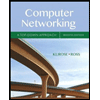
Java
Design and implement a GUI program to convert a positive number given in one base to another base. For this problem, assume that both bases are less than or equal to 10. Consider the sample data:
number = 2010, base = 3, and new base = 4. In this case, first convert 2010 in base 3 into the equivalent number in base 10 as follows:
2 * 3^3 + 0 * 3^2 + 1 * 3 + 0 = 54 + 0 + 3 + 0 = 57
To convert 57 to base 4, you need to finde the remainders obtained by dividing by 4, as shown in the following;
57 % 4 = 1, quotient = 14
14 % 4 2, quotient = 3
3 % 4 =3, quotient = 0. Therefore, 57 in base 4 is 321.
Here is my code:
String inputnumber;
int base;
//int outputbase;
int newbase;
System.out.println("Enter the base number to convert from: ");
base = console.nextInt();
System.out.println("The base number you want to convert from is: " + base);
System.out.println("Enter the number: ");
inputnumber = console.next();
System.out.println("The number you entered is: " + inputnumber);
System.out.println("Enter the new base to convert to: ");
newbase = console.nextInt();
System.out.println("The new base you want to convert to is: " + newbase);
// System.out.println("The number " + inputnumber + " in base " + base
// + "is" + + " in" + newbase);
if (base < 0 || base >= 11) {
System.out.println("You entered an invalid base. The base should be between 1 and 10");
}
int x;
x = inputnumber.length();
int number = Integer.parseInt(inputnumber);
int sum = 0;
for (int i = 0; i < x; i++)
{ sum = sum + number%10 * (int)Math.pow(base, i);
number = (number - number%10)/10;}
System.out.println(sum);
String reverse = " "; String output = " ";
int i ;
int y ;
do {
y = sum % newbase;
reverse += String.valueOf(y);
sum = (sum / newbase) ;
// i++;
// System.out.print(y);
}
while (sum > 0 );
for (i = reverse.length() - 1; i > 0; i--)
{
output += reverse.charAt(i);
}System.out.println("The number " + inputnumber + " in base " + base
+ " is " + output + " in base " + newbase) ;
System.out.println();

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Say we are about to build an ArrayList. Your ArrayList should guarantee that the array capacity is at most four times the number of elements. What would you like to do to maintain such a limit on the capacity? What is the benefit of using iterators? Can you describe your first experience of GUIs? And could you describe what is the advantage of using GUIs over Command-Line Interface (CLI) operations?arrow_forwardComputer Science Create a UML diagram of the following methods & classes (java language) using LucidApp. Class Name Method Description Calculation Multiply Multiplies two variables with data type double and returns the product Returns the area of the Rectangle using the method "Multiply" of the "Calculation" class in the calculation Rectangle Area Rec_Prism Volume - Returns the volume of a Rectangular Prism - Inherits class "Rectangle" and utilizes the method "Area" in the calculation Triangle Returns the area of the Triangle using the method “Multiply" of the "Calculation" class in the calculation Area Tri_Prism Volume Returns the volume of a Triangular Prism - Inherits class "Triangle" and utilizes the method "Area" in the calculation Reflexive Power - Inherits class "Calculation" - Returns the product of a number multiplied by itself based on the exponent - Requires two input parameters: a = the number b= the exponent - Calls the method "Multiply" to perform the multiply operation.…arrow_forwardPlease answer this question. Yes or no does a tic tac toe game made on a computer using java have a searching and sorting code. Explain why, or why not. Do not make a tic tac toe game using java. Just answer the question above.arrow_forward
- Write a program in JAVA to input the number of elements in an array, also input the values in the array from the console. Replace all those elements which are even and greater than 5, with -99. Print the final array. Constraints: The array should initially not contain -99. The value of the number of elements should not be greater than 50.arrow_forwardA teacher has three exam grades for all the members of her class, and she worries that the grades are too low. You need to complete the method, named testAverage, in the class named Grades.java. There are two parameters to this method: the first is an integer representing the number of students in the class and, the second is a two-dimensional array of integers. In other words, each row represents the three grades for one student, while the columns represent the three sets of test grades. The return value should be the number of students with an average exam grade below seventy. The grades should be treated as double variables. For example, consider the grades for the four students in the following 2-D array, [ [88, 84, 89] [76, 64, 67] [85, 76, 79] [95, 90, 91] ] The first student has an exam average of 87.0 (= (88+84+89)/3 = 261/3), the second student has an exam average of 69.0 (= (77+64+67)/3 = 207/3), the third student has an exam average of 80.0 (= (85+76+79)/3 = 240/3), while…arrow_forwardWrite a GUI program on Java to play dice games between two players. The game starts with a coin toss determining who starts. Players roll the dice in turn to get the combination of the two dice. In each round the player with the lowest combination loses a “life” – each player starts with six lives. When the count of lives goes down to 0, the player is out of the game. If the player is not satisfied with the result of rolling, he/she may re-roll. If he/she wishes re-roll, the result of the second is the final.arrow_forward
- This is needed in Java Given that an ArrayList of Strings named nameList has already been created and names have already been inserted, write the statement necessary to delete the name at index 15.arrow_forwardWrite a Java program (Hangman.java) to play the classic word game Hangman. In the game, a word is randomly selected from an array of possible words. Then, the user is prompted to guess letters in the word one at a time. When the user makes a correct guess, all instances of that letter in the word are shown. When all letters in the word have been guessed, the game ends and the score (number of missed guesses) is displayed. A user can play the game multiple times if they choose. Here is a sample run of a correct program (user input indicated by orange text):Enter a letter in word ******** > cEnter a letter in word c******* > rEnter a letter in word c******r > s s is not in the wordEnter a letter in word c******r > tEnter a letter in word c****t*r > mEnter a letter in word c*m**t*r > t t is already in the wordEnter a letter in word c*m**t*r > pEnter a letter in word c*mp*t*r > oEnter a letter in word comp*t*r > eEnter a letter in word comp*ter >…arrow_forwardTHIS IS MEANT TO BE IN JAVA. What we've learned so far is variables, loops, and we just started learning some array today. The assignment is to get an integer from input, and output that integer squared, ending with newline. But i've been given some instructions that are kind of confusing to me. Please explain why and please show what the end result is. Here are some extra things i've been told to do with the small amount of code i've already written... Type 2 in the input box, then run the program so I can note that the answer is 4 Type 3 in the input box instead, run, and note the output is 6. Change the output statement to output a newline: System.out.println(userNumSquared);. Type 2 in the input box Change the program to use * rather than +, and try running with input 2 (output is 4) and 3 (output is now 9, not 6 as before). This is what I have so far... import java.util.Scanner; public class NumSquared {public static void main(String[] args) {Scanner scnr = new…arrow_forward
- You are working as a software developer on NASA's Mars Explorer robotic rover project. You need to implement some code to compute the surface area of the 3D, tube-shaped part shown below: a cylinder of radius r and height h (in units centimeters) with a hollowed-out center also in the shape of a cylinder with radius n and height h (also in cm). Both the outside and the inside of the tube will need to be coated in a thin layer of gold, which is why we need to know its surface area. Write code that will compute and print the total surface area of the shape. n esc r Examples: Inputs r = 10, n = 8.5, h = 22.5 r = 9.25, n = 6.8, h r = 4.15, n = 3.9, h = 11.7 = 12.16 X h Output Value 2789.7342763877364 1473.347264273195 604.4267185874082 2 3 80 F3 000 DO F4 ✓0 F5 完成时间:18:42 PNEUE MacBook Air F6 F7 DII F8 tv 8 F9 F10 Farrow_forwardPlease help me build this quick project in Java.arrow_forwardYou have an integer matrix representing a plot of land, where the value at that location represents the height above sea level. A value of zero indicates water. A pond is a region of water connected vertically, horizontally, or diagonally. The size of the pond is the total number of connected water cells. Write a method to compute the sizes of all ponds in the matrix.EXAMPLEInput:0 2 1 00 1 0 11 1 0 10 1 0 1Output: 2, 4, 1 (in any order)arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
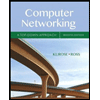
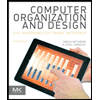
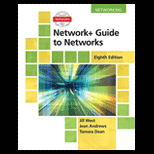
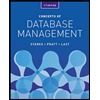
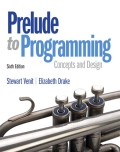
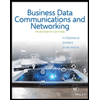