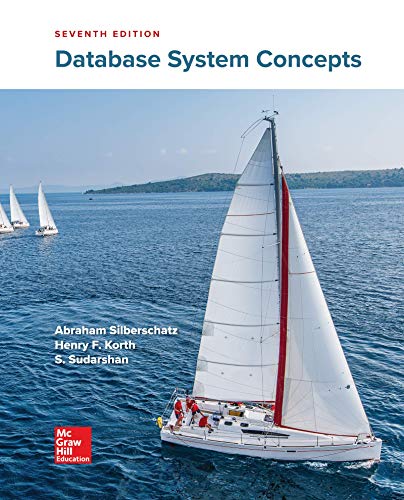
Java program:
1. Write a class Point. Points are described as having an (x,y) location. Instance
variables should be private. Your class should contain a constructor that takes two
integer values to set the point, accessor and mutator methods for the private
instance variables and a toString method that prints the coordinates of the point.
2. Write a class Circle. Since circles are described by a centerpoint and a radius,
the Circle class should extend the Point class. You need to add a private
double for the radius. You should include a constructor, an accessor and a mutator
method for the radius. Negative radii are not permitted (check and print an error
message in the mutator). You should override the toString method to print the
coordinates of the point, the radius, and the area of the circle.
3. Write a class Cylinder. Since cylinders are circles with heights, the Cylinder
class should extend the Circle class. Add the appropriate instance variable and
include a constructor, an accessor and a mutator method. Override the toString
method to print the coordinates of the point, the radius, the height and the volume
of the cylinder.
4. Write a driver class called Application that reads three x,y locations, radii and
heights from the user. Create 3 point, 3 circle and 3 cylinder objects using the
input values. Use polymorphism (and a for loop) to store all objects in one array.
Finally use polymorphism to print the description of each object.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- There is a class hierarchy that includes three classes: class Animal { protected int age; public int getAge() { return age; } public void setAge(int age) { this.age = age; }}class Pet extends Animal { protected String name; public String getName() { return name; } public void setName(String name) { this.name = name; }}class Cat extends Pet { protected String color; public String getColor() { return color; } public void setColor(String color) { this.color = color; }} Given the following object: Pet cat = new Cat(); Select all invalid method invocations.arrow_forward1) Define a Person class containing two private attributes: last name, first name. Provide this class with a constructor allowing the initialization of its attributes and a method that displays the last name and first name of a person: void display (). 2) Define a Customer class inheriting from the Person class and having a private attribute: numidentity. Provide this class with a constructor allowing you to create a customer from their last name, first name and identity card number and a method: • void display() which displays the last name, first name and ID number of a customer. 3) Define an Owner class inheriting from the Person class and having two private attributes: name of the video club (nomclub), address of the club (adrclub). Provide this class with a constructor allowing to create a landlord from his name, his first name, the name of his video club, the address of his club and a method: • void display() who displays the name, first name, last name and address of his video…arrow_forwardAssignment: Write the class XXXX_Worker with constructors, accessors, mutuators, and a toString method. A Worker has a Worker Name and Number. Write the class XXXX_ProductionWorker which is a subclass of Worker. The production worker has a shift number (values: 1 or 2) and an Hourly pay rate. A shift number of 1 means the day shift and 2 means the night shift. Write the class XXXX_ShiftSupervisor which is a subclass of Worker. The shift supervisor is a salaried worker who supervises a shift. The shift supervisor has a yearly bonus field. The yearly bonus is earned at year end based on performance. Write a class, XXXX_TestWorker, which does the following: Creates one Shift Supervisor object from information entered by the user. Creates an Array of Production Workers that can hold 3 objects. Itcreates3ProductionWorkerobjectsfrominformationenteredbytheuser Prints the information about each object in the format shown below using the toString methods of the…arrow_forward
- Complete the partial declaration of Class Car: public class Car { // declare three private instance variables: color, speed, model // define a constructor with two parameters: c , m which will assign values to color and model. //define set and get method for all instance variables //define a method named calspeed( ) with int return type, the method will increase the speed by 50 mile per hour. } Complete the application below. public class CarApp { public static void main() { string color = blue; string model = C2021;i int speed = 80; // declare necessary object matching to the constructor definition // display the color and model on the screen // call the calspeed( ) method and display the new speed after calculation. } }arrow_forward1. Create abstract class Pet , which is the abstract super class of all animals.1. Declare an integer attribute called legs, which records the number of legs for this animal.2. Define a constructor that initializes the legs attribute.3. Declare an abstract method eat.4. Declare a concrete method walk that prints out something about how the animals walks (include the number of legs).2. Create the Dog class that implements Pet class.1. This class must include a String attribute to store the name and food of the pet2. Define a default constructor that calls the super class constructor to specify that all dogs have 4 legs and name.3. override the eat and walk method.3. Create the Cat class that Implements Pet class.1. This class must include a String attribute to store the name of the pet, and a string to save food .2. Define a constructor that takes one String parameter that specifies the cat's name. This constructor must also call the super class constructor to specify that all cats have…arrow_forwardJAVA CODE Write a java set of classes that could be used to do simple calculations on geometricobjects. The set should include the following classes: a) Shapes ,containing abstract methods( a methods that is declared without animplementation) area and perimeter b) Rectangle, a subclass of Shape, containing a constructor (with parameters forlength and width) along with methods area and perimeter. c) Triangle, a subclass of Shape, containing a constructor (with parameters for the three sides of a triangle) along with methods area ( s = (a+b+c)/2, A = sqrt( s(s-a)(s-b)(s-c) ) ) and perimeter d) Square, a subclass of Rectangle, containing a constructor (with a parameter for thelength of a side) and methods that invoke the methods of the Rectangle class todetermine the perimeter and area of a square.arrow_forward
- java program 1.Define a class named Circle 2. A Circle object stores a radius and the (x, y) coordinates of its center point using Point class (It should have at least two private fields to store x and y coordinates of a point, one constructor, a toString method, and a distance method). 3. Each Circle object should have 2 private fields, a Point object, and radius. Each Circle object should have the following public methods: Circle(p, radius) - Constructs a new circle with a center specified by the given Point p and with the given integer radius getCenter() - Returns point object for the center of the circle getRadius() - Returns the circle's radius. getArea() - Returns the area occupied by the circle, using the formula πr^2 getCircumference() - Returns the circle's circumference (distance around the circle), using the formula 2πr. toString() - Returns a string representation of the circle, such as "Circle[center=(75, 20),radius=30]" contains(p) - Returns true if the point p lies…arrow_forward2. Add a constructor for Animal class shown. The constructor should pass in a string parameter named "sound". 1 using System; 2 3 public class Animal 4 { 5 public string Sound { get; set; } public void Speak() { Console.Writeline("The dog says " + Sound); } 10 11 } 12 13 public class Program 14 { public static void Main() { 15 16 17 18 } 19 } A 00arrow_forwardConsider the following class definition. public class Gadget { private static int status = 0; public Gadget () { status - 10; public static void setstatus (int s) { status The following code segment appears in a method in a class other than Gadget. Gadget a = new Gadget () ; Gadget.setstatus(3); Gadget b = new Gadget () ; Which of the following best describes the behavior of the code segment? a. The code segment does not compile because the setstatus method should be called on an object of the class Gadget, not on the class itself. b. The code segment creates two Gadget objects a and b. The class Gadget's static variable status is set to 10, then to 3, and then back to 10. c. The code segment creates two Gadget objects a and b. After executing the code segment, the object a has a status value of 3 and the object b has a status value of 3. d. The code segment creates two Gadget objects a and b. After executing the code segment, the object a has a status value of 3 and the object b has a…arrow_forward
- Java Programming problem Create a checking account class with three attributes: account number, owner’s name, and balance. Create constructor, getters and setters. If one is to set the initial balance of an account as a negative value in a setter or a constructor, remind the user about the error and set it as 0. Create a CheckingAccountDemo class, and create a main method in which Ask for the name, account number, and initial balance for one account from the keyboard input. With these input values as arguments in a call to the constructor, create a CheckingAccount object. Print the summary of this account that includes information on the name, account number, and balance by calling the getters.arrow_forwardDesign a class named Author with the following members: A field for the author’s name (a String) A field for the author’s year of birth (an int) One or more constructors and appropriate accessor and mutator methods A toString method that displays the author’s info as: Author: Mary Shelley (1797) Save the file as Author.java. Next, create an abstract class named Book with the following members: A field for the book title (a String) A field for the book author (a reference to an Author object) A field for the book price (a double) A constructor that requires the title and author Get methods for the title, author, and price An abstract method named setPrice A toString method that displays the book’s title, author’s info, and price as: Frankenstein Author: Mary Shelley (1797) Price: $23.95 Save the file as Book.java. Create Fiction and NonFiction child classes of Book. Each must include a setPrice method that sets the price for all Fiction books to $23.95 and for all NonFiction books…arrow_forwardDesign and implement a class dayType that implements the day of the week in a program. The classdayType should store the day, such as Sun for Sunday. The program should be able to perform thefollowing operations on an object of type dayType:a) Set the day.b) Print the day.c) Return the day.d) Return the next day.e) Return the previous day.f) Calculate and return the day by adding certain days to the current day. For example, if the current day is Monday and we add 4 days, the day to be returned is Friday. Similarly, if today is Tuesday and we add 13 days, the day to be returned is Monday.g) Add the appropriate constructors.The class dateType was designed to implement the date in a program, but the member function setDateand the constructor do not check whether the date is valid before storing the date in the member variables.Rewrite the definitions of the function setDate and the constructor so that the values for the month, day,and year are checked before storing the date into the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
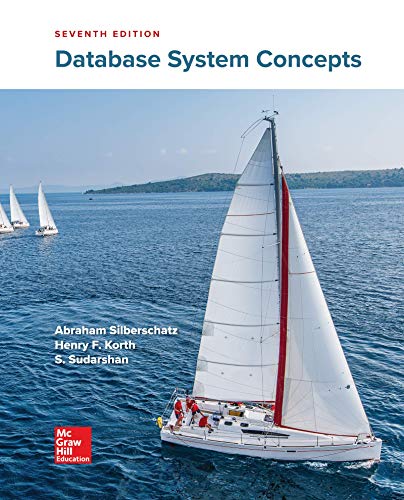
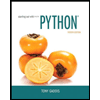
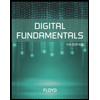
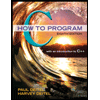
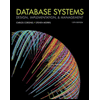
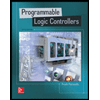