Java Programming: There must be no error in the code. Attached is rubric with output it must show. Show the full code and the screenshot of the output in the terminal of Java. Create a Parser class that must have a constructor that accepts your collection of Tokens. We will be treating the collection of tokens as a queue – taking off the front. It isn’t necessary to use a Java Queue, but you may. We will add three helper functions to parser. These should be private: matchAndRemove – accepts a token type. Looks at the next token in the collection: If the passed in token type matches the next token’s type, remove that token and return it. If the passed in token type DOES NOT match the next token’s type (or there are no more tokens) return null. expectEndsOfLine – uses matchAndRemove to match and discard one or more ENDOFLINE tokens. Throw a SyntaxErrorException if no ENDOFLINE was found. peek – accepts an integer and looks ahead that many tokens and returns that token. Returns null if there aren’t enough tokens to fulfill the request. Parser – parse Create a public parse method. There are no parameters, and it returns Node. This will be called from main once lexing is complete. For now, we are going to only parse a subset of Shank V2 – mathematical expressions. Parse should call expression() then expectEndOfLine() in a loop until either returns null. Don’t worry about storing the return node but you should print it out (using ToString()) for testing. The tricky part of mathematical expressions is order of operations. Fortunately, this is fairly easily solved in recursive descent by using a few functions. The intuition is this: accept tokens from the highest priority first. When you can’t find any that fit the pattern, fall back to lower priority. The thing that makes this “weird” is that we will call the lowest level first. But it calls the next highest first thing. Let’s take a look: EXPRESSION = TERM { (plus or minus) TERM} TERM = FACTOR { (times or divide or mod) FACTOR} FACTOR = {-} number or lparen EXPRESSION rparen Notice the curly braces – these indicate 0 or more. expression(), term() and factor() will all be methods of our recursive descent parser. All of them must use matchOrRemove(). The formulae above are very literal. Consider the first one: EXPRESSION = TERM { (plus or minus) TERM} expression() calls term(). Then it (in a loop) looks for a + or -. If it finds one, it calls term again and constructs a MathOpNode using the two term() returns as the left and right. That MathOpNode becomes the left if there are more +/- sign. So 1+2+3 becomes: MathOpNode(+,IntegerNode(1),(MathOpNode(+,IntegerNode(2),IntegerNode(3))) Let’s look at a complete example: 3*-4+5: our tokens will look something like this: NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE– your token types might differ a little We will call expression(). The first thing it does is look for a term by calling term(). term() calls factor() first thing. factor() will matchAndRemove MINUS and not find it. It then tries NUMBER succeeds. NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE It looks at the NUMBER node, sees no decimal point and makes an IntegerNode (3) and returns it. term() gets the IntegerNode from factor() and now should loop looking for TIMES or DIVIDE. It finds a TIMES. NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE It should then call factor(). factor() looks for the MINUS. It finds it. It internally notes that we are now in “negative” mode. It then finds the NUMBER. It sees no decimal point and makes an IntegerNode (-4) and returns it. NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE term() gets the IntegerNode. It constructs a MathOpNode of type multiply with a left and right side of the two IntegerNodes. It now looks for multiply and divide and doesn’t find them. It returns the MathOpNode that it has. Now expression() has a MathOpNode. It looks for plus or minus and finds plus. NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE Now expression() calls term() which calls factor() which looks for NUMBER, finds it and constructs an IntegerNode (5). NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE term() won’t find a times or divide, so it returns the IntegerNode. expression() now has a + with a left and a right side, so it creates that MathOpNode. It looks for plus/minus, doesn’t find it and returns the + MathOpNode. parse() should print the returned Node (the MathOpNode).
Java Programming: There must be no error in the code. Attached is rubric with output it must show. Show the full code and the screenshot of the output in the terminal of Java.
Create a Parser class that must have a constructor that accepts your collection of Tokens. We will be treating the collection of tokens as a queue – taking off the front. It isn’t necessary to use a Java Queue, but you may.
We will add three helper functions to parser. These should be private:
matchAndRemove – accepts a token type. Looks at the next token in the collection:
If the passed in token type matches the next token’s type, remove that token and return it.
If the passed in token type DOES NOT match the next token’s type (or there are no more tokens) return null.
expectEndsOfLine – uses matchAndRemove to match and discard one or more ENDOFLINE tokens. Throw a SyntaxErrorException if no ENDOFLINE was found.
peek – accepts an integer and looks ahead that many tokens and returns that token. Returns null if there aren’t enough tokens to fulfill the request.
Parser – parse
Create a public parse method. There are no parameters, and it returns Node. This will be called from main once lexing is complete. For now, we are going to only parse a subset of Shank V2 – mathematical expressions. Parse should call expression() then expectEndOfLine() in a loop until either returns null. Don’t worry about storing the return node but you should print it out (using ToString()) for testing.
The tricky part of mathematical expressions is order of operations. Fortunately, this is fairly easily solved in recursive descent by using a few functions. The intuition is this: accept tokens from the highest priority first. When you can’t find any that fit the pattern, fall back to lower priority. The thing that makes this “weird” is that we will call the lowest level first. But it calls the next highest first thing. Let’s take a look:
EXPRESSION = TERM { (plus or minus) TERM}
TERM = FACTOR { (times or divide or mod) FACTOR}
FACTOR = {-} number or lparen EXPRESSION rparen
Notice the curly braces – these indicate 0 or more.
expression(), term() and factor() will all be methods of our recursive descent parser. All of them must use matchOrRemove(). The formulae above are very literal. Consider the first one:
EXPRESSION = TERM { (plus or minus) TERM}
expression() calls term(). Then it (in a loop) looks for a + or -. If it finds one, it calls term again and constructs a MathOpNode using the two term() returns as the left and right. That MathOpNode becomes the left if there are more +/- sign. So 1+2+3 becomes:
MathOpNode(+,IntegerNode(1),(MathOpNode(+,IntegerNode(2),IntegerNode(3)))
Let’s look at a complete example: 3*-4+5: our tokens will look something like this:
NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE– your token types might differ a little
We will call expression(). The first thing it does is look for a term by calling term(). term() calls factor() first thing. factor() will matchAndRemove MINUS and not find it. It then tries NUMBER succeeds.
NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE
It looks at the NUMBER node, sees no decimal point and makes an IntegerNode (3) and returns it. term() gets the IntegerNode from factor() and now should loop looking for TIMES or DIVIDE. It finds a TIMES.
NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE
It should then call factor(). factor() looks for the MINUS. It finds it. It internally notes that we are now in “negative” mode. It then finds the NUMBER. It sees no decimal point and makes an IntegerNode (-4) and returns it.
NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE
term() gets the IntegerNode. It constructs a MathOpNode of type multiply with a left and right side of the two IntegerNodes. It now looks for multiply and divide and doesn’t find them. It returns the MathOpNode that it has.
Now expression() has a MathOpNode. It looks for plus or minus and finds plus.
NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE
Now expression() calls term() which calls factor() which looks for NUMBER, finds it and constructs an IntegerNode (5).
NUMBER TIMES MINUS NUMBER PLUS NUMBER ENDOFLINE
term() won’t find a times or divide, so it returns the IntegerNode. expression() now has a + with a left and a right side, so it creates that MathOpNode. It looks for plus/minus, doesn’t find it and returns the + MathOpNode. parse() should print the returned Node (the MathOpNode).
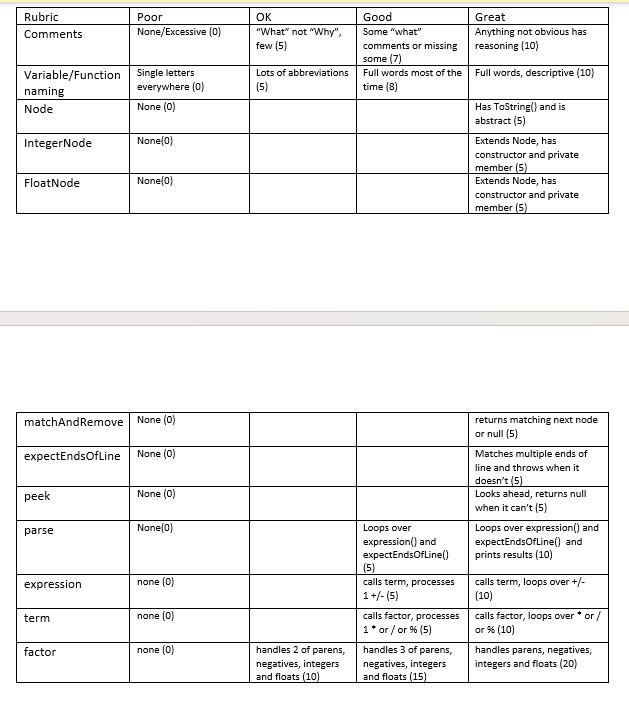
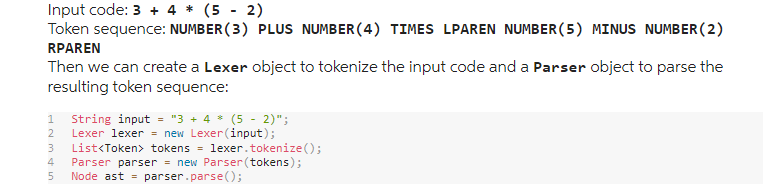

Step by step
Solved in 3 steps

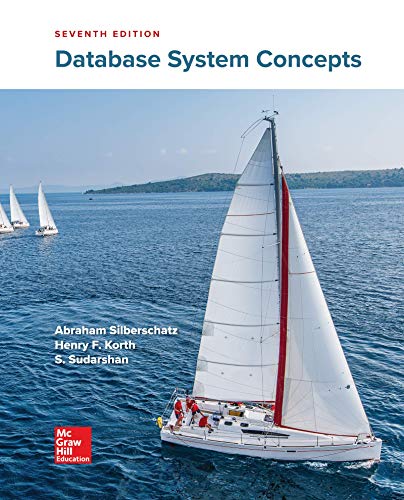
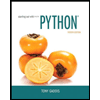
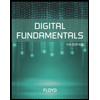
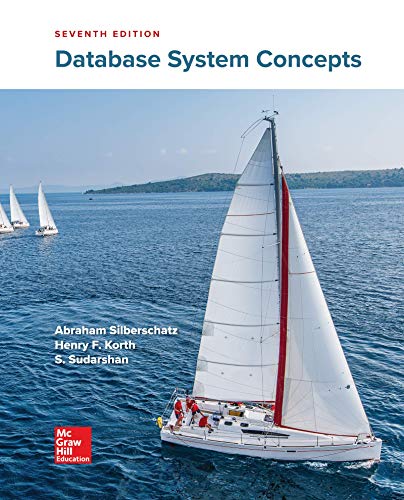
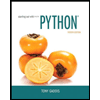
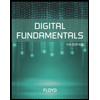
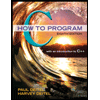
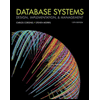
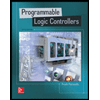