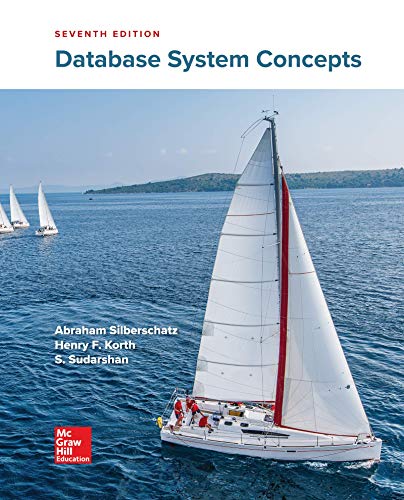
In JAVA
Write a
program runs, a loop should run 5 times; in each iteration, the program should
• Generate a random integer in the range of 1 to 6 --this is the value of the computer’s die.
• Generate another random integer in the range of 1 to 6 --this is the value of the user’s die.
The die with the highest value wins. (In case of a tie, there is no winner for that particular roll of
the dice.) As the loop iterates, the program should count the number of times the computer wins
and the number of times the user wins. In the end, the program should display who was the grand
winner, the computer, or the user. The program should perform input validation for the player’s
input (only ‘y’ or ‘n’ are acceptable).

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- in Java Tasks Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don't display the computer's choice yet.) 2. The user enters his or her choice of "rock", "paper", or "scissors" at the keyboard. (You can use a menu if you prefer.) 3. The computer's choice is displayed. Tasks 4. A winner is selected according to the following rules: a. If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) b. If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) C. If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps…arrow_forwardJava Programming: Write a command line game that plays a simple version of blackjack. The program should generate random numbers between 1 and 10 each time the player gets a card. It should keep a running total of the players cards, and ask the player whether or not it should deal another card. Sample output for the game is written below. Your program should produce the same output. The players get two cards to start with. Then they are asked if they want more cards. Players can continue to take as many cards as they like. The goal is to get close to 21 without going over. If the total is greater than 21 we say the player "busted".arrow_forwardIn Javaarrow_forward
- Using a Sentinel Value to Control a while Loop Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the average…arrow_forwardCode in C++ Language As a cashier, you would always hate those times when customers choose to take back their order since you'd always summon your manager and yell, "Ma'am pa-void!" No matter how you hate it, you always have to do it since you have no other choice. Given (1) an array of prices of the items, (2) another array of the items the customer wishes to take back, and (3) the customer's payment, print the change of the customer. Input: The first number indicates the number of items "n" brought to the cashier. The next "n" numbers are the prices of the items. The next number indicates the number of items "m" that the customer wants to take back and not proceed. It is assured that 0 <= m <= n. The next "m" numbers are the items to be void that ranges from item 1 to item "n". The final number is the customer's payment. It is also assured that the given payment >= final price of items. INPUT: 5 103.65 650.95 10.25 1067.30 65.18 2 1 5 2000.00 Output: The change…arrow_forwardUsing a Sentinel Value to Control a while Loop in Java Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the…arrow_forward
- Accumulating Totals in a Loop Summary In this lab, you add a loop and the statements that make up the loop body to a Java program that is provided. When completed, the program should calculate two totals: the number of left-handed people and the number of right-handed people in your class. Your loop should execute until the user enters the character X instead of L for left-handed or R for right-handed. The inputs for this program are as follows: R, R, R, L, L, L, R, L, R, R, L, X Variables have been declared for you, and the input and output statements have been written. Instructions Ensure the file named LeftOrRight.java is open. Write a loop and a loop body that allows you to calculate a total of left-handed and right-handed people in your class. Execute the program by clicking Run and using the data listed above and verify that the output is correct.arrow_forwardJava Programming: Write a program that reads five integer values from the user, then analyzes them as if they were a hand of cards. When your program runs it might look like this (user input is in orange for clarity): Enter five numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card 3: 8 Card 4: 2 Card 5: 9 Pair! (This is a pair, since there are two eights). You are only required to find the hands Pair and Straight.arrow_forwardPrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forward
- In java, create a program that will use a do while loop with a "yes/no" prompt. The program should include user input and output with getters and setters. Once the end of the loop is reached, ask the user if they'd like to do the program again. Answering "Yes" will start the program over again. Answering "No" will end the program. Answering with anything else will be an invalid input. Once the loop begins again, the program should also discard all previous inputs so that there won't be any overlapping. Use Scanner.nextLine();, it will help.arrow_forwardJava Calculating the User's Sum Write a complete program that reads in numbers and calculates the sum of the numbers in that range. Code Specifications In the main method, read input from the user. Read in two integers from the user: a lower end of the range and an upper end of the range. Check if the numbers are valid: the lower number cannot be greater than the upper number. If the numbers are invalid, use a loop to ask for new numbers. Continue looping until you get two valid values. Write a method called calculateTheSum. The method takes in a lower and upper end of the range. The method calculates the sum of all values from lower (inclusive) to upper (inclusive). Invoke the method from main the output the result. Test Cases I recommend testing your code using the test cases below. I've listed the user inputs along with a sample of the result. User Inputs Result lower = 10, upper = 1 the program should ask for new input lower = 5, upper = -5 the program should ask…arrow_forwardin Java languagearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
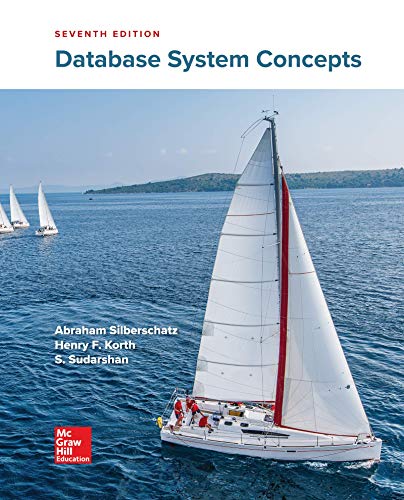
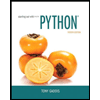
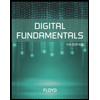
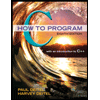
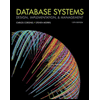
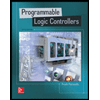