
* The method that encodes text
* @param plainText the text to encode
* @return the encoded text
*/
public abstract String encode(String plainText);
}
Filename: MessageDecoder.Java
public interface MessageDecoder {
/**
* This method will decode the cypher text
* @param cipherText the encoded message
* @return the decoded text
*/
public abstract String decode(String cipherText);
}
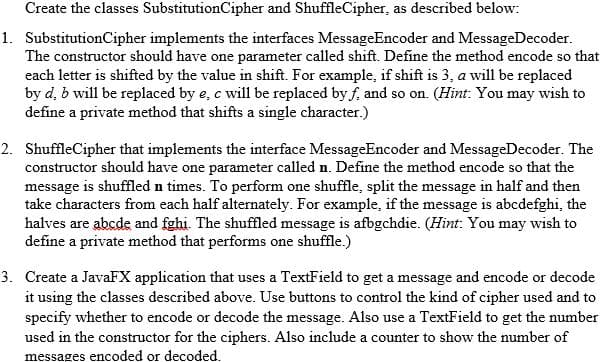
Transcribed Image Text:Create the classes SubstitutionCipher and ShuffleCipher, as described below:
1. SubstitutionCipher implements the interfaces MessageEncoder and MessageDecoder.
The constructor should have one parameter called shift. Define the method encode so that
each letter is shifted by the value in shift. For example, if shift is 3, a will be replaced
by d, b will be replaced by e, c will be replaced by f, and so on. (Hint: You may wish to
define a private method that shifts a single character.)
2. ShuffleCipher that implements the interface MessageEncoder and MessageDecoder. The
constructor should have one parameter called n. Define the method encode so that the
message is shuffled n times. To perform one shuffle, split the message in half and then
take characters from each half alternately. For example, if the message is abcdefghi, the
halves are abcde and fghi. The shuffled message is afbgchdie. (Hint: You may wish to
define a private method that performs one shuffle.)
3. Create a JavaFX application that uses a TextField to get a message and encode or decode
it using the classes described above. Use buttons to control the kind of cipher used and to
specify whether to encode or decode the message. Also use a TextField to get the number
used in the constructor for the ciphers. Also include a counter to show the number of
messages encoded or decoded.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
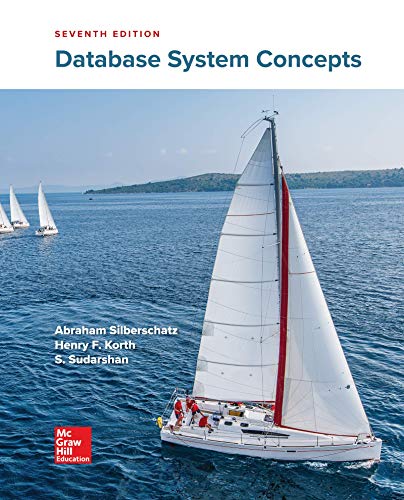
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
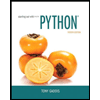
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
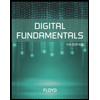
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
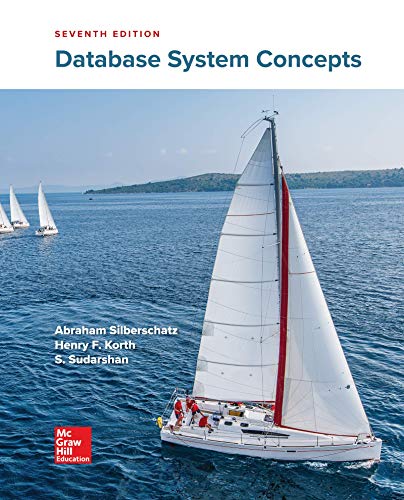
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
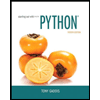
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
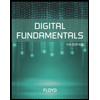
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
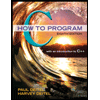
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
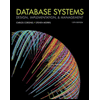
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
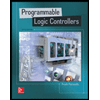
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education