Deeper Class Design - the Square, Circle, Picture Class he Bigger Picture – The ObjectList (or ArrayList (v1.0)) Class A picture is simply a composition of shapes, and in this last section, we’ll build a class used to manage such a picture. We’ll create this new class by reusing code from an existing piece of software. We’ll create an Picture class that will contain, amongst other state items (data), an array (or list) of Objects that are the Squares and Circles in the Picture to be drawn. Picture.java will be a simple abstraction here, and will just “draw” shapes to the console in the order that they appear in the list –ignoring the coordinate pairs stored in each Shape for now. Again, note the static storage restriction of only 100 shapes per picture; in future sections, we’ll learn how to dynamically resize our arrays. Aside: when we get to working with any of the Java graphics framework classes and/or swing, then a simple use of this Picture class (called a PicturePanel extending JPanel) would accommodate for much more interesting shape behaviors and interactions. Copy-and-paste the IntList.java code into a new file called Picture.java Note this is the same process we used to quickly create the Circle.java class Change the class name from IntList to Picture. Change the instance variable that is your int[] array to an Object[] array called myShapes. Change your “public void add(int nx)” method to “public void add(Object nx)”. Remove functions sum(), indexOf(), and save() and recompile using the provided ShapesPictureDriver.java driver. Run your main and notice it still processes a list of integers correctly Now run the driver code and uncomment the Picture segment and execute it. Data Members private Object[] myShapes; //used to be “data[]” This is an array of type Object, which can thus store any object of any class What about primitives? private int numElements; This integer tracks the number of live shapes in our array Method Members void add ( Object shape ); This function adds the Circle or Square to the array of circles @Override public String toString(); { //iterates through the array, calling toString() on each shape and appending this //to one large string to be returned Sample Output [] [] O O O Alternate Sample Output [] [] O O O Square class public class Square { privateintx; privateinty; privatedoublesideLength; private String shape = "[]"; public Square() { } public Square (int nx, int ny) { x = nx; y = ny; } public Square (int nx, int ny, double len) { x = nx; y = ny; sideLength = len; } publicvoid draw() { System.out.println(shape); } publicint getX() { returnx; } publicvoid setX(int nX) { x = nX; } publicint getY() { returny; } publicvoid setY(int nY) { y = nY; } publicdouble getSideLength() { returnsideLength; } publicvoid setSideLength(double sl) { sideLength = sl; } publicdouble getArea() { returnsideLength * sideLength; } public String toString() { returnshape; } publicboolean equals(Square that) { if (this.x == that.x & this.y == that.y && this.sideLength == that.sideLength) { return true; } return false; } }
In java language
Deeper Class Design - the Square, Circle, Picture Class
he Bigger Picture – The ObjectList (or ArrayList (v1.0)) Class
A picture is simply a composition of shapes, and in this last section, we’ll build a class used to manage such a picture. We’ll create this new class by reusing code from an existing piece of software. We’ll create an Picture class that will contain, amongst other state items (data), an array (or list) of Objects that are the Squares and Circles in the Picture to be drawn. Picture.java will be a simple abstraction here, and will just “draw” shapes to the console in the order that they appear in the list –ignoring the coordinate pairs stored in each Shape for now. Again, note the static storage restriction of only 100 shapes per picture; in future sections, we’ll learn how to dynamically resize our arrays. Aside: when we get to working with any of the Java graphics framework classes and/or swing, then a simple use of this Picture class (called a PicturePanel extending JPanel) would accommodate for much more interesting shape behaviors and interactions.
- Copy-and-paste the IntList.java code into a new file called Picture.java
- Note this is the same process we used to quickly create the Circle.java class
- Change the class name from IntList to Picture.
- Change the instance variable that is your int[] array to an Object[] array called myShapes.
- Change your “public void add(int nx)” method to “public void add(Object nx)”.
- Remove functions sum(), indexOf(), and save() and recompile using the provided ShapesPictureDriver.java driver.
- Run your main and notice it still processes a list of integers correctly
- Now run the driver code and uncomment the Picture segment and execute it.
Data Members
- private Object[] myShapes; //used to be “data[]”
- This is an array of type Object, which can thus store any object of any class
- What about primitives?
- This is an array of type Object, which can thus store any object of any class
- private int numElements;
- This integer tracks the number of live shapes in our array
Method Members
- void add ( Object shape );
- This function adds the Circle or Square to the array of circles
- @Override
public String toString(); {
//iterates through the array, calling toString() on each shape and appending this
//to one large string to be returned
Sample Output
[] [] O O O
Alternate Sample Output
[]
[]
O
O
O
Square class
public class Square {
privateintx;
privateinty;
privatedoublesideLength;
private String shape = "[]";
public Square() {
}
public Square (int nx, int ny) {
x = nx;
y = ny;
}
public Square (int nx, int ny, double len) {
x = nx;
y = ny;
sideLength = len;
}
publicvoid draw() {
System.out.println(shape);
}
publicint getX() {
returnx;
}
publicvoid setX(int nX) {
x = nX;
}
publicint getY() {
returny;
}
publicvoid setY(int nY) {
y = nY;
}
publicdouble getSideLength() {
returnsideLength;
}
publicvoid setSideLength(double sl) {
sideLength = sl;
}
publicdouble getArea() {
returnsideLength * sideLength;
}
public String toString() {
returnshape;
}
publicboolean equals(Square that) {
if (this.x == that.x & this.y == that.y && this.sideLength == that.sideLength) {
return true;
}
return false;
}
}
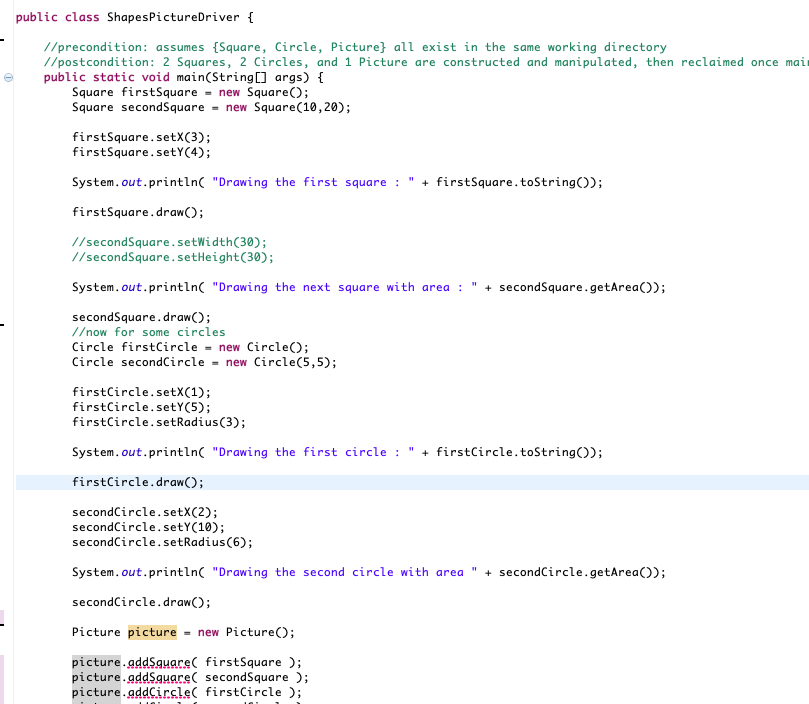
![public class Picture { //or Intlist, caps at 100 elements with no bullet-proofing or bounds checks, etc.
private int numElements
private Object0 myShapes;
0; //This integer tracks the number of live shapes in our array
public void addCObject nx) {
//add the value n to array data
myShapes [numElements] = nx;
//add one to num_elements
numElements++;
//In a loop, append all the elements in our data array to this string, separated by a comma
public String toString() {
String retVal - "";
for(int i = 0; i < numElements; i++) {
if (i==0) {
retVal += myShapes[i];
else {
retVal = retVal + ",'
}
}
return retVal;
+ myShapes [i];](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa426d8d0-2bb0-421c-8768-59095f46a7fa%2F5a4e71d7-73b2-4231-8431-37a4cd22cccc%2Fduzj8te_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

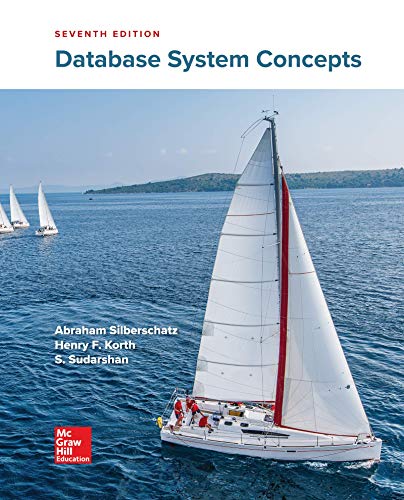
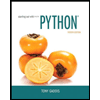
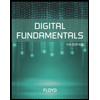
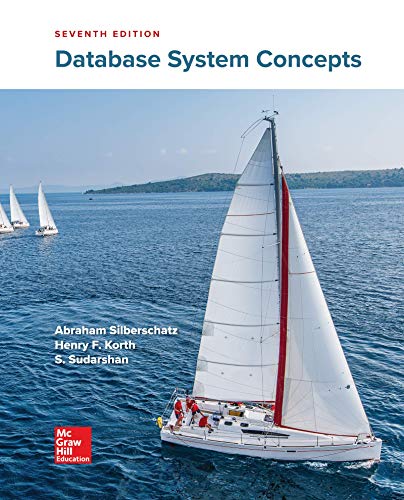
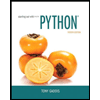
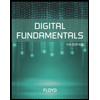
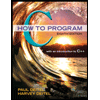
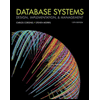
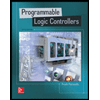