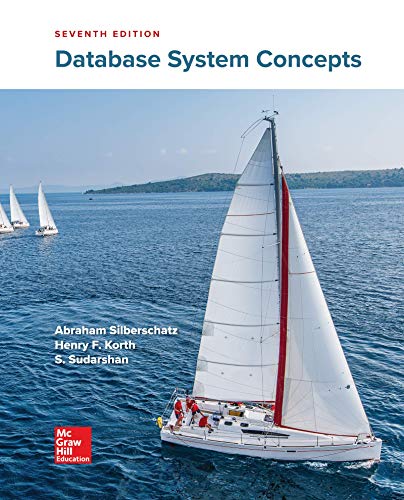
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
// JumpinJava.cpp - This program looks up and prints the names and prices of coffee orders.
// Input: Interactive
// Output: Name and price of coffee orders or error message if add-in is not found
#include <iostream>
#include <string>
using namespace std;
int main()
{
// Declare variables.
string addIn; // Add-in ordered
const int NUM_ITEMS = 5; // Named constant
// Initialized array of add-ins
string addIns[] = {"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"};
// Initialized array of add-in prices
double addInPrices[] = {.89, .25, .59, 1.50, 1.75};
bool foundIt = false; // Flag variable
int x; // Loop control variable
double orderTotal = 2.00; // All orders start with a 2.00 charge
// Get user input
cout << "Enter coffee add-in or XXX to quit: ";
cin >> addIn;
// Write the rest of the program here.
return 0;
} // End of main()
![ays
JumpinJive.cpP
1 // JumpinJava.cpp - This program looks up and prints the n
Summary
2 // Input:
Interactive
3//Output: Name and price of coffee orders or error messag
4.
In this lab, you use what you have learned about parallel
5. #include <iostream>
</>
arrays to complete a partially completed C++ program.
6 #include <string>
The program should:
7 using namespace std;
8)
• Either print the name and price for a coffee add-in
9 int main()
10 {
from the Jumpin' Jive Coffee Shop
|11
/ Declare variables
• Or it should print the message
12
string addIn;
/ Add-in ordered
13
const int NUM ITEMS
5; // Named constant
Sorry, we do not carry that.
14
WInitialized array of add-ins
string addIns[]
//Initiaized array of add-in prices
15
{"Cream", "Cinnamon", "Chocolate", "Amar
Read the problem description carefully before you
16
17
double addInPrices[]
{.89, .25, . 59, 1.50, 1.75};
/ Flag variable
begin. The file provided for this lab includes the
18
bool foundIt = false;
necessary variable declarations and input statements.
19
int x
//Loop control variable
double orderTotal = 2.00; // All orders start with a 2.00 ch
You need to write the part of the program that searches
20
21
22
for the name of the coffee add-in(s) and either prints
the name and price of the add-in or prints the error
// Get user input
23
cout << "Enter coffee add-in or XXX to quit:
message if the add-in is not found. Comments in the
24
cin >> addIn;
code tell you where to write your statements.
25
26
7/ Write the rest of the program here](https://content.bartleby.com/qna-images/question/4bfbb203-4399-4804-a6b8-bbfebafe4401/145b10d7-ac08-46b7-848c-c38e56c4a409/nnbm8i_thumbnail.jpeg)
Transcribed Image Text:ays
JumpinJive.cpP
1 // JumpinJava.cpp - This program looks up and prints the n
Summary
2 // Input:
Interactive
3//Output: Name and price of coffee orders or error messag
4.
In this lab, you use what you have learned about parallel
5. #include <iostream>
</>
arrays to complete a partially completed C++ program.
6 #include <string>
The program should:
7 using namespace std;
8)
• Either print the name and price for a coffee add-in
9 int main()
10 {
from the Jumpin' Jive Coffee Shop
|11
/ Declare variables
• Or it should print the message
12
string addIn;
/ Add-in ordered
13
const int NUM ITEMS
5; // Named constant
Sorry, we do not carry that.
14
WInitialized array of add-ins
string addIns[]
//Initiaized array of add-in prices
15
{"Cream", "Cinnamon", "Chocolate", "Amar
Read the problem description carefully before you
16
17
double addInPrices[]
{.89, .25, . 59, 1.50, 1.75};
/ Flag variable
begin. The file provided for this lab includes the
18
bool foundIt = false;
necessary variable declarations and input statements.
19
int x
//Loop control variable
double orderTotal = 2.00; // All orders start with a 2.00 ch
You need to write the part of the program that searches
20
21
22
for the name of the coffee add-in(s) and either prints
the name and price of the add-in or prints the error
// Get user input
23
cout << "Enter coffee add-in or XXX to quit:
message if the add-in is not found. Comments in the
24
cin >> addIn;
code tell you where to write your statements.
25
26
7/ Write the rest of the program here
![Parallel Arrays
JumpinJive.cpp
Co a n you wilele to wite your ɔlateiiemLS.
1 // JumpinJava.cpp
21/ Input:
3 // Output: Name and price of coffee orders or error message if add-in is no
This program looks up and prints the names and prices a
dy Tools
Interactive
Instructions
4.
5 #include <iostream>
1. Study the prewritten code to make sure you
6 #include <string>
understand it.
7 using namespace std;
5 Tips
8.
2. Write the code that searches the array for the name
9 int mainO
Tips
of the add-in ordered by the customer.
10 {
11
// Declare variables.
3. Write the code that prints the name and price of the
12
string addIn;
const int NUM ITEMS = 5; // Named constant
no.
W Add-in ordered
add-in or the error message, and then write the
13
code that prints the cost of the total order.
14
// Initialized array of add-ins
string addIns[]
V/ Initialized array of add-in prices
15
{"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"};
4. Execute the program by clicking the Run button at
16
17
double addInPrices[]
bool foundIt = false;
the bottom of the screen. Use the following data:
= {,89, .25, .59, 1.50, 1.75};
18
// Flag variable
// Loop control variable
boxes
Cream
19
int X;
20
double orderTotal
2.00; // All orders start with a 2.00 charge
21
Caramel
22
// Get user input
Whiskey
23
cout << "Enter coffee add-in or XXX to quit: ";
ck
24
cin >> addIn;
chocolate
25
26
// Write the rest of the program here.
Chocolate
27
return O;
28 }// End of main()
Cinnamon
29
Vanilla
!!](https://content.bartleby.com/qna-images/question/4bfbb203-4399-4804-a6b8-bbfebafe4401/145b10d7-ac08-46b7-848c-c38e56c4a409/suztw3d_thumbnail.jpeg)
Transcribed Image Text:Parallel Arrays
JumpinJive.cpp
Co a n you wilele to wite your ɔlateiiemLS.
1 // JumpinJava.cpp
21/ Input:
3 // Output: Name and price of coffee orders or error message if add-in is no
This program looks up and prints the names and prices a
dy Tools
Interactive
Instructions
4.
5 #include <iostream>
1. Study the prewritten code to make sure you
6 #include <string>
understand it.
7 using namespace std;
5 Tips
8.
2. Write the code that searches the array for the name
9 int mainO
Tips
of the add-in ordered by the customer.
10 {
11
// Declare variables.
3. Write the code that prints the name and price of the
12
string addIn;
const int NUM ITEMS = 5; // Named constant
no.
W Add-in ordered
add-in or the error message, and then write the
13
code that prints the cost of the total order.
14
// Initialized array of add-ins
string addIns[]
V/ Initialized array of add-in prices
15
{"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"};
4. Execute the program by clicking the Run button at
16
17
double addInPrices[]
bool foundIt = false;
the bottom of the screen. Use the following data:
= {,89, .25, .59, 1.50, 1.75};
18
// Flag variable
// Loop control variable
boxes
Cream
19
int X;
20
double orderTotal
2.00; // All orders start with a 2.00 charge
21
Caramel
22
// Get user input
Whiskey
23
cout << "Enter coffee add-in or XXX to quit: ";
ck
24
cin >> addIn;
chocolate
25
26
// Write the rest of the program here.
Chocolate
27
return O;
28 }// End of main()
Cinnamon
29
Vanilla
!!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- #include <iostream>#include <string>#include <climits> using namespace std;class BalancedTernary { protected: // Store the value as a reversed string of +, 0 and - characters string value; // Helper function to change a balanced ternary character to an integer int charToInt(char c) const { if (c == '0') return 0; return 44 - c; } // Helper function to negate a string of ternary characters string negate(string s) const { for (int i = 0; i < s.length(); ++i) { if (s[i] == '+') s[i] = '-'; else if (s[i] == '-') s[i] = '+'; } return s; } public: // Default constructor BalancedTernary() { value = "0"; } // Construct from a string BalancedTernary(string s) { value = string(s.rbegin(), s.rend()); } // Construct from an integer BalancedTernary(long long n) { if (n == 0) { value = "0"; return; } bool neg = n < 0; if (neg) n = -n; value = ""; while (n !=…arrow_forwardjust answer asaparrow_forwardC++ Coding extra credit activity copiable code: #include <iostream>// OTHER INCLUDES??? using namespace std; // YOUR CODE HERE #include "testcode.h" // For automatic grading purposes DO NOT REMOVE (but you can comment out temporarily for testing) int main() { testTimerClass(); // Test code for automatic grading. DO NOT REMOVE (but you can temporarily comment out for your own testing). Remove any test code you may have added prior to final submission.} Additional Files: testcode.h and testTimerClass These files will automatically be compiled with your code. You cannot modify them. testcode.h using namespace std; void testGetTime(){ Timer timer(true); system("sleep 2"); cout << timer.getTime() << std::endl;}void testStartAndStop(){ Timer timer(true); system("sleep 1"); timer.printTime(); timer.stop(); system("sleep 1"); timer.printTime(); timer.start(); system("sleep 1"); timer.printTime(); timer.stop(); system("sleep 1");…arrow_forward
- // MovieGuide.cpp - This program allows each theater patron to enter a value from 0 to 4 // indicating the number of stars that the patron awards to the Guide's featured movie of the // week. The program executes continuously until the theater manager enters a negative number to // quit. At the end of the program, the average star rating for the movie is displayed. #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. double numStars; // star rating. double averageStars; // average star rating. double totalStars = 0; // total of star ratings. int numPatrons = 0; // keep track of number of patrons // This is the work done in the housekeeping() function // Get input. cout << "Enter rating for featured movie: "; cin >> numStars; // This is the work done in the detailLoop() function // Write while loop here //…arrow_forwardQuestion 1: break phrase Problem statement Breaking a string into two parts based on a delimiter has applications. For example, given an email address, breaking it based on the delimiter "@" gives you the two parts, the mail server's domain name and email username. Another example would be separating a phone number into the area code and the rest. Given a phrase of string and a delimiter string (shorter than the phrase but may be longer than length 1), write a C++ function named break_string to break the phrase into two parts and return the parts as a C++ std::pair object (left part goes to the "first" and right part goes to the "second").arrow_forwardMain.cpp #include <iostream>#include "Deck.h" int main() { Deck deck; deck.shuffle(); std::cout << "WAR Card Game\n\n"; std::cout << "Dealing cards...\n\n"; Card player1Card = deck.Deal(); Card player2Card = deck.Deal(); std::cout << "Player 1's card: "; player1Card.showCard(); std::cout << std::endl; std::cout << "Player 2's card: "; player2Card.showCard(); std::cout << std::endl; int player1Value = player1Card.getValue(); int player2Value = player2Card.getValue(); if (player1Value > player2Value) { std::cout << "Player 1 wins!" << std::endl; } else if (player1Value < player2Value) { std::cout << "Player 2 wins!" << std::endl; } else { std::cout << "It's a tie!" << std::endl; } return 0;} Card.h #ifndef CARD_H#define CARD_H class Card {public: Card(); Card(char r, char s); int getValue(); void showCard();…arrow_forward
- Background Information This assignment tests your understanding of and ability to apply the programming concepts we have covered in the unit so far, including the usage of variables, input and output, data types, selection, iteration, functions and data structures. Above all else, it tests your ability to design and then implement a solution to a problem using these concepts. Assignment Overview You are required to design and implement a "Word Game" program in which the user must identify a randomly selected "password" from a list of 8 words. The 8 words are selected at random from the list of 100 words in the starter file (word_game.py) provided to you with this assignment brief. Please use the starter file as the basis of your assignment code. The user has 4 attempts in which to guess the password. Whenever they guess incorrectly, they are told how many of the letters are the same between the word they guessed and the password. For example, if the password is "COMEDY" and…arrow_forwardC++ Programmingarrow_forwardQuestion 1: break phrase Problem statement Breaking a string into two parts based on a delimiter has applications. For example, given an email address, breaking it based on the delimiter "@" gives you the two parts, the mail server's domain name and email username. Another example would be separating a phone number into the area code and the rest. Given a phrase of string and a delimiter string (shorter than the phrase but may be longer than length 1), write a C++ function named break_string to break the phrase into two parts and return the parts as a C++ std::pair object (left part goes to the "first" and right part goes to the "second"). Do the following Write your algorithm as code comments. I recommend to follow UMPIRE technique Implement your functionarrow_forward
- 3. int count(10); while(count >= 0) { count -= 2; std::cout << count << endl;arrow_forwardMain.cpp #include <iostream>#include "Deck.h" int main() { Deck deck; deck.shuffle(); std::cout << "WAR Card Game\n\n"; std::cout << "Dealing cards...\n\n"; Card player1Card = deck.Deal(); Card player2Card = deck.Deal(); std::cout << "Player 1's card: "; player1Card.showCard(); std::cout << std::endl; std::cout << "Player 2's card: "; player2Card.showCard(); std::cout << std::endl; int player1Value = player1Card.getValue(); int player2Value = player2Card.getValue(); if (player1Value > player2Value) { std::cout << "Player 1 wins!" << std::endl; } else if (player1Value < player2Value) { std::cout << "Player 2 wins!" << std::endl; } else { std::cout << "It's a tie!" << std::endl; } return 0;} Card.h #ifndef CARD_H#define CARD_H class Card {public: Card(); Card(char r, char s); int getValue(); void showCard();…arrow_forward// EmployeeBonus.cpp - This program calculates an employee's yearly bonus. #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables here string employeeFirstName; string employeeLastName; double numTransactions; double numShifts; double dollarValue; double score; double bonus; const double BONUS_1 = 50.00; const double BONUS_2 = 75.00; const double BONUS_3 = 100.00; const double BONUS_4 = 200.00; // This is the work done in the housekeeping() function cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter number of shifts: "; cin >> numShifts; cout << "Enter number of transactions: "; cin >> numTransactions; cout << "Enter dollar value of transactions: "; cin >> dollarValue; // This is…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
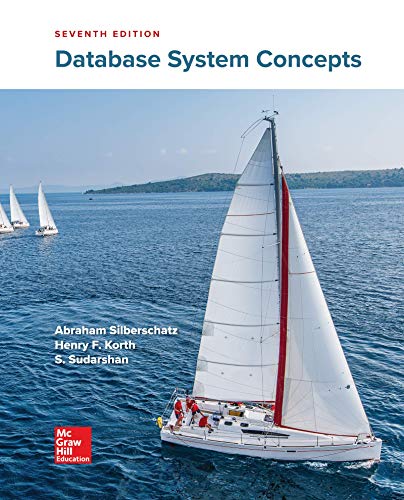
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
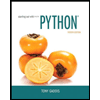
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
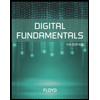
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
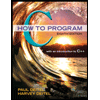
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
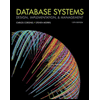
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
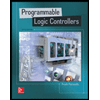
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education