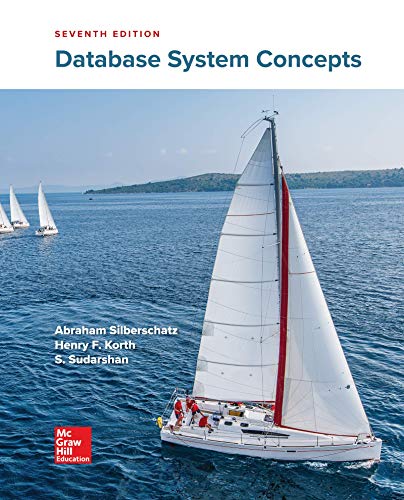
Concept explainers
C++ PLEASE!!
// FILE: simplestring.h
// CLASS PROVIDED: string (a sequence of characters)
//
// CONSTRUCTOR for the string class:
// string(const char str[ ] = "") -- default argument is the empty string.
// Precondition: str is an ordinary null-terminated string.
// Postcondition: The string contains the sequence of chars from str.
//
// CONSTANT MEMBER FUNCTIONS for the string class:
// size_t length( ) const
// Postcondition: The return value is the number of characters in the
// string.
//
// char operator [ ](size_t position) const
// Precondition: position < length( ).
// Postcondition: The value returned is the character at the specified
// position of the string. A string's positions start from 0 at the start
// of the sequence and go up to length( )-1 at the right end.
//
// MODIFICATION MEMBER FUNCTIONS for the string class:
// void operator +=(const string& addend)
// Postcondition: addend has been catenated to the end of the string.
//
// void operator +=(const char addend[ ])
// Precondition: addend is an ordinary null-terminated string.
// Postcondition: addend has been catenated to the end of the string.
//
// void operator +=(char addend)
// Postcondition: The single character addend has been catenated to the
// end of the string.
//
// NON-MEMBER FUNCTIONS for the string class:
// string operator +(const string& s1, const string& s2)
// Postcondition: The string returned is the catenation of s1 and s2.
//
// ostream& operator <<(ostream& outs, const string& source)
// Postcondition: The sequence of characters in source has been written
// to outs. The return value is the ostream outs.
//
// istream& getline(istream& ins, string& target)
// Postcondition: A string has been read from the istream ins. The reading
// operation reads all characters (including white space) until a newline
// or end of file is encountered. The newline character is read and
// discarded (but not added to the end of the string).
//
// VALUE SEMANTICS for the string class:
// Assignments and the copy constructor may be used with string objects.
//
// DYNAMIC MEMORY usage by the string class:
// If there is insufficient dynamic memory then the following functions call
// new_handler: The constructors, operator +=, operator +, and the
// assignment operator.
#ifndef SIMPLESTRING_H
#define SIMPLESTRING_H
#include <cstdlib> // Provides size_t
#include <iostream> // Provides ostream and istream
class string_node
{
// define a node class here.
};
class string
{
public:
// CONSTRUCTORS and DESTRUCTOR
string(const char str[] = "");
string(const string& source);
~string();
// MODIFICATION MEMBER FUNCTIONS
void operator +=(const string& addend);
void operator +=(const char addend[]);
void operator +=(char addend);
string& operator =(const string& source);
int length() const;
char operator [ ](int position) const;
private:
string_node* head_ptr;
string_node* tail_ptr;
int many_nodes;
mutable string_node* cursor;
mutable size_type cursor_index;
}
// NON-MEMBER FUNCTIONS for the string class.
string operator +(const string& s1, const string& s2);
ostream& operator <<( ostream& outs, const string& source);
void getline( istream& ins, string& target);
}
#endif
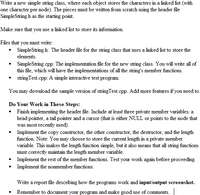

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- C++ This program does not compile! Spot the error and give the line number. Presume that the header file is error free and // Implementation file for the Rectangle class.1. #include "Rectangle.h" // Needed for the Rectangle class2. #include <iostream> // Needed for cout3. #include <cstdlib> // Needed for the exit function4. using namespace std; //***********************************************************// setWidth sets the value of the member variable width. *//*********************************************************** 5. void Rectangle::setWidth(double w){6. if (w >= 0)7. width = w;8. else{9. cout << "Invalid width\n";10. exit(EXIT_FAILURE);}} //***********************************************************// setLength sets the value of the member variable length. *//*********************************************************** 11. void Rectangle::setLength(double len){12. if (len >= 0)13. length = len;14. else{15. cout << "Invalid length\n";16.…arrow_forward#include using namespace std; void scanPrice (string [], double []); // Do not modify the code double printReceipt(string [], double[]); // Do not modify the code int main () { double sum; string item [3] = {"Vegetable","Egg", "Meat"}; double price [3];| %3D scanPrice (item,price); sum = RrintReceipt (item,price); cout Price: 10 The price of Vegetable is RM 2 The price of Egg is RM 3 The price of Meat is RM 10 Your total expenses is RM 15arrow_forwardC++ Coding Help (Operators: new delete) Assign pointer myGas with a new Gas object. Call myGas's Read() to read the object's fields. Then, call myGas's Print() to output the values of the fields. Finally, delete myGas. Ex: If the input is 14 45, then the output is: Gas's volume: 14 Gas's temperature: 45 Gas with volume 14 and temperature 45 is deallocated. #include <iostream>using namespace std; class Gas { public: Gas(); void Read(); void Print(); ~Gas(); private: int volume; int temperature;};Gas::Gas() { volume = 0; temperature = 0;}void Gas::Read() { cin >> volume; cin >> temperature;} void Gas::Print() { cout << "Gas's volume: " << volume << endl; cout << "Gas's temperature: " << temperature << endl;} Gas::~Gas() { // Covered in section on Destructors. cout << "Gas with volume " << volume << " and temperature " << temperature << " is deallocated."…arrow_forward
- initial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forwardC++arrow_forwardC++ Define an "Expression" class that manages expression info: operand1 (integer), operand2 (integer), and the expression operator (char). It must provide at least the following method:- toString() to return a string containing all the expression info such as 50 + 50 = 100 Write a command-driven program named "ListExpressions" that will accept the following commands:add, listall, listbyoperator, listsummary, exit "add" command will read the expression and save it away in the list "listall" command will display all the expressions "listbyoperator" command will read in the operator and display only expressions with that given operator "listsummary" command will display the total number of expressions, the number of expressions for each operator, the largest and smallest expression values "exit" command will exit the program Requirements:- It must be using the array of pointers (not vector) to Expression objects to manage the list of Expression…arrow_forward
- #include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forward/* Interface File: Movie.h Declaration of Movie Class (Variables - "Data Members" or "Attributes" AND Functions - "Member Functions" or "Methods") */ #ifndef MOVIE_H // Include Guard or Header Guard -If already defined ignore rest of code #define MOVIE_H // Otherwise, define MOVIE_H #include<string> // Note: Not "using namespace std;" or even "using std::string" class Movie { private: std::string title = ""; // Explict scope used --> std::string int year = 0; public: Movie(std::string title = "", int year = 1888); // Declaring a Default Constructor ~Movie(); // A Destructor used for freeing up resources void set_title(std::string title_param); std::string get_title() const; // "const" safeguards class variable changes within function std::string get_title_upper() const; void set_year(int year_param); int get_year() const; }; //…arrow_forwardpython programing: You are going to create a race class, and inside that class it has a list of entrants to the race. You are going to create the daily schedule of three or so races and populate the races with the jockey/horse entries and then print out the schedule. Create a race class that has The name of the race as a character string, ie “RACE 1” or “RACE 2”, The time of the race A list of entries for that race Create a list of of 3 (or 4) of those race objects(races) for the day For the list of entries for a particular race, make a entrant class (the building block of your entrants list) you should have The horse name The jockey name Now you need to Populate the structure (ie set up three races on the schedule, add 2-3 horses entries to each race. You can hardcode the entries if you want to, rather than getting them from the user, just to save the time of making a menu interface and whatnot. Be able to print the days schedule So example output would be…arrow_forward
- C++ code. Please describe what EACH line means/does. Ignore code that is commented out.arrow_forward// Fix this program that works// Use welcome function that display the message in diffreent color// Use Draw function to diplay table in gree/yellow// Remove, add, any code that you believe is not useful#include <iostream>#include <windows.h> using namespace std;char mat[3][3];void table(); //function to print the tablevoid welcome(); //function for welcome screenint main(){ int i, j, m, n, sum = 0; char ch; welcome(); system("pause"); for (m = 0; m < 3; m++) for (n = 0; n < 3; n++) mat[m][n] ='W'; table(); while (sum < 10) { //================== for player 1 =================== cout << "Player 1 is X Choose the position : "; cout << "Row:"; cin >> i; cout << "Coloumn:"; cin >> j; //============== if position is wrong ============ for (; i > 3 || i < 1 || j>3 || j < 1 || ('x' == mat[i - 1][j - 1] || 'o'…arrow_forwardC++ Define an "Expression" class that manages expression info: operand1 (integer), operand2 (integer), and the expression operator (char). It must provide at least the following method:- toString() to return a string containing all the expression info such as 50 + 50 = 100 Write a command-driven program named "ListExpressions" that will accept the following commands:add, listall, listbyoperator, listsummary, exit "add" command will read the expression and save it away in the list "listall" command will display all the expressions "listbyoperator" command will read in the operator and display only expressions with that given operator "listsummary" command will display the total number of expressions, the number of expressions for each operator, the largest and smallest expression values "exit" command will exit the program Requirements:- It must be using the array of pointers to Expression objects to manage the list of Expression objects. Please…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
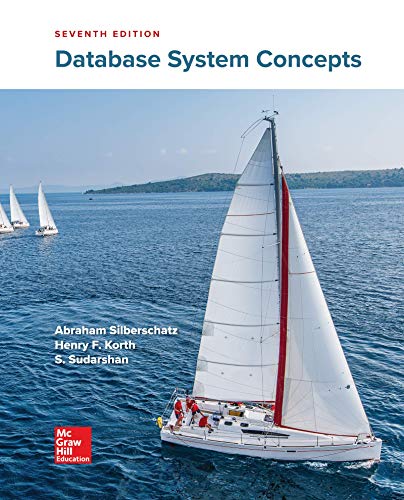
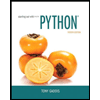
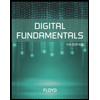
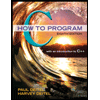
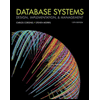
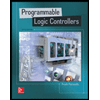