Java programming lanaguage A. Design and implement the class Circle that contains the double private data field radius and the following member methods: getRadius that returns the radius of Circle setRadius that sets the radius of Circle area that calculates and return the area of a Circle object (area = π*r²). toString that returns the radius of a Circle object as a string. Two constructors; the first one without arguments. The second constructor with one parameter r. It initializes the radius to r. B.Design and implement the class Cylinder that inherits the class Circle and contains one double private data field height and the following member methods: getHeight that returns the height of Cylinder setHeight that sets the height of Cylinder area that calculates and return the area of a Cylinder object (area = 2*π*r²+2*π*r*h). volume that calculates and return the volume of a Cylinder object (volume = π*r²*h). toString that returns the radius and height of a Cylinder object as string. Two constructors; the first one without arguments. The second constructor with two parameters r and h. It initializes the radius to r and the height to h. C. Using sentinel controlled loop, write Test_Driver program to read radius and height of 20 Cylinder objects and store them in an array. Your program must be able to output, for Cylinder object, its radius, height, area and
Java programming lanaguage A. Design and implement the class Circle that contains the double private data field radius and the following member methods: getRadius that returns the radius of Circle setRadius that sets the radius of Circle area that calculates and return the area of a Circle object (area = π*r²). toString that returns the radius of a Circle object as a string. Two constructors; the first one without arguments. The second constructor with one parameter r. It initializes the radius to r. B.Design and implement the class Cylinder that inherits the class Circle and contains one double private data field height and the following member methods: getHeight that returns the height of Cylinder setHeight that sets the height of Cylinder area that calculates and return the area of a Cylinder object (area = 2*π*r²+2*π*r*h). volume that calculates and return the volume of a Cylinder object (volume = π*r²*h). toString that returns the radius and height of a Cylinder object as string. Two constructors; the first one without arguments. The second constructor with two parameters r and h. It initializes the radius to r and the height to h. C. Using sentinel controlled loop, write Test_Driver program to read radius and height of 20 Cylinder objects and store them in an array. Your program must be able to output, for Cylinder object, its radius, height, area and
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Java programming lanaguage
- A. Design and implement the class Circle that contains the double private data field radius and the following member methods:
- getRadius that returns the radius of Circle
- setRadius that sets the radius of Circle
- area that calculates and return the area of a Circle object (area = π*r²).
- toString that returns the radius of a Circle object as a string.
- Two constructors; the first one without arguments. The second constructor with one parameter r. It initializes the radius to r.
- B.Design and implement the class Cylinder that inherits the class Circle and contains one double private data field height and the following member methods:
- getHeight that returns the height of Cylinder
- setHeight that sets the height of Cylinder
- area that calculates and return the area of a Cylinder object
(area = 2*π*r²+2*π*r*h).
- volume that calculates and return the volume of a Cylinder object
(volume = π*r²*h).
- toString that returns the radius and height of a Cylinder object as string.
- Two constructors; the first one without arguments. The second constructor with two parameters r and h. It initializes the radius to r and the height to h.
- C. Using sentinel controlled loop, write Test_Driver program to read radius and height of 20 Cylinder objects and store them in an array. Your program must be able to output, for Cylinder object, its radius, height, area and
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
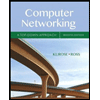
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
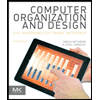
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
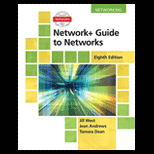
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
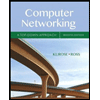
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
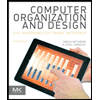
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
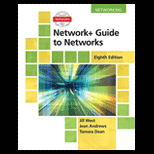
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
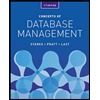
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
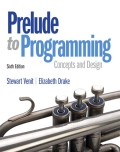
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
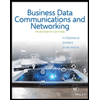
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY