In Java: Write a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, and output all integers less than or equal to that value. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 50 60 140 200 75 100 the output is: 50 60 75 The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. For coding simplicity, follow every output value by a space, including the last one. Such functionality is common on sites like Amazon, where a user can filter results. Write your code to define and use two methods: public static void getUserValues(int[] myArr, int arrSize, Scanner scnr) public static void outputIntsLessThanOrEqualToThreshold(int[] userValues, int userValsSize, int upperThreshold) Utilizing methods will help to make main() very clean and intuitive. import java.util.Scanner; public class LabProgram { /* Define your methods here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int[] userValues = new int[20]; int upperThreshold; int numVals; numVals = scnr.nextInt(); getUserValues(userValues, numVals, scnr); upperThreshold = scnr.nextInt(); outputIntsLessThanOrEqualToThreshold(userValues, numVals, upperThreshold);
In Java:
Write a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, and output all integers less than or equal to that value. Assume that the list will always contain less than 20 integers.
Ex: If the input is:
5 50 60 140 200 75 100
the output is:
50 60 75
The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. For coding simplicity, follow every output value by a space, including the last one.
Such functionality is common on sites like Amazon, where a user can filter results.
Write your code to define and use two methods:
public static void getUserValues(int[] myArr, int arrSize, Scanner scnr)
public static void outputIntsLessThanOrEqualToThreshold(int[] userValues, int userValsSize, int upperThreshold)
Utilizing methods will help to make main() very clean and intuitive.
import java.util.Scanner;
public class LabProgram {
/* Define your methods here */
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int[] userValues = new int[20];
int upperThreshold;
int numVals;
numVals = scnr.nextInt();
getUserValues(userValues, numVals, scnr);
upperThreshold = scnr.nextInt();
outputIntsLessThanOrEqualToThreshold(userValues, numVals, upperThreshold);

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

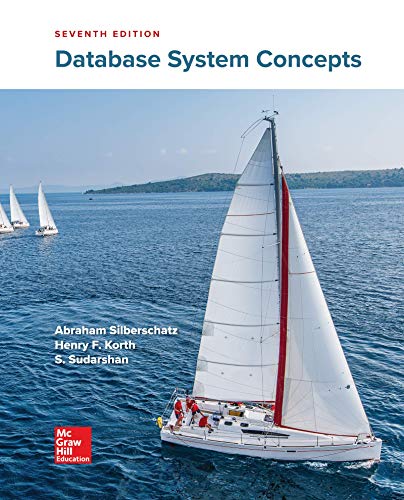
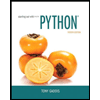
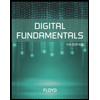
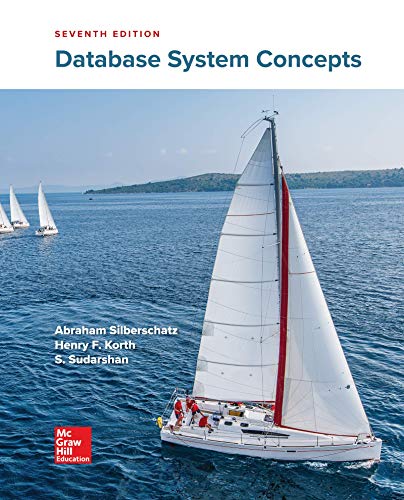
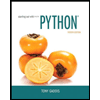
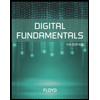
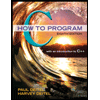
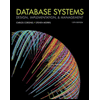
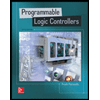