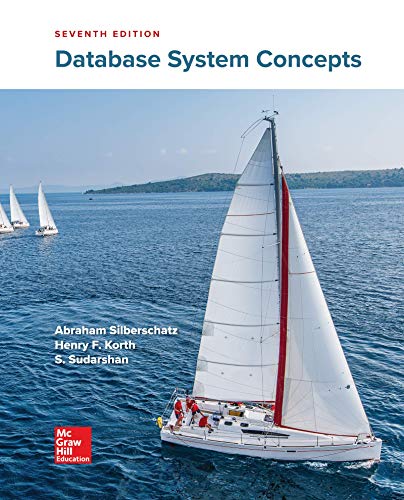
Concept explainers
In Python
accept input representing a 10-digit phone number and generate an output with
- the area code, sourrounded by parentheses, then a space,
- then the prefix, and
- line number, separated by hyphens.
For this lab, you will use the following functions to accomplish the same formatted output, given the same 10-digit input:
get_area_code(ten_digit_number) -- which will return the area code only, no space or parentheses
get_prefix(ten_digit_number) -- which will return the prefix only, no space or hyphen
get_line_number(ten_digit_number) -- which will return the line number only, no space or hyphen
Example of the
The following program reads 10 digits as a phone number and generates a formatted output. For example if the user enters: 8772692967, then the output will be: (877) 269-2967
Please enter 10 digits: 8005551212
Here is your formatted phone number: (800) 555-1212
# Define your functions here
3.if __name__ == '__main__':
4. print("The following program reads 10 digits as a phone number and 5. generates a formatted output.")
6. print("For example if the user enters: 8772692967, then the output will be: ", end=" ")
7. print("(877) 269-2967")
8. print()
9. phone_number = input("Please enter 10 digits: ")
#Type your code here. Your code must call the functions.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Python Write a function called count_dashes that takes a line of text as inputand outputs the number of dashes in it, as shown below. In addition to printing the requested information, return the number of dashes. The function should output (print) its findings as follows:There are DDD dashes in 'XXX'.where XXX and DDD are replaced by the appropriate values that are based on the given argument.arrow_forwardIn computer animation, a "jiffy" is commonly defined as 1/100th of a second. Define a function named jiffies_to_seconds that takes the number of "jiffies" as a parameter, and returns the number of seconds. Then, write a main program that reads the number of jiffies (float) as an input, calls function jiffies_to_seconds() with the input as argument, and outputs the number of seconds. Output each floating-point value with three digits after the decimal point, which can be achieved as follows: print (f'{your_value:.3f}') Ex: If the input is: 15.25 the output is: 0.152 The program must define and call the following function: def jiffies_to_seconds (user_jiffies) 461710.3116374.qx3zqy7 LAB ACTIVITY 7.11.1: LAB: A jiffy 1 # Define your function here 12345 3 if __name__ main.py == '__main__': # Type your code here. Your code must call the function. 0/10 Load default template...arrow_forwardA palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward, disregarding spaces, punctuation, and capitalization. Write a function isPalindrome that takes in a string s and returns a boolean value indicating whether s is a palindrome. Example: Input: s = "racecar" Output: true Input: s = "Hello" Output: false Input: s = "A man, a plan, a canal, Panama" Output: true Input: s = "12321" Output: true Note: The input string may contain spaces, punctuation, and capitalization, but they should be disregarded while checking for palindromes. An empty string is considered a palindrome.arrow_forward
- Flowchart, create. Writing Functions that Require a Single Parameter in Python def sums(n): s = 0 for i in range(1, n + 1): s += i print("Sum is:", s) def products(n): p = 1 for i in range(1, n + 1): p *= i print("Product is:", p) numberString = input("Enter a positive integer or 0 to quit: ") number = int(numberString) while number != 0: sums(number) products(number) numberString = input("Enter a positive integer or 0 to quit: ") number = int(numberString)arrow_forwardPlease do it in python I have asked this question before but i need it in try and except You have been asked to take a small icon that appears on the screen of a smart telephone and scale it up so it looks bigger on a regular computer screen. The icon will be encoded as characters (x and *) in a 3 x 3 grid as follows: Write a program that accepts a positive integer scaling factor and outputs the scaled icon. A scaling factor of k means that each character is replaced by a k ? k grid consisting only of that character. Input Specification: (Use a try and except here)The input will be an integer such that 0 < k ≤ 10. Output Specification:The output will be 3k lines, which represent each individual line scaled by a factor of k and repeated k times. A line is scaled by a factor of k by replacing each character in the line with k copies of the character. Use try and exceptarrow_forwardIn Java: Forms often allow a user to enter an integer. Write a program that takes in a string representing an integer as input, and outputs yes if every character is a digit 0-9. Ex: If the input is: 1995 the output is: yes Ex: If the input is: 42,000 or 1995! the output is: no Hint: Use a loop and the Character.isDigit() function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
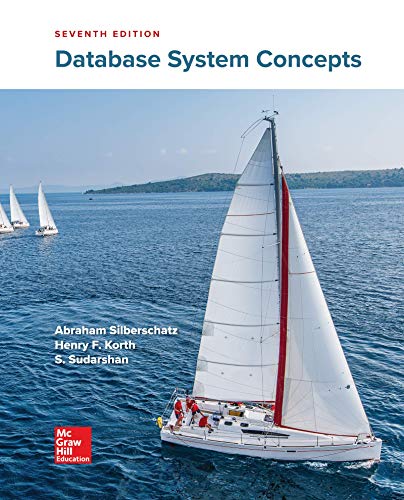
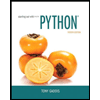
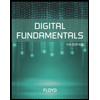
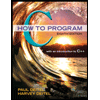
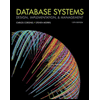
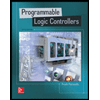