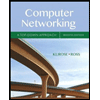
Java Programming
I am using intellij to write my code
1. Implement the shape hierarchy shown above, in which
Shape, TwoDeimentionalShape and
ThreeDimentionalShape should be abstract classes.
2. Include abstract method getArea in the
TwoDimensionalShape class. Also, include abstract
methods getArea and getVolume in the
ThreeDimensionalShape class.
3. Each subclass of TwoDimensionalShape should contain
method getArea to calculate the area of the twodimensional shape.
4. Each subclass of ThreeDimensionalShape should have
getArea and getVolume to calculate the surface area and
volume.
5. Override the toString method in each concrete class (third
level), to include basic information about the object, e.g.,
“Sphere object, radius = 4”
• Create a test program that uses an array of Shape references
to objects of each concrete class in the hierarchy. For
example,
Shape[] shapes = new Shapes [5];
Shape[0] = new Circle(2);
• Create a loop that process all the shapes in the array, print
basic information for each object. Also, determine whether
each shape is TwoDimensionalShape or
ThreeDimensionalShape:
• If it is TwoDimensionalShape, display its area.
• If it is ThreeDimensionalShape, display its area and volume
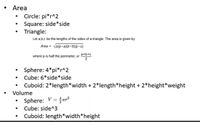
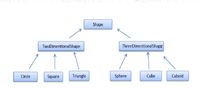

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 10 images

- what are the positives and negatives of the two designs attached. The Java code next to each design shows an example of how the constructors are implemented in the subclasses. Constructor code for the other two other subclasses is same as the Bipedal constructor in each design (except the number of legs value). designs attachedarrow_forwardJava program: The class diagram with four classes Mammal, Human, Student and Doctor is given. The Mammal class is given as well. Write down the remaining classes as described in the class diagram. The fields and methods for each class is given in the attachment. //in Mammel.java Public interface Mammal{ public double hairColor(): } 1. Class Human: Fields: age(int), weight(double), height(double) Methods: getAge, getWeight, getHeight 2. Class Student: Fields: major (String), gpa (double), creditHours (int) methods: getMajor: returns major, getGpa: returns gpa, getYear: returns freshman, sophmore, junior, senior as determined by earned credit hours Freshman : less than 32 credit hours sophmore: at least 32 but less than 64 credit hours junior: at least 64 credit hours but less than 96 credit hours senior: at least 96 credit hours 3.Class Doctor: Fields: years(int), Speciality (String) methods: getYears: returns years, getSpeciality: returns speciality, getSalary: calculates…arrow_forwardNeed it in the java language, doesn't have to be perfect. If you can do the whole program that would be best but if not an outline would suffice so I can get a good idea of how to finish the program. Thanks!arrow_forward
- MUST BE IN JAVA. PLEASE USE COMMENTS AND WRITE THE CODE IN SIMPLEST FORM.arrow_forwardUse java programming properties to write all the necessary classes and/or interfaces for a solution to the problem. Focus on class structure and interaction. You may implement your solution however you wish, but you will be graded on the appropriateness of your solution to the requirements. Note the use of capitalization and parentheses for clarification. You may use whatever constructors or additional methods you wish. Define a structure that can represent Animals. Animals have two behaviors; they can speak() and they can move(). By default, when an animal moves, the text ”This animal moves forward” is displayed. By default, when an animal speaks, the text ”This animal speaks” is displayed. A general Animal should not be able to be instantiated. Define two classes, Goose and Lynx, that are Animals. Both Goose and Lynx behave such that where “Animals” is displayed in speak() or move(), “goose” or “lynx” is displayed by the appropriate classes. Finally, any instance of Goose can fly(),…arrow_forwardYou need to perform following things to demonstrate the concept of abstract class. Step 1: Create the abstract class that has following methods. • Calculate the area of circle(Abstract method) • Calculate the area of triangle(Abstract method) • Calculate the area of rectangle(Method with implementation) Step 2: Create another class that extends above class and provide implementation of abstract methods. Step 3: Create the main method inside above class and display output of area of circle, area of triangle and area of rectangle. You need to submit the following things: • Java program with both the classes An output screenshot created using Microsoft Wordarrow_forward
- Create a short program in Java which contains the below information: - Has one abstract and two regular classes - Has two interfaces (the two regular classes have to implement at least one interface - At least one regular class must implement both interfaces - The two classes have to extend the abstract class - The abstract class must have a method - At least one interface must have a method - At least two fields in each class, this includes the superclass - All fields are instantiated within the constructorarrow_forwardWrite the definition of classes and interfaces in the given diagram using following details: a) Interface shape has a method draw(). b) Override the method draw() in all subclasses. c) Define a non-abstract method fillColor() in Circle class. (I want the complete solution using the codes in bluej program also diagram) bluej java coodarrow_forwardProvide answers fastly and correctlyarrow_forward
- First, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information, and all of these should be defined as Private: A first name (given name) A last name (family name/surname) Student number (ID) – an integer number (of type long) The Student class will have at least the following constructors and methods: (i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables of Student. (ii) only necessary set and get methods for a valid class design. (iii) a reportGrade method, which you have nothing to report here, you can just print to the screen a message “There is no grade here.”. This method will be overridden in the respective child classes. (iv) an equals method which compares two student objects and returns true if they have the same student number (ID), otherwise it returns false. You may add other…arrow_forwardIn the class diagram below we have a money class for an object-oriented parking system that is to be designed using java. Briefly explain any implementation decisions and the reasoning behind those without writing the complete code. N.B explain how the implementation will proceed instead of writing codearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
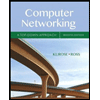
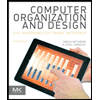
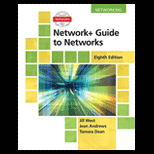
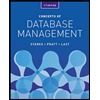
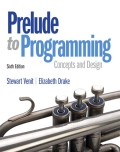
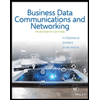